写一个linux GPIO 设备树驱动代码
时间: 2024-10-19 09:08:59 浏览: 69
在Linux内核中,GPIO(General Purpose Input/Output,通用输入/输出)设备树驱动主要是为了管理和控制系统板上的GPIO引脚。编写这样的驱动通常涉及到以下几个步骤:
1. **结构声明**:
首先,你需要定义一组数据结构来表示GPIO引脚的状态和配置信息,如`struct gpio_chip`。
```c
struct gpiochip {
const char *name; /* GPIO名称 */
int label_count; /* 管理的GPIO数量 */
unsigned long (*get)(void *, unsigned);
void (*set)(void *, unsigned);
void (*set_dir)(void *, unsigned, bool);
...
};
```
2. **注册函数**:
通过`gpiochip_add()`或类似函数将GPIO描述符注册到内核中。
```c
static struct gpiochip my_gpio = { ... };
int __init gpiochip_init(void) {
return gpiochip_add(&my_gpio, -1); // -1 表示自动查找ID
}
```
3. **初始化操作**:
初始化GPIO,设置其方向(输入/输出),并可能映射硬件寄存器。
```c
static int __init gpiochip_probe(struct platform_device *pdev) {
struct resource *res;
gpiochip_set_direction(&my_gpio, ...); // 设置所有GPIO为输入/输出
res = platform_get_resource(pdev, IORESOURCE_GPIO, 0);
gpiochip_add_resources(&my_gpio, &res->start, 1); // 添加GPIO资源
...
return gpiochip_register(&my_gpio);
}
```
4. **处理中断**(如果需要):
如果GPIO用于中断,需要注册中断处理函数,并在内核初始化时开启中断。
```c
static irqreturn_t gpio_handler(int irq, void *dev_id) {
struct gpiochip *gc = dev_id;
...
}
static int gpiochip_irq_set_type(struct gpiochip *gc, unsigned offset, ...)
```
5. **清理工作**:
在模块卸载时,记得释放GPIO资源并注销驱动。
```c
static void gpiochip_remove(struct platform_device *pdev) {
gpiochip_unregister(&my_gpio);
gpiochip_free_resources(&my_gpio);
}
```
阅读全文
相关推荐
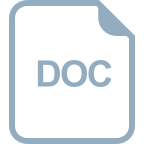
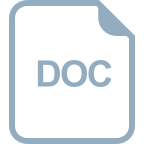
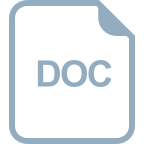
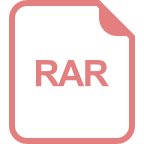
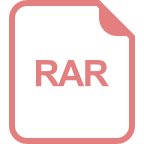
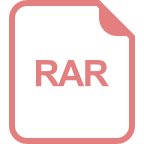
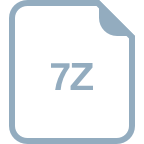





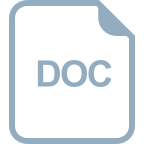
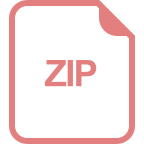
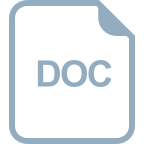
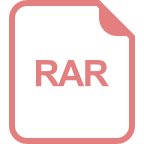
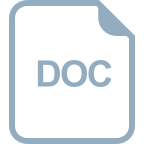
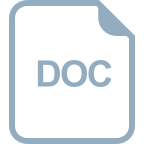
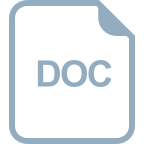