document.querySelector('#start-btn');
时间: 2024-04-17 09:20:31 浏览: 161
document.querySelector('#start-btn') 是一个 JavaScript 方法,用于通过选择器选择文档中的元素。它接受一个选择器作为参数,并返回匹配该选择器的第一个元素。在这个例子中,选择器是 '#start-btn',表示选择 id 为 'start-btn' 的元素。
该方法常用于获取页面中的特定元素,以便进行后续的操作或事件绑定。如果页面中存在多个匹配的元素,该方法只会返回第一个匹配的元素。
相关问题
优化代码 并使该代码能够实现监测ntp客户端连接服务器的状态 且将其绘制成表格 <html> <head> <meta charset="utf-8"> <title>NTP</title> <script src="jquery-3.4.1.min.js"></script> <style type> .btn { background-color: #4CAF50; border: none; color: white; padding: 10px 15px; text-align: center; text-decoration: none; display: inline-block; font-size: 10px; margin: 4px 2px; transition-duration: 0.4s; cursor: pointer; } .btn:hover { background-color: white; color: #4CAF50; border: 2px solid #4CAF50; } </style> </head> <body> <buttion class = "btn" onclick="startNTP()">启动NTP</buttion> <script> function startNTP(){ var xhr = new XMLHttpRequest(); xhr.open('GET','ntp.php',true); xhr.onload = function(){ alert(xhr.responseText); }; xhr.send(); } </script> </body> </html>
下面是优化后的代码,可以实现监测ntp客户端连接服务器的状态,并将其绘制成表格:
<html>
<head>
<meta charset="utf-8">
<title>NTP</title>
<script src="jquery-3.4.1.min.js"></script>
<style type="text/css">
table {
font-family: Arial, sans-serif;
border-collapse: collapse;
width: 100%;
}
td, th {
border: 1px solid #ddd;
text-align: left;
padding: 8px;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
.btn {
background-color: #4CAF50;
border: none;
color: white;
padding: 10px 15px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 10px;
margin: 4px 2px;
transition-duration: 0.4s;
cursor: pointer;
}
.btn:hover {
background-color: white;
color: #4CAF50;
border: 2px solid #4CAF50;
}
</style>
</head>
<body>
<button class="btn" onclick="startNTP()">启动NTP</button>
<table id="ntpTable">
<thead>
<tr>
<th>服务器地址</th>
<th>状态</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<script>
function startNTP(){
var xhr = new XMLHttpRequest();
xhr.open('GET','ntp.php',true);
xhr.onload = function(){
updateTable(xhr.responseText);
};
xhr.send();
}
function updateTable(response) {
var ntpData = JSON.parse(response);
var tableBody = document.querySelector('#ntpTable tbody');
tableBody.innerHTML = '';
ntpData.forEach(function(ntp) {
var row = document.createElement('tr');
var serverCell = document.createElement('td');
serverCell.textContent = ntp.server;
row.appendChild(serverCell);
var statusCell = document.createElement('td');
statusCell.textContent = ntp.status;
row.appendChild(statusCell);
tableBody.appendChild(row);
});
}
</script>
</body>
</html>
需要确保 `ntp.php` 返回的是一个JSON格式的数据,例如:
```
[
{"server": "ntp1.example.com", "status": "正常"},
{"server": "ntp2.example.com", "status": "异常"}
]
```
<h1>通讯录</h1> <input type="text" id="search-input" placeholder="搜索姓名或电话"> <button id="search-btn">搜索</button> <button class="add-btn">添加联系人</button> <table> <thead> <tr> <th>姓名</th> <th>电话</th> <th>邮箱</th> <th>操作</th> </tr> </thead> <tbody id="contact-list"> <!-- 数据渲染 --> </tbody> </table> <!-- 分页按钮 --> <div id="page-buttons"></div> <!-- 弹出层,用于添加或编辑联系人 --> <div id="modal" class="modal" style="display: none;"> <div class="modal-content"> <span class="close">×</span> <form> <div class="form-group"> <label for="name">姓名</label> <input type="text" id="name" name="name" required> </div> <div class="form-group"> <label for="phone">电话</label> <input type="text" id="phone" name="phone" required> </div> <div class="form-group"> <label for="email">邮箱</label> <input type="text" id="email" name="email" required> </div> <button id="save-btn">保存</button> <button id="cancel-btn">取消</button> </form> </div> </div>帮我添加如下功能:点击搜索按钮或者通过搜索框的内容可以查找到查找的内容,让数据具有添加删除和编辑的功能,编辑的时候增加弹窗;还能对数据进行分页展示,每页最多展示5条数据
好的,我可以帮你添加这些功能。首先,我们需要编写一些 JavaScript 代码来实现这些功能。
1. 搜索功能
我们可以在搜索按钮上添加点击事件,当用户点击搜索按钮时,我们可以获取搜索框中的内容,并根据这个内容来筛选联系人列表中的数据。下面是代码示例:
```javascript
const searchBtn = document.querySelector('#search-btn');
const searchInput = document.querySelector('#search-input');
const contactList = document.querySelector('#contact-list');
searchBtn.addEventListener('click', () => {
const searchText = searchInput.value.toLowerCase();
const contacts = Array.from(contactList.children);
contacts.forEach(contact => {
