C# TCP/IP通讯连接池
时间: 2024-12-11 09:43:42 浏览: 28
在C#中,TCP/IP通讯连接池是一种优化网络通信性能的技术,它允许应用程序复用已经创建好的网络连接,而不是每次需要与服务器通信时都创建新的连接。这样做可以减少创建和销毁连接所消耗的时间,提高效率,并节省系统资源。
通常,C#中的连接池会通过`System.Net.Sockets`命名空间里的`TcpClient`或`Socket`类实现。例如,你可以使用`PoolManager`库或自定义管理类来维护一个连接池:
1. 创建连接池:
```csharp
using System.Net;
using System.Net.Sockets;
using System.Collections.Generic;
public class ConnectionPool
{
private readonly List<TcpClient> _pool = new List<TcpClient>();
public TcpClient GetConnection()
{
if (_pool.Count > 0)
return _pool[0];
// 创建并初始化新连接
var client = new TcpClient();
// 设置其他属性,如超时、缓冲区大小等
return client;
}
public void ReturnConnection(TcpClient client)
{
_pool.Add(client);
}
}
```
2. 使用连接池:
```csharp
var pool = new ConnectionPool();
TcpClient client = pool.GetConnection();
// 进行网络通信...
client.Close(); // 使用完后返回到池中
```
阅读全文
相关推荐
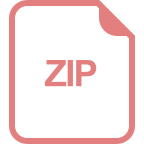
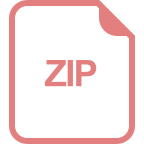
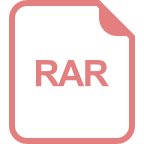















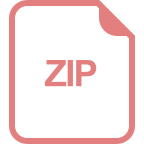