在只知道大华平台地址、用户名、密码、ClientId、ClientSecret情况下下载大华视频监控示例
时间: 2023-12-04 22:01:46 浏览: 65
在只知道大华平台地址、用户名、密码、ClientId、ClientSecret的情况下,可以通过调用大华平台提供的接口获取视频地址,再通过上面提供的Java代码将视频下载到本地。
以下是一个示例代码,可以作为参考:
```java
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.util.Base64;
public class DownloadVideo {
public static void main(String[] args) {
String apiUrl = "http://xxx.xxx.xxx.xxx";
String username = "your_username";
String password = "your_password";
String clientId = "your_client_id";
String clientSecret = "your_client_secret";
String cameraIndexCode = "your_camera_index_code"; // 摄像头编号
String savePath = "D:/video.mp4"; // 保存路径
try {
// 获取AccessToken
String accessToken = getAccessToken(apiUrl, clientId, clientSecret, username, password);
// 获取视频地址
String videoUrl = getVideoUrl(apiUrl, accessToken, cameraIndexCode);
// 下载视频
URL url = new URL(videoUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setConnectTimeout(5 * 1000);
conn.setRequestMethod("GET");
conn.setRequestProperty("Charset", "UTF-8");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Authorization", "Bearer " + accessToken);
InputStream inputStream = conn.getInputStream();
byte[] getData = readInputStream(inputStream);
FileOutputStream fos = new FileOutputStream(savePath);
fos.write(getData);
if (fos != null) {
fos.close();
}
if (inputStream != null) {
inputStream.close();
}
System.out.println("视频下载完成");
} catch (Exception e) {
e.printStackTrace();
}
}
public static String getAccessToken(String apiUrl, String clientId, String clientSecret, String username, String password) throws Exception {
String authUrl = apiUrl + "/artemis/api/auth/token?appKey=" + clientId + "&appSecret=" + clientSecret;
URL url = new URL(authUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setConnectTimeout(5 * 1000);
conn.setRequestMethod("POST");
conn.setRequestProperty("Charset", "UTF-8");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setDoOutput(true);
conn.setDoInput(true);
String authString = username + ":" + password;
String authStringEnc = Base64.getEncoder().encodeToString(authString.getBytes("utf-8"));
conn.setRequestProperty("Authorization", "Basic " + authStringEnc);
String params = "{\"grantType\":\"password\",\"username\":\"" + username + "\",\"password\":\"" + password + "\"}";
byte[] postData = params.getBytes("utf-8");
conn.getOutputStream().write(postData);
InputStream inputStream = conn.getInputStream();
byte[] getData = readInputStream(inputStream);
String result = new String(getData, "utf-8");
String accessToken = "";
if (result.contains("accessToken")) {
String[] arr = result.split(",");
for (String str : arr) {
if (str.contains("accessToken")) {
accessToken = str.split(":")[1].replaceAll("\"", "");
break;
}
}
}
return accessToken;
}
public static String getVideoUrl(String apiUrl, String accessToken, String cameraIndexCode) throws Exception {
String url = apiUrl + "/artemis/api/video/v1/cameras/" + URLEncoder.encode(cameraIndexCode, "utf-8") + "/previewURLs";
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setConnectTimeout(5 * 1000);
conn.setRequestMethod("GET");
conn.setRequestProperty("Charset", "UTF-8");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Authorization", "Bearer " + accessToken);
InputStream inputStream = conn.getInputStream();
byte[] getData = readInputStream(inputStream);
String result = new String(getData, "utf-8");
String videoUrl = "";
if (result.contains("url")) {
String[] arr = result.split(",");
for (String str : arr) {
if (str.contains("url")) {
videoUrl = str.split(":")[1].replaceAll("\"", "");
break;
}
}
}
return videoUrl;
}
public static byte[] readInputStream(InputStream inputStream) throws Exception {
byte[] buffer = new byte[1024];
int len;
ByteArrayOutputStream bos = new ByteArrayOutputStream();
while ((len = inputStream.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
bos.close();
return bos.toByteArray();
}
}
```
上面的代码中,我们首先调用`getAccessToken()`方法获取AccessToken,然后调用`getVideoUrl()`方法获取视频地址,最后通过调用`downloadVideo()`方法将视频下载到本地。需要注意的是,视频地址返回的是一个JSON字符串,需要对字符串进行解析获取实际可用的视频地址。另外,需要将摄像头编号替换成实际可用的编号。
相关推荐
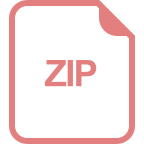
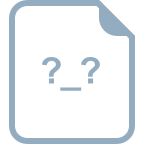
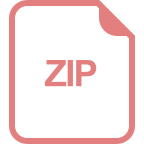