const name = contact.querySelector('.name').textContent.toLowerCase();
const phone = contact.querySelector('.phone').textContent.toLowerCase();
if (name.includes(searchText) || phone.includes(searchText)) {
contact.style.display = 'table-row';
} else {
contact.style.display = 'none';
}
});
});
```
2. 添加、删除和编辑联系人
我们可以在添加联系人按钮上添加点击事件,当用户点击添加联系人按钮时,弹出添加联系人的弹窗。在弹窗中,我们可以添加保存和取消按钮。当用户点击保存按钮时,我们可以获取输入框中的内容,并将这些内容添加到联系人列表中。当用户点击取消按钮时,我们可以关闭弹窗。
对于删除联系人和编辑联系人,我们可以在每个联系人的操作列中添加删除和编辑按钮。当用户点击删除按钮时,我们可以将该联系人从联系人列表中删除。当用户点击编辑按钮时,我们可以弹出编辑联系人的弹窗,并将该联系人的信息填充到弹窗中。
下面是代码示例:
```javascript
const addBtn = document.querySelector('.add-btn');
const modal = document.querySelector('#modal');
const closeBtn = document.querySelector('.close');
const saveBtn = document.querySelector('#save-btn');
const cancelBtn = document.querySelector('#cancel-btn');
const tbody = document.querySelector('#contact-list');
let contacts = []; // 存储联系人数据
// 添加联系人弹窗
addBtn.addEventListener('click', () => {
modal.style.display = 'block';
});
closeBtn.addEventListener('click', () => {
modal.style.display = 'none';
});
cancelBtn.addEventListener('click', () => {
modal.style.display = 'none';
});
// 保存联系人
saveBtn.addEventListener('click', event => {
event.preventDefault();
const name = document.querySelector('#name').value;
const phone = document.querySelector('#phone').value;
const email = document.querySelector('#email').value;
const contact = { name, phone, email };
contacts.push(contact);
renderContacts(contacts);
modal.style.display = 'none';
});
// 删除联系人
tbody.addEventListener('click', event => {
if (event.target.classList.contains('delete-btn')) {
const contact = event.target.closest('.contact');
const index = contacts.findIndex(c => c.name === contact.querySelector('.name').textContent);
contacts.splice(index, 1);
renderContacts(contacts);
}
});
// 编辑联系人弹窗
tbody.addEventListener('click', event => {
if (event.target.classList.contains('edit-btn')) {
const contact = event.target.closest('.contact');
const name = contact.querySelector('.name').textContent;
const phone = contact.querySelector('.phone').textContent;
const email = contact.querySelector('.email').textContent;
document.querySelector('#name').value = name;
document.querySelector('#phone').value = phone;
document.querySelector('#email').value = email;
modal.style.display = 'block';
saveBtn.removeEventListener('click', saveContact);
saveBtn.addEventListener('click', editContact);
function editContact(event) {
event.preventDefault();
const newName = document.querySelector('#name').value;
const newPhone = document.querySelector('#phone').value;
const newEmail = document.querySelector('#email').value;
const index = contacts.findIndex(c => c.name === name);
contacts[index] = { name: newName, phone: newPhone, email: newEmail };
renderContacts(contacts);
modal.style.display = 'none';
}
}
});
// 渲染联系人列表
function renderContacts(contacts) {
tbody.innerHTML = '';
contacts.forEach(contact => {
const tr = document.createElement('tr');
tr.classList.add('contact');
tr.innerHTML = `
<td class="name">${contact.name}</td>
<td class="phone">${contact.phone}</td>
<td class="email">${contact.email}</td>
<td class="actions">
<button class="edit-btn">编辑</button>
<button class="delete-btn">删除</button>
</td>
`;
tbody.appendChild(tr);
});
renderPageButtons(contacts);
}
// 分页展示联系人列表
function renderPageButtons(contacts) {
const pageButtons = document.querySelector('#page-buttons');
pageButtons.innerHTML = '';
const numPages = Math.ceil(contacts.length / 5);
for (let i = 1; i <= numPages; i++) {
const button = document.createElement('button');
button.textContent = i;
button.addEventListener('click', () => {
const start = (i - 1) * 5;
const end = start + 5;
renderContacts(contacts.slice(start, end));
});
pageButtons.appendChild(button);
}
}
// 初始化
renderContacts(contacts);
```
以上代码中,我们使用了一个数组 `contacts` 来存储联系人信息。在添加联系人弹窗中,当用户点击保存按钮时,我们将输入框中的内容添加到该数组中,并调用 `renderContacts` 函数来重新渲染联系人列表。在删除联系人和编辑联系人时,我们从该数组中删除或编辑相应的联系人,并重新渲染联系人列表。
我们还添加了一个 `renderPageButtons` 函数来渲染分页按钮。该函数根据联系人数组的长度来计算出需要展示的页数,并根据需要展示的页数来创建相应的分页按钮。当用户点击分页按钮时,我们根据该页对应的索引来截取联系人数组,并重新渲染联系人列表。
阅读全文
相关推荐

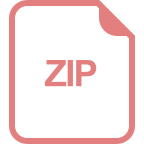










