输出一个整数,表示这个数组中第二大的元素 c
时间: 2024-12-15 18:14:40 浏览: 2
为了找到一个数组中的第二大的元素,你可以遍历整个数组并维护两个变量:`max1` 和 `max2`。初始时,假设 `max1` 为数组的第一个元素,而 `max2` 为负无穷大。然后,对于数组中的每个元素,如果它大于当前的 `max1`,就将 `max1` 更新为该元素;如果它小于 `max1` 但大于 `max2`,则更新 `max2`。遍历结束后,`max2` 就会存储数组中的第二大的值。
这是一个简单的算法示例:
```python
def find_second_largest(arr):
if len(arr) < 2:
return None # 如果数组长度小于2,没有第二大的元素
max1 = max2 = float('-inf')
for num in arr:
if num > max1:
max2 = max1
max1 = num
elif num > max2 and num != max1:
max2 = num
return max2 if max2 != float('-inf') else None # 返回第二大的元素,如果没有则返回None
# 示例数组
arr = [5, 2, 8, 4, 9]
second_largest = find_second_largest(arr)
print("第二大的元素是:", second_largest)
```
如果你需要在 C++ 中实现类似的功能,可以使用类似的思路,但是语法稍有不同:
```cpp
#include <algorithm>
#include <vector>
int findSecondLargest(std::vector<int>& nums) {
if (nums.size() < 2) {
return -1; // 或者抛出异常,取决于你的需求
}
int max1 = nums[0], max2 = INT_MIN;
for (int num : nums) {
if (num > max1) {
max2 = max1;
max1 = num;
} else if (num > max2 && num != max1) {
max2 = num;
}
}
return max2;
}
// 示例数组
std::vector<int> arr = {5, 2, 8, 4, 9};
int second_largest = findSecondLargest(arr);
cout << "第二大的元素是: " << second_largest << endl;
```
阅读全文
相关推荐
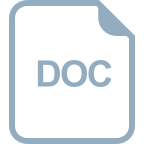
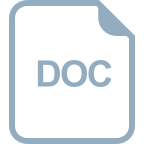
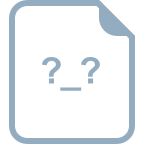















