python程序封装成dll
时间: 2023-10-16 10:19:53 浏览: 49
要将 Python 程序封装成 DLL,可以使用 Python 的 C API 和 Windows 的 DLL 功能。具体来说,可以按照以下步骤进行操作:
1. 在 C++ 代码中包含 Python.h 头文件。
2. 使用 Py_Initialize() 函数初始化 Python 解释器。
3. 使用 PyRun_SimpleString() 函数或 PyRun_File() 函数执行 Python 代码。
4. 将 Python 代码封装成 DLL。
以下是一个简单的例子,演示如何将 Python 程序封装成 DLL:
```c++
#include <Python.h>
#include <Windows.h>
// Python 代码
const char* python_code = "def add(a, b):\n return a + b\n";
// DLL 入口函数
BOOL APIENTRY DllMain(HMODULE hModule,
DWORD ul_reason_for_call,
LPVOID lpReserved)
{
switch (ul_reason_for_call)
{
case DLL_PROCESS_ATTACH:
// 初始化 Python 解释器
Py_Initialize();
// 执行 Python 代码
PyRun_SimpleString(python_code);
break;
case DLL_THREAD_ATTACH:
case DLL_THREAD_DETACH:
break;
case DLL_PROCESS_DETACH:
// 关闭 Python 解释器
Py_Finalize();
break;
}
return TRUE;
}
// 封装的函数
__declspec(dllexport) int add(int a, int b)
{
PyObject* pName = PyUnicode_DecodeFSDefault("__main__");
PyObject* pModule = PyImport_Import(pName);
Py_DECREF(pName);
if (pModule != NULL) {
PyObject* pFunc = PyObject_GetAttrString(pModule, "add");
if (pFunc && PyCallable_Check(pFunc)) {
PyObject* pArgs = PyTuple_New(2);
PyTuple_SetItem(pArgs, 0, PyLong_FromLong(a));
PyTuple_SetItem(pArgs, 1, PyLong_FromLong(b));
PyObject* pResult = PyObject_CallObject(pFunc, pArgs);
Py_DECREF(pArgs);
if (pResult != NULL) {
int result = (int)PyLong_AsLong(pResult);
Py_DECREF(pResult);
return result;
}
else {
Py_DECREF(pFunc);
Py_DECREF(pModule);
PyErr_Print();
return -1;
}
}
else {
if (PyErr_Occurred())
PyErr_Print();
return -1;
}
}
else {
PyErr_Print();
return -1;
}
}
```
在上面的例子中,我们使用 Py_Initialize() 函数初始化 Python 解释器,使用 PyRun_SimpleString() 函数执行 Python 代码,然后将 Python 代码封装成 DLL。我们定义了一个 add() 函数,该函数调用了 Python 程序中的 add() 函数,实现了两个整数相加的功能。
要使用该 DLL,可以在 C++ 代码中使用 LoadLibrary() 函数加载该 DLL,然后使用 GetProcAddress() 函数获取 add() 函数的地址,并调用该函数即可。
相关推荐
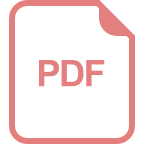
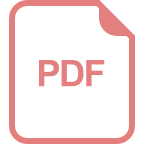














