使用链表实现多项式求值
时间: 2023-05-14 09:05:00 浏览: 142
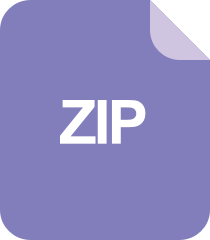
用链表实现多项式_C++_

可以使用链表来实现多项式求值。具体实现方法是,将多项式的每一项作为链表的一个节点,节点中包含该项的系数和指数。然后遍历链表,将每一项的系数乘以对应的指数次幂的值,再将所有结果相加即可得到多项式的值。以下是示例代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int coef; // 系数
int exp; // 指数
struct Node *next;
} Node;
// 创建一个新节点
Node *createNode(int coef, int exp) {
Node *node = (Node *)malloc(sizeof(Node));
node->coef = coef;
node->exp = exp;
node->next = NULL;
return node;
}
// 向链表中插入一个节点
void insertNode(Node **head, Node *node) {
if (*head == NULL) {
*head = node;
} else {
Node *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
}
// 遍历链表,求多项式的值
int evaluate(Node *head, int x) {
int sum = 0;
Node *p = head;
while (p != NULL) {
sum += p->coef * pow(x, p->exp);
p = p->next;
}
return sum;
}
int main() {
Node *head = NULL;
insertNode(&head, createNode(2, 3));
insertNode(&head, createNode(3, 2));
insertNode(&head, createNode(4, 1));
insertNode(&head, createNode(5, 0));
int x = 2;
int result = evaluate(head, x);
printf("The value of the polynomial at x=%d is %d\n", x, result);
return 0;
}
```
这段代码中,我们先定义了一个节点结构体,包含系数和指数两个成员变量,以及一个指向下一个节点的指针。然后定义了三个函数,分别用于创建节点、插入节点和求多项式的值。在主函数中,我们先创建了一个多项式,然后调用 evaluate 函数求出了该多项式在 x=2 时的值。
阅读全文
相关推荐






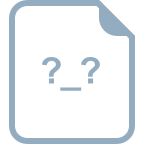
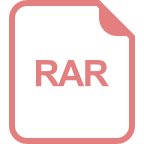
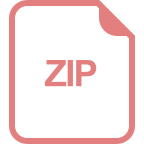