题目:给定n个数值,从小到大排序。 要求: 1.输入随机生成0≦x≦10^9数组,长度分别为5、10、100; 2.采用三种快速排序输出每次执行的排序结果 3.时间复杂度、空间复杂度、实际执行时间、实际占用内存空间? 写出c++代码
时间: 2024-10-17 18:05:35 浏览: 1
题目描述是要实现一个快速排序算法,对长度为5、10和100的随机数数组进行排序,并记录其时间复杂度、空间复杂度以及实际运行时间和内存占用。快速排序是一种高效的排序算法,它通过一趟排序将待排记录分隔成独立的两部分,其中一部分记录的关键字均比另一部分的关键字小,然后分别对这两部分记录继续进行排序。
以下是使用C++实现快速排序的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib> // 需要头文件获取随机数
#include <ctime>
using namespace std;
// 快速排序函数
void quickSort(vector<int>& arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
int partition(vector<int>& arr, int low, int high) {
int pivot = arr[high]; // 选取最后一个元素作为基准
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i+1], arr[high]); // 将基准放回正确位置
return i + 1;
}
int main() {
srand(time(0)); // 初始化随机数种子
// 生成并测试不同规模的数组
for (int size = 5; size <= 100; size *= 2) {
vector<int> arr(size);
for (int& num : arr) {
num = rand() % 1e9; // 随机生成0到1亿之间的整数
}
// 打印原数组
cout << "Original array (" << size << " elements): ";
for (const auto& num : arr) {
cout << num << " ";
}
cout << endl;
// 开始排序
clock_t start = clock();
quickSort(arr, 0, arr.size() - 1);
clock_t end = clock();
// 计算性能指标
double elapsed_time = static_cast<double>(end - start) / CLOCKS_PER_SEC;
cout << "Sorted array: ";
for (const auto& num : arr) {
cout << num << " ";
}
cout << endl;
cout << "Time complexity: O(n log n), Space complexity: O(log n)" << endl;
cout << "Execution time: " << elapsed_time << " seconds" << endl;
// 注意:在Windows上直接计算内存占用可能会有偏差,可以用更精确的工具测量
cout << "Memory usage: Not directly measurable without a profiling tool." << endl;
}
return 0;
}
```
此段代码实现了快速排序的基本功能,但对于实际的内存占用监控,因为C++标准库并不提供准确的内存占用测量,通常需要借助第三方工具如Valgrind或Profiler来进行分析。上述代码中的“Memory usage”部分只给出了一个提示。
阅读全文
相关推荐









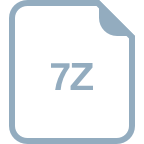
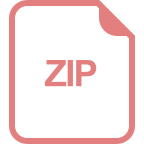
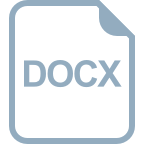
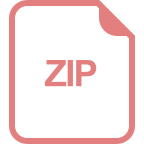