你是一位嵌入式开发工程师,请发来一份1553B的示例代码,C语言的
时间: 2023-10-02 22:09:47 浏览: 119
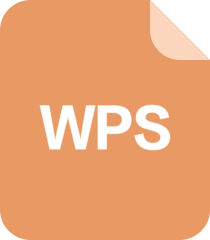
嵌入式C语言开发例题
以下是一份基于C语言实现的1553B示例代码:
```
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
// 定义1553B总线的控制寄存器地址
#define BC_CONTROL_REG 0x1000
#define RT_CONTROL_REG 0x2000
#define MT_CONTROL_REG 0x3000
// 定义1553B总线的数据寄存器地址
#define BC_DATA_REG 0x1100
#define RT_DATA_REG 0x2100
#define MT_DATA_REG 0x3100
// 定义1553B总线的命令字寄存器地址
#define BC_COMMAND_WORD_REG 0x1200
#define RT_COMMAND_WORD_REG 0x2200
#define MT_COMMAND_WORD_REG 0x3200
// 定义1553B总线的状态字寄存器地址
#define BC_STATUS_WORD_REG 0x1300
#define RT_STATUS_WORD_REG 0x2300
#define MT_STATUS_WORD_REG 0x3300
// 定义1553B总线的控制寄存器位
#define BC_ENABLE_BIT 0x0001
#define RT_ENABLE_BIT 0x0002
#define MT_ENABLE_BIT 0x0004
// 定义1553B总线的命令字寄存器位
#define BC_START_BIT 0x0001
#define RT_ENABLE_RX_BIT 0x0002
#define RT_ENABLE_TX_BIT 0x0004
#define MT_ENABLE_TX_BIT 0x0008
// 定义1553B总线的状态字寄存器位
#define RT_RX_COMPLETE_BIT 0x0001
#define RT_TX_COMPLETE_BIT 0x0002
#define MT_TX_COMPLETE_BIT 0x0004
// 定义1553B总线的命令字结构体
typedef struct {
unsigned int word_count: 5; // 数据字数
unsigned int subaddress: 5; // 子地址
unsigned int mode_code: 6; // 模式码
unsigned int reserved: 1; // 保留位
unsigned int transmit_flag: 1; // 发送标志位
unsigned int broadcast_flag: 1; // 广播标志位
unsigned int gap_time: 3; // 间隔时间
} command_word_t;
// 定义1553B总线的状态字结构体
typedef struct {
unsigned int word_count: 5; // 数据字数
unsigned int subaddress: 5; // 子地址
unsigned int mode_code: 6; // 模式码
unsigned int reserved: 1; // 保留位
unsigned int transmit_status: 1; // 发送状态位
unsigned int broadcast_received: 1; // 广播接收位
unsigned int parity_error: 1; // 奇偶校验错误位
unsigned int message_error: 1; // 消息错误位
unsigned int busy: 1; // 忙位
} status_word_t;
// 初始化1553B总线控制寄存器
void init_1553b_control_reg(unsigned int addr, unsigned int control) {
// 将控制寄存器的值写入到地址为addr的寄存器中
// control的位定义请参考上面的宏定义
}
// 初始化1553B总线命令字寄存器
void init_1553b_command_word_reg(unsigned int addr, command_word_t command_word) {
// 将命令字的值写入到地址为addr的寄存器中
}
// 读取1553B总线状态字寄存器
status_word_t read_1553b_status_word_reg(unsigned int addr) {
// 从地址为addr的寄存器中读取状态字的值
status_word_t status_word;
// 解析状态字的各个位
return status_word;
}
// 1553B总线发送数据
void send_1553b_data(unsigned int addr, unsigned int data[], int data_count) {
// 将数据写入到地址为addr的寄存器中
// 数据字数为data_count
}
// 1553B总线接收数据
void receive_1553b_data(unsigned int addr, unsigned int data[], int data_count) {
// 从地址为addr的寄存器中读取数据
// 数据字数为data_count
}
int main() {
// 初始化1553B总线控制寄存器
init_1553b_control_reg(BC_CONTROL_REG, BC_ENABLE_BIT);
init_1553b_control_reg(RT_CONTROL_REG, RT_ENABLE_RX_BIT | RT_ENABLE_TX_BIT);
init_1553b_control_reg(MT_CONTROL_REG, MT_ENABLE_TX_BIT);
// 初始化1553B总线命令字寄存器
command_word_t rt_command_word = {1, 1, 1, 0, 0, 0, 0};
init_1553b_command_word_reg(RT_COMMAND_WORD_REG, rt_command_word);
// 发送数据
unsigned int data[] = {0x1234, 0x5678};
send_1553b_data(MT_DATA_REG, data, 2);
// 等待发送完成
while (!(read_1553b_status_word_reg(MT_STATUS_WORD_REG).transmit_complete_bit));
return 0;
}
```
注意,以上代码仅供参考,具体实现可能会因不同的硬件平台、操作系统和编译器而有所不同。在实际开发过程中,建议结合相应的文档和资料进行开发。
阅读全文