class类编写图书管理
时间: 2023-06-23 18:08:31 浏览: 99
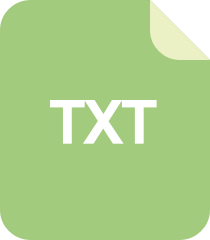
c++课程设计图书管理
好的,下面是一个简单的图书管理系统的类:
```python
class Book:
def __init__(self, title, author, ISBN, num_copies):
self.title = title
self.author = author
self.ISBN = ISBN
self.num_copies = num_copies
def display(self):
print("Title:", self.title)
print("Author:", self.author)
print("ISBN:", self.ISBN)
print("Number of copies:", self.num_copies)
def check_availability(self):
if self.num_copies > 0:
return True
else:
return False
def loan(self):
if self.check_availability():
self.num_copies -= 1
print("Loan successful.")
else:
print("Book not available for loan.")
def return_book(self):
self.num_copies += 1
print("Book returned.")
```
这个类包括以下方法:
- `__init__()`:初始化图书的标题、作者、ISBN号和副本数量。
- `display()`:打印图书的详细信息。
- `check_availability()`:检查图书是否可供借阅。
- `loan()`:借出一本图书,并更新库存数量。
- `return_book()`:归还一本图书,并更新库存数量。
使用这个类,你可以创建一个图书对象并执行相应的操作:
```python
book1 = Book("The Great Gatsby", "F. Scott Fitzgerald", "978-3-16-148410-0", 5)
book1.display() # Title: The Great Gatsby
# Author: F. Scott Fitzgerald
# ISBN: 978-3-16-148410-0
# Number of copies: 5
book1.loan() # Loan successful.
book1.display() # Title: The Great Gatsby
# Author: F. Scott Fitzgerald
# ISBN: 978-3-16-148410-0
# Number of copies: 4
book1.return_book() # Book returned.
book1.display() # Title: The Great Gatsby
# Author: F. Scott Fitzgerald
# ISBN: 978-3-16-148410-0
# Number of copies: 5
```
阅读全文
相关推荐
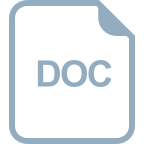














