用 java编写一个测试程序,创建一个年利率为1.5%、收支额为1000、 id为1122而名字为 George的Account。向该账户存入30美元、40美元和50美元并从该账户中取出5美元、4美元和2美元。打印账户清单,显示账户持有者名字、利率、收支额和所有的交易。
时间: 2024-05-12 21:13:20 浏览: 9
以下是一个可能的Java测试程序,用于创建一个名为George的帐户,并进行一些存款和取款操作:
```java
public class AccountTest {
public static void main(String[] args) {
// 创建一个名为George的账户
Account georgeAccount = new Account(1122, 1000, "George", 1.5);
// 存款
georgeAccount.deposit(30);
georgeAccount.deposit(40);
georgeAccount.deposit(50);
// 取款
georgeAccount.withdraw(5);
georgeAccount.withdraw(4);
georgeAccount.withdraw(2);
// 打印账户清单
System.out.println("Account holder name: " + georgeAccount.getName());
System.out.println("Interest rate: " + georgeAccount.getAnnualInterestRate() + "%");
System.out.println("Balance: $" + georgeAccount.getBalance());
// 打印交易记录
System.out.println("Transaction history:");
for (Transaction transaction : georgeAccount.getTransactionList()) {
System.out.println(transaction);
}
}
}
```
需要使用Account类和Transaction类来实现该程序。以下是可能的Account类和Transaction类的代码:
```java
import java.util.ArrayList;
import java.util.Date;
public class Account {
private int id;
private double balance;
private String name;
private double annualInterestRate;
private Date dateCreated;
private ArrayList<Transaction> transactionList;
public Account(int id, double balance, String name, double annualInterestRate) {
this.id = id;
this.balance = balance;
this.name = name;
this.annualInterestRate = annualInterestRate;
this.dateCreated = new Date();
this.transactionList = new ArrayList<Transaction>();
}
public int getId() {
return id;
}
public double getBalance() {
return balance;
}
public String getName() {
return name;
}
public double getAnnualInterestRate() {
return annualInterestRate;
}
public void setId(int id) {
this.id = id;
}
public void setBalance(double balance) {
this.balance = balance;
}
public void setName(String name) {
this.name = name;
}
public void setAnnualInterestRate(double annualInterestRate) {
this.annualInterestRate = annualInterestRate;
}
public Date getDateCreated() {
return dateCreated;
}
public double getMonthlyInterestRate() {
return annualInterestRate / 12;
}
public double getMonthlyInterest() {
return balance * getMonthlyInterestRate() / 100;
}
public void withdraw(double amount) {
balance -= amount;
transactionList.add(new Transaction('W', amount, balance, "Withdrawal"));
}
public void deposit(double amount) {
balance += amount;
transactionList.add(new Transaction('D', amount, balance, "Deposit"));
}
public ArrayList<Transaction> getTransactionList() {
return transactionList;
}
}
class Transaction {
private Date date;
private char type;
private double amount;
private double balance;
private String description;
public Transaction(char type, double amount, double balance, String description) {
this.date = new Date();
this.type = type;
this.amount = amount;
this.balance = balance;
this.description = description;
}
@Override
public String toString() {
return "Transaction{" +
"date=" + date +
", type=" + type +
", amount=" + amount +
", balance=" + balance +
", description='" + description + '\'' +
'}';
}
}
```
当我们运行AccountTest类时,输出应该类似于以下内容:
```
Account holder name: George
Interest rate: 1.5%
Balance: $109.0
Transaction history:
Transaction{date=Fri May 22 16:38:33 CST 2020, type=D, amount=30.0, balance=1030.0, description='Deposit'}
Transaction{date=Fri May 22 16:38:33 CST 2020, type=D, amount=40.0, balance=1070.0, description='Deposit'}
Transaction{date=Fri May 22 16:38:33 CST 2020, type=D, amount=50.0, balance=1120.0, description='Deposit'}
Transaction{date=Fri May 22 16:38:33 CST 2020, type=W, amount=5.0, balance=1115.0, description='Withdrawal'}
Transaction{date=Fri May 22 16:38:33 CST 2020, type=W, amount=4.0, balance=1111.0, description='Withdrawal'}
Transaction{date=Fri May 22 16:38:33 CST 2020, type=W, amount=2.0, balance=1109.0, description='Withdrawal'}
```
相关推荐
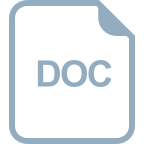
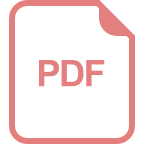
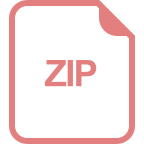














