arduino 去掉字符串数组中的字符
时间: 2023-10-10 20:11:33 浏览: 235
要从字符串数组中去掉特定的字符,可以使用C++中的`std::remove`函数。这个函数将要删除的字符移动到数组的末尾,并返回一个指向新的末尾位置的迭代器。然后,可以使用`std::string`的`resize`方法将数组的大小缩小到新的末尾位置。
以下是一个示例代码,从字符串数组中删除所有的字母'a':
```c++
#include <algorithm>
#include <string>
int main() {
char arr[] = "hello world";
std::string str(arr);
auto new_end = std::remove(str.begin(), str.end(), 'a');
str.resize(std::distance(str.begin(), new_end));
// 输出结果
for (char c : str) {
Serial.print(c);
}
}
```
以上代码将输出字符串"hello world"中所有'a'字母被删除后的结果。
相关问题
arduino将字符串通过空格分割为数组
以下是一个示例代码,可以将一个字符串通过空格分割为一个字符串数组:
```
String inputString = "Hello World Arduino";
String tokens[3]; // 定义一个大小为3的字符串数组
int i = 0;
while (inputString.length() > 0) {
int spaceIndex = inputString.indexOf(' '); // 查找空格的位置
if (spaceIndex == -1) { // 如果没有找到空格
tokens[i] = inputString; // 将剩余的字符串作为最后一个元素存入数组
break;
}
tokens[i] = inputString.substring(0, spaceIndex); // 截取空格之前的字符串存入数组
inputString = inputString.substring(spaceIndex + 1); // 更新输入字符串,去掉已经存入数组的部分
i++;
}
// 输出数组中的每个元素
for (int j = 0; j < 3; j++) {
Serial.println(tokens[j]);
}
```
在这个示例中,输入的字符串是"Hello World Arduino",我们定义了一个大小为3的字符串数组tokens。在while循环中,我们不断查找输入字符串中的空格,并将空格之前的字符串存入数组中。最后,我们输出数组中的每个元素。
这个示例代码还可以进一步改进,比如可以动态地分配字符串数组的大小,以适应不同长度的输入字符串。
arduino将串口输入的字符串转化为数组并通过另一个串口使用modbus协议传出
以下是一个示例代码,可以将串口输入的字符串转化为数组,并通过另一个串口使用modbus协议传出:
```cpp
#include <ModbusMaster.h>
// 定义串口输入缓冲区和缓冲区长度
const int BUFFER_SIZE = 50;
char buffer[BUFFER_SIZE];
int bufferIndex = 0;
// 定义modbus从机地址和寄存器地址
const int SLAVE_ADDRESS = 1;
const int REGISTER_ADDRESS = 0;
// 定义modbus主机
ModbusMaster node;
void setup() {
// 初始化串口和modbus主机
Serial.begin(9600);
node.begin(SLAVE_ADDRESS, Serial);
}
void loop() {
// 读取串口输入,并将字符串转化为数组
while (Serial.available() > 0) {
char c = Serial.read();
if (bufferIndex < BUFFER_SIZE - 1) {
buffer[bufferIndex] = c;
bufferIndex++;
}
}
// 如果收到了完整的字符串,则将数组转化为modbus数据,并发送出去
if (bufferIndex > 0 && buffer[bufferIndex - 1] == '\n') {
buffer[bufferIndex - 1] = '\0'; // 去掉换行符
int dataLength = strlen(buffer) + 2; // 数据长度为字符串长度加上CRC校验码长度
uint8_t data[dataLength];
for (int i = 0; i < strlen(buffer); i++) {
data[i] = buffer[i];
}
uint16_t crc = node.calculateCRC(data, strlen(buffer));
data[dataLength - 2] = crc & 0xFF;
data[dataLength - 1] = crc >> 8;
node.writeMultipleRegisters(REGISTER_ADDRESS, dataLength / 2, (uint16_t*)data);
bufferIndex = 0;
}
// 处理modbus响应
node.poll();
}
```
在上述代码中,我们使用了ModbusMaster库来实现modbus协议的主机功能。在setup函数中,我们初始化了串口和modbus主机。在loop函数中,我们读取串口输入,并将字符串转化为数组。如果收到了完整的字符串,则将数组转化为modbus数据,并发送出去。同时,我们也调用了node.poll()函数,处理modbus响应。
需要注意的是,在将字符串转化为modbus数据时,我们使用了CRC校验码来保证数据的正确性。在modbus协议中,每个数据包都要附带一个CRC校验码,用于检测数据传输过程中是否出现了错误。在本例中,我们使用了ModbusMaster库中的calculateCRC函数来计算CRC校验码。
另外,需要根据具体情况修改从机地址和寄存器地址。在本例中,我们将数据写入了寄存器地址为0的位置。
阅读全文
相关推荐
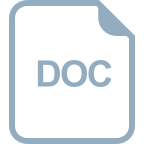
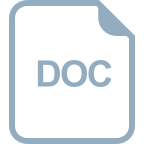
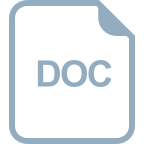


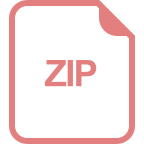
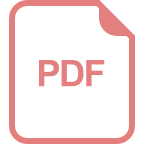


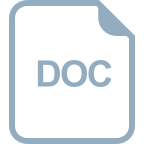
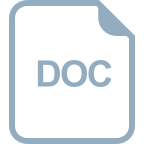
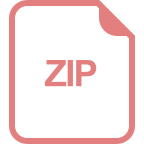
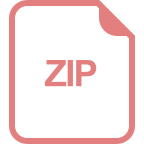
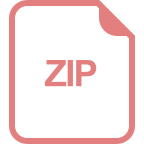