Python selenium 坐标
时间: 2023-11-08 11:03:40 浏览: 139
可以使用 `location_once_scrolled_into_view` 属性获取元素在可见窗口中的坐标。示例如下:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.baidu.com")
# 定位百度首页的搜索框元素
search_box = driver.find_element_by_id("kw")
# 获取搜索框元素在可见窗口中的坐标
location = search_box.location_once_scrolled_into_view
print(location)
driver.quit()
```
输出:
```
{'x': 128, 'y': 210}
```
相关问题
python selenium坐标点定位
在使用 Selenium 进行页面元素定位时,可以通过坐标点定位元素。具体步骤如下:
1. 导入相关库和模块:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
```
2. 启动浏览器驱动:
```python
driver = webdriver.Chrome()
```
3. 打开要操作的网页:
```python
driver.get('https://www.example.com')
```
4. 获取要定位的元素对象:
```python
element = driver.find_element_by_xpath('//input[@id="example"]')
```
5. 构建鼠标操作对象:
```python
action = ActionChains(driver)
```
6. 将鼠标移动到元素对象所在的位置:
```python
action.move_to_element_with_offset(element, x_offset, y_offset).perform()
```
其中,x_offset 和 y_offset 分别表示元素对象左上角的横坐标和纵坐标相对于元素对象所在的位置的偏移量。
7. 在元素对象所在的位置执行鼠标操作:
```python
action.click().perform()
```
8. 关闭浏览器驱动:
```python
driver.quit()
```
python selenium 定位返回值
Python Selenium 定位返回值是指使用 Selenium 定位元素时,定位到元素后会返回一个 WebElement 对象,通过该对象可以对元素进行操作或者获取元素的属性值。WebElement 对象具有许多属性和方法,包括获取元素的文本内容、获取元素的属性值、获取元素的坐标位置、获取元素的大小等。
例如,使用 find_element_by_id 方法定位到一个 id 为 "example" 的元素,并获取该元素的文本内容和属性值可以使用以下代码:
```python
from selenium import webdriver
# 创建 WebDriver 对象
driver = webdriver.Chrome()
# 访问网页
driver.get("http://www.example.com")
# 定位元素并获取文本内容
elem = driver.find_element_by_id("example")
text = elem.text
print(text)
# 定位元素并获取属性值
attr_value = elem.get_attribute("class")
print(attr_value)
# 关闭浏览器
driver.quit()
```
阅读全文
相关推荐
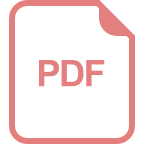
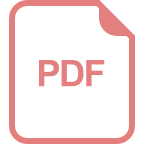
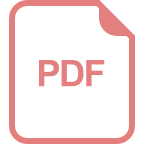













