c++编写定义判断闰年的函数
时间: 2024-02-17 09:04:56 浏览: 64
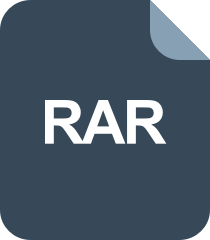
c++判断闰年
下面是一个使用 C++ 编写的判断闰年的函数:
```c++
#include <iostream>
bool isLeapYear(int year)
{
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
return true;
}
return false;
}
return true;
}
return false;
}
int main()
{
int year;
std::cout << "请输入要判断的年份:" << std::endl;
std::cin >> year;
if (isLeapYear(year)) {
std::cout << year << " 年是闰年" << std::endl;
} else {
std::cout << year << " 年不是闰年" << std::endl;
}
return 0;
}
```
在函数中,我们首先对年份进行了 4、100 和 400 的判断,如果符合这些条件,则该年是闰年,否则不是闰年。在主函数中,我们通过输入年份来调用该函数,并根据返回值输出判断结果。
阅读全文
相关推荐
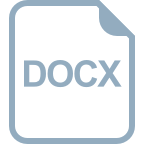
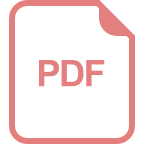















