自定义hivesink 实现RichSinkFunction
时间: 2024-03-22 13:42:32 浏览: 18
要自定义一个 Hivesink 并实现 `RichSinkFunction` 接口,可以按照以下步骤进行:
1. 继承 `RichSinkFunction` 接口,并实现其中的 `open()`、`invoke()` 和 `close()` 方法。
2. 在 `open()` 方法中创建一个 `HiveOutputFormat` 对象并初始化它。
3. 在 `invoke()` 方法中获取 Flink 中的数据,并将其转换为 Hive 表中的记录。
4. 在 `invoke()` 方法中使用 `HiveOutputFormat` 将数据写入到 Hive 中。
5. 在 `close()` 方法中关闭 `HiveOutputFormat` 对象。
以下是一个示例代码:
```
public class CustomHiveSink extends RichSinkFunction<MyRecord> {
private transient HiveOutputFormat<Text, NullWritable> outputFormat;
private String tableName;
private String[] fieldNames;
private String[] fieldTypes;
public CustomHiveSink(String tableName, String[] fieldNames, String[] fieldTypes) {
this.tableName = tableName;
this.fieldNames = fieldNames;
this.fieldTypes = fieldTypes;
}
@Override
public void open(Configuration parameters) throws Exception {
super.open(parameters);
HiveConf hiveConf = new HiveConf();
outputFormat = new HiveOutputFormat<Text, NullWritable>(hiveConf, tableName);
outputFormat.setOutput(new JobConf());
outputFormat.initialize(new JobConf());
outputFormat.setSchema(new TableSchema(fieldNames, fieldTypes));
}
@Override
public void invoke(MyRecord record, Context context) throws Exception {
// 将 Flink 中的数据转换为 Hive 表中的记录
Object[] values = new Object[fieldNames.length];
values[0] = record.getField1();
values[1] = record.getField2();
// ...
Text text = new Text(StringUtils.join(values, ","));
// 使用 HiveOutputFormat 将数据写入到 Hive 中
outputFormat.writeRecord(text, NullWritable.get());
}
@Override
public void close() throws Exception {
super.close();
outputFormat.close();
}
}
```
在 `open()` 方法中,创建一个 `HiveOutputFormat` 对象,并设置其输出表名、表结构等属性。在 `invoke()` 方法中,将 Flink 中的数据转换为 Hive 表中的记录,并使用 `HiveOutputFormat` 将数据写入到 Hive 中。在 `close()` 方法中,关闭 `HiveOutputFormat` 对象。
需要注意的是,由于 `HiveOutputFormat` 是非线程安全的,因此不能在多线程中共享同一个 `HiveOutputFormat` 对象,每个线程都需要创建自己的 `HiveOutputFormat` 对象。
相关推荐
















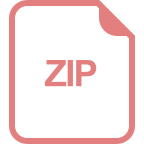