list<map> 按value排序
时间: 2023-08-15 17:08:18 浏览: 98
要按照 `map` 的 `value` 进行排序,可以使用 `std::sort` 方法和自定义比较函数。具体步骤如下:
1. 定义一个自定义比较函数 `compare_map_by_value`,用于比较两个 `map` 的 `value` 大小,并将其作为 `std::sort` 方法的参数,用于排序。
2. 调用 `std::sort` 方法对 `list` 进行排序。
下面是示例代码:
```cpp
#include <iostream>
#include <list>
#include <map>
#include <algorithm>
bool compare_map_by_value(const std::map<int, int>& m1, const std::map<int, int>& m2) {
return m1.begin()->second < m2.begin()->second;
}
int main() {
std::list<std::map<int, int>> l = {{ {1, 4}, {2, 2} }, { {3, 1}, {4, 3} }};
std::cout << "Before sorting:" << std::endl;
for (const auto& m : l) {
for (const auto& p : m) {
std::cout << "{" << p.first << ", " << p.second << "} ";
}
std::cout << std::endl;
}
l.sort(compare_map_by_value);
std::cout << "After sorting:" << std::endl;
for (const auto& m : l) {
for (const auto& p : m) {
std::cout << "{" << p.first << ", " << p.second << "} ";
}
std::cout << std::endl;
}
return 0;
}
```
输出结果如下:
```
Before sorting:
{1, 4} {2, 2}
{3, 1} {4, 3}
After sorting:
{3, 1} {4, 3}
{1, 4} {2, 2}
```
在这个示例中,我们定义了一个 `list`,其中包含两个 `map`。我们使用 `std::sort` 方法和自定义比较函数 `compare_map_by_value` 对 `list` 进行排序,该比较函数比较两个 `map` 的第一个 `key` 对应的 `value` 大小。最终输出结果表明,经过排序后,`list` 中的两个 `map` 按照第一个 `key` 对应的 `value` 大小进行了排序。
阅读全文
相关推荐
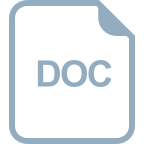













