打地鼠的小游戏HTML+CSS+JS
时间: 2023-09-08 15:08:18 浏览: 137
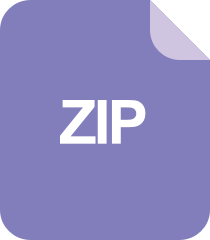
html实现打地鼠小游戏(源码)

好的,下面是一个简单的打地鼠小游戏的 HTML、CSS 和 JavaScript 代码:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>打地鼠小游戏</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<h1>打地鼠</h1>
<div id="game">
<div id="holes">
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
</div>
<div id="scoreboard">
<p>得分:<span id="score">0</span></p>
<p>时间:<span id="time">30</span> 秒</p>
<button id="start-btn">开始游戏</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS 代码:
```css
body {
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
font-size: 3em;
margin-bottom: 0.5em;
}
#game {
margin: 2em auto;
}
#holes {
display: flex;
flex-wrap: wrap;
justify-content: center;
}
.hole {
width: 100px;
height: 100px;
background-image: url('mole.png');
background-size: cover;
margin: 10px;
cursor: pointer;
}
#scoreboard {
margin-top: 2em;
}
#scoreboard p {
margin: 0.5em 0;
}
#score {
font-weight: bold;
}
#time {
font-weight: bold;
color: red;
}
#start-btn {
margin-top: 1em;
font-size: 1.2em;
padding: 0.5em 1em;
background-color: green;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
#start-btn:hover {
background-color: darkgreen;
}
```
JavaScript 代码:
```javascript
const holes = document.querySelectorAll('.hole');
const scoreBoard = document.querySelector('#score');
const timeBoard = document.querySelector('#time');
const startBtn = document.querySelector('#start-btn');
let lastHole;
let timeUp = false;
let score = 0;
let timeLeft = 30;
function randomTime(min, max) {
return Math.floor(Math.random() * (max - min + 1) + min);
}
function randomHole(holes) {
const idx = Math.floor(Math.random() * holes.length);
const hole = holes[idx];
if (hole === lastHole) {
return randomHole(holes);
}
lastHole = hole;
return hole;
}
function peep() {
const time = randomTime(200, 1000);
const hole = randomHole(holes);
hole.classList.add('up');
setTimeout(() => {
hole.classList.remove('up');
if (!timeUp) {
peep();
}
}, time);
}
function startGame() {
score = 0;
timeLeft = 30;
scoreBoard.textContent = score;
timeBoard.textContent = timeLeft;
timeUp = false;
peep();
setTimeout(() => {
timeUp = true;
}, timeLeft * 1000);
}
function bonk(e) {
if (!e.isTrusted) return;
score++;
this.classList.remove('up');
scoreBoard.textContent = score;
}
holes.forEach(hole => hole.addEventListener('click', bonk));
startBtn.addEventListener('click', startGame);
```
这个小游戏的逻辑是每隔一段时间,在九个洞中随机出现一个地鼠,玩家需要在限定时间内尽可能地击打出现的地鼠,最终获得的得分会显示在页面上。
阅读全文
相关推荐

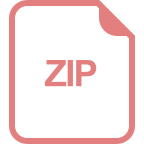
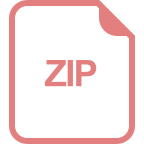
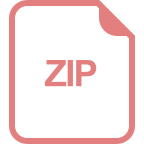
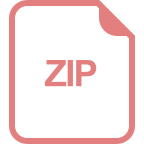
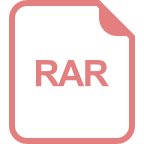
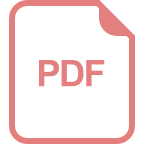
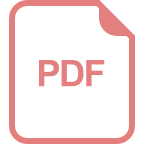
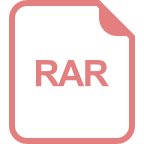
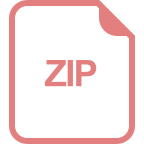
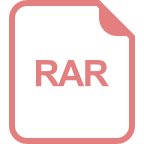



