非递归算法求二叉树节点个数
时间: 2023-06-18 16:08:47 浏览: 162
非递归算法求二叉树节点个数可以使用层序遍历的方式,也叫广度优先搜索。具体步骤如下:
1. 新建一个队列,将根节点入队。
2. 当队列不为空时,取出队列头部的节点,将其左右子节点入队。
3. 对每个出队节点计数。
4. 遍历完整棵树后,返回节点数。
下面是Python实现代码:
```python
def count_nodes(root):
if not root:
return 0
count = 0
queue = [root]
while queue:
node = queue.pop(0)
count += 1
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return count
```
时间复杂度为$O(n)$,空间复杂度为$O(w)$,其中$w$是二叉树的宽度,即最大的一层节点数。
相关问题
一、问题描述 已知一棵二叉树,求该二叉树的叶结点的个数 、基本要求 (1)采用二叉链表存储二叉树。 (2)采用递归算法求二叉树中叶结点个数。 (3) 采用非递归算法求二叉树中叶结点个数 、设计提示 1.数据结构设计 根据“基本要求”,二叉树采用二叉链表存储结构 2.功能设计 完成该实验至少涉及以下 4 个功能: (1) 创建二叉树。(2)显示二叉树.用于了解创建的二叉树是否正确。 (3)采用递归算法求叶结点个数。 (4)采用非递归算法求叶结点个数
二、解决方案
1.数据结构设计
二叉树采用二叉链表存储结构,每个节点包含三个成员变量:data表示节点的数据域,left表示节点的左子树指针,right表示节点的右子树指针。
```C++
struct TreeNode {
int data;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : data(x), left(NULL), right(NULL) {}
};
```
2.功能设计
(1) 创建二叉树。
采用递归方式创建二叉树,输入-1表示该节点为空。
```C++
TreeNode* createTree() {
int val;
cin >> val;
if (val == -1) {
return NULL;
}
TreeNode* root = new TreeNode(val);
root->left = createTree();
root->right = createTree();
return root;
}
```
(2) 显示二叉树。
采用前序遍历方式显示二叉树。
```C++
void displayTree(TreeNode* root) {
if (root == NULL) {
return;
}
cout << root->data << " ";
displayTree(root->left);
displayTree(root->right);
}
```
(3) 采用递归算法求叶结点个数。
如果当前节点为空,返回0;如果当前节点为叶子节点,返回1;否则递归计算左子树和右子树的叶子节点个数之和。
```C++
int countLeafNodes(TreeNode* root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return countLeafNodes(root->left) + countLeafNodes(root->right);
}
```
(4) 采用非递归算法求叶结点个数。
采用栈来存储节点,先将根节点入栈。每次取出栈顶元素,如果该元素为叶子节点,则计数器加1,否则将其左右子节点依次入栈,直到栈为空。
```C++
int countLeafNodesNonRecursive(TreeNode* root) {
if (root == NULL) {
return 0;
}
stack<TreeNode*> s;
s.push(root);
int count = 0;
while (!s.empty()) {
TreeNode* node = s.top();
s.pop();
if (node->left == NULL && node->right == NULL) {
count++;
} else {
if (node->right != NULL) {
s.push(node->right);
}
if (node->left != NULL) {
s.push(node->left);
}
}
}
return count;
}
```
三、完整代码
```C++
#include <iostream>
#include <stack>
using namespace std;
struct TreeNode {
int data;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : data(x), left(NULL), right(NULL) {}
};
TreeNode* createTree() {
int val;
cin >> val;
if (val == -1) {
return NULL;
}
TreeNode* root = new TreeNode(val);
root->left = createTree();
root->right = createTree();
return root;
}
void displayTree(TreeNode* root) {
if (root == NULL) {
return;
}
cout << root->data << " ";
displayTree(root->left);
displayTree(root->right);
}
int countLeafNodes(TreeNode* root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return countLeafNodes(root->left) + countLeafNodes(root->right);
}
int countLeafNodesNonRecursive(TreeNode* root) {
if (root == NULL) {
return 0;
}
stack<TreeNode*> s;
s.push(root);
int count = 0;
while (!s.empty()) {
TreeNode* node = s.top();
s.pop();
if (node->left == NULL && node->right == NULL) {
count++;
} else {
if (node->right != NULL) {
s.push(node->right);
}
if (node->left != NULL) {
s.push(node->left);
}
}
}
return count;
}
int main() {
TreeNode* root = createTree();
displayTree(root);
cout << endl;
cout << "Number of leaf nodes (recursive): " << countLeafNodes(root) << endl;
cout << "Number of leaf nodes (non-recursive): " << countLeafNodesNonRecursive(root) << endl;
return 0;
}
```
非递归算法求二叉树的叶结点个数C++
可以使用迭代法(非递归算法)来求解二叉树的叶结点个数,具体思路如下:
1.定义一个计数器 count,初始化为0。
2.使用栈来遍历二叉树。首先将根节点入栈。
3.进入循环,当栈不为空时执行以下操作:
- 弹出栈顶元素,如果该元素为叶结点,则将 count 加1。
- 如果该元素有右子树,则将右子树入栈。
- 如果该元素有左子树,则将左子树入栈。
4.遍历结束后,返回 count 值即可。
下面是C++代码实现:
```c++
int countLeaves(TreeNode* root) {
if (!root) {
return 0;
}
int count = 0;
stack<TreeNode*> st;
st.push(root);
while (!st.empty()) {
TreeNode* node = st.top();
st.pop();
if (!node->left && !node->right) {
count++;
}
if (node->right) {
st.push(node->right);
}
if (node->left) {
st.push(node->left);
}
}
return count;
}
```
其中,TreeNode 表示二叉树的节点。
阅读全文
相关推荐


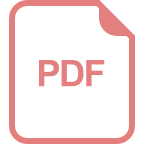



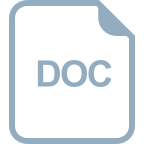





