#include <iostream>#include <thre
时间: 2024-08-15 18:05:44 浏览: 54
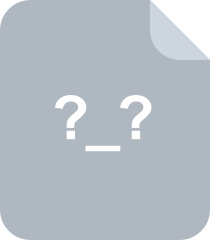
DDY游戏大合集.cpp
看起来您提供的代码片段似乎是C++相关的,但是您给出的`#include <thre>`并不是标准的C++头文件。在C++中,我们通常会包括像`<iostream>`这样的库来实现基本输入输出操作。
如果您想要了解如何使用C++进行基本的输入输出,下面是一个简单的示例:
### 示例代码:
```cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
```
这段代码展示了如何使用`iostream`库来进行打印输出以及结束程序。
### 相关问题:
1. 如何在C++中读取用户的输入并存储到变量中?
2. `std::endl`与`\n`有什么区别?
3. C++中的命名空间是如何工作的?
阅读全文
相关推荐
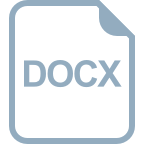
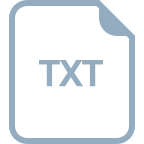








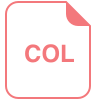







