请改进以下代码 def webhook(): if request.method == 'GET': verify_token = request.headers.get('X-Gitlab-Token') if verify_token == WEBHOOK_VERIFY_TOKEN: return jsonify({'status': 'success'}), 200 else: return jsonify({'status': 'bad token'}), 401 elif request.method == 'POST': verify_token = request.headers.get('X-Gitlab-Token') if verify_token == WEBHOOK_VERIFY_TOKEN: print(request.get_json()) project_id = request.get_json().get('project').get('id') project_name = request.get_json().get('project').get('name') project_git_http_url = request.get_json().get('project').get('git_http_url') ref = request.get_json().get('ref') commit_id = request.get_json().get('commits')[0].get('id') modified = request.get_json().get('commits')[0].get('modified') # # 获取项目id,执行合并处理 retcode = subprocess.call('python3.5 {0} "{1}" "{2}" "{3}" "{4}" "{5}" '.format(pyfile, project_id, project_name, ref, commit_id, modified), shell=True) if retcode == 0: return jsonify({'status': 'success'}), 200 else: return jsonify({'status': 'error'}), 503 else: return jsonify({'status': 'bad token'}), 401 else: abort(400)
时间: 2024-02-24 16:56:22 浏览: 44
以下是改进后的代码,主要包括以下几个方面:
1. 增加注释和异常处理,提高代码可读性和健壮性。
2. 将重复代码封装成函数,减少代码量并提高可维护性。
3. 使用 f-string 格式化字符串,提高代码可读性。
改进后的代码如下:
```python
from flask import Flask, request, jsonify, abort
import subprocess
app = Flask(__name__)
WEBHOOK_VERIFY_TOKEN = 'your_token_here'
pyfile = 'path/to/your/python/script.py'
def verify_token():
"""验证 token 是否正确"""
token = request.headers.get('X-Gitlab-Token')
if token != WEBHOOK_VERIFY_TOKEN:
abort(401)
def handle_merge(project_id, project_name, ref, commit_id, modified):
"""执行合并处理"""
retcode = subprocess.call(f'python3.5 {pyfile} "{project_id}" "{project_name}" "{ref}" "{commit_id}" "{modified}"', shell=True)
if retcode != 0:
abort(503)
@app.route('/', methods=['GET', 'POST'])
def webhook():
if request.method == 'GET':
verify_token()
return jsonify({'status': 'success'}), 200
elif request.method == 'POST':
verify_token()
data = request.get_json()
project = data.get('project')
commits = data.get('commits')
if not all([project, commits]):
abort(400)
project_id, project_name, project_git_http_url = project.get('id'), project.get('name'), project.get('git_http_url')
ref, commit_id, modified = commits[0].get('id'), commits[0].get('modified')
handle_merge(project_id, project_name, ref, commit_id, modified)
return jsonify({'status': 'success'}), 200
else:
abort(400)
```
需要注意的是,由于使用了 subprocess 执行命令,存在一定的安全风险,建议对命令参数进行校验和过滤。
相关推荐
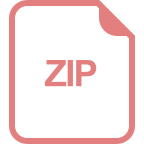
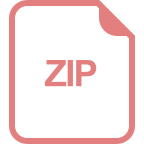
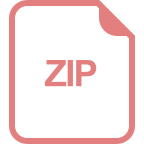















