cv2.imwrite(file_path, frame)中文路肩
时间: 2024-04-22 19:25:21 浏览: 211
在使用 `cv2.imwrite()` 保存图像时,如果你的文件路径包含中文字符,可能会遇到编码问题。为了避免这个问题,你可以使用 Unicode 字符串来表示文件路径。
以下是一个示例,展示了如何在文件路径中包含中文字符:
```python
import cv2
import os
# 创建保存图像的文件夹
save_dir = '保存路径'
if not os.path.exists(save_dir):
os.makedirs(save_dir)
# 从摄像头读取图像
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
# 保存图像到指定文件夹
file_path = os.path.join(save_dir, '图片.png')
cv2.imwrite(file_path, frame)
# 释放摄像头资源
cap.release()
```
在这个示例中,我们创建了一个名为 "保存路径" 的文件夹,并将图像保存为 "图片.png"。我们使用 `os.path.join()` 方法来拼接路径,并确保中文字符的正确性。
请注意,不同操作系统对于中文路径的支持可能有所不同。如果你在某些操作系统上仍然遇到问题,建议使用英文字符或非中文字符作为文件路径的一部分。
相关问题
def process_image(image_path, output_folder): gray = cv2.imread(image_path) edges = cv2.Canny(gray, 50, 150) lines = cv2.HoughLines(edges, 1, np.pi / 180, threshold=90) sum_angle = 0 count = 0 if lines is not None: for line in lines: rho, theta = line[0] angle = theta * 180 / np.pi sum_angle += angle count += 1 avg_angle = sum_angle / count rotated_image = rotate_image(gray, avg_angle) filename = os.path.basename(image_path) output_path = os.path.join(output_folder, filename) cv2.imwrite(output_path, rotated_image) def rotate_image(image, angle): height, width = image.shape[:2] center = (width // 2, height // 2) rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1.0) rotated_image = cv2.warpAffine(image, rotation_matrix, (width, height)) return rotated_image input_folder = 'img_out_binary' output_folder = 'rotated_img' os.makedirs(output_folder, exist_ok=True) for filename in os.listdir(input_folder): if filename.endswith(".jpg") or filename.endswith(".JPG"): image_path = os.path.join(input_folder, filename) process_image(image_path, output_folder) 优化程序,使程序可以对图片文件名包含汉字的图片进行处理和保存
要使程序能够处理和保存包含汉字的图片文件名,您可以对`process_image()`函数进行修改。在处理图片之前,您可以对文件名进行URL编码,然后使用编码后的文件名来保存旋转后的图片。以下是修改后的代码:
```python
def process_image(image_path, output_folder):
# 对文件名进行URL编码
encoded_filename = urllib.parse.quote(os.path.basename(image_path))
gray = cv2.imread(image_path)
edges = cv2.Canny(gray, 50, 150)
lines = cv2.HoughLines(edges, 1, np.pi / 180, threshold=90)
sum_angle = 0
count = 0
if lines is not None:
for line in lines:
rho, theta = line[0]
angle = theta * 180 / np.pi
sum_angle += angle
count += 1
avg_angle = sum_angle / count
rotated_image = rotate_image(gray, avg_angle)
# 使用编码后的文件名来保存旋转后的图片
output_path = os.path.join(output_folder, encoded_filename)
cv2.imwrite(output_path, rotated_image)
```
在修改后的代码中,首先使用`urllib.parse.quote()`函数对文件名进行编码,然后使用编码后的文件名来构建输出路径。这样可以确保保存的文件名不会受到包含汉字的影响。
另外,确保在程序中正确导入`urllib.parse`模块来使用URL编码函数。
def CaptureImages(window_name,student_id): capture_pic_num = 100 folder_path = f"C:/Users/DELL/PycharmProjects/pythonProject/{student_id}" os.makedirs(folder_path, exist_ok=True) cv2.namedWindow(window_name) cap = cv2.VideoCapture(0) classifier = cv2.CascadeClassifier("haarcascade_frontalface_alt2.xml") color = (0, 255, 0) num = 0 while cap.isOpened(): ret, frame = cap.read() if not ret: break gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = classifier.detectMultiScale(gray, scaleFactor=1.2, minNeighbors=3, minSize=(32, 32)) if len(faces) > 0: for (x, y, w, h) in faces: img_path = f"{folder_path}/{num}.jpg" image = frame[y - 10:y + h + 10, x - 10:x + w + 10] cv2.imwrite(img_path, image) num += 1 cv2.rectangle(frame, (x - 10, y - 10), (x + w + 10, y + h + 10), color, 2) font = cv2.FONT_HERSHEY_SIMPLEX cv2.putText(frame, f'num:{num}', (x + 30, y + 30), font, 1, (255, 0, 255), 4) if num >= capture_pic_num: break cv2.imshow(window_name, frame) if cv2.waitKey(30) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows()怎么将这个功能与一个qt界面的按钮相连接
要将这个功能与一个Qt界面的按钮相连接,你需要按照以下步骤进行操作:
1. 导入必要的模块和库:
```python
import cv2
import os
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QVBoxLayout, QWidget
from PyQt5.QtCore import Qt, QThread
from PyQt5.QtGui import QImage, QPixmap
```
2. 创建一个 Qt 主窗口类(例如 `MainWindow`):
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Capture Images")
self.setFixedSize(300, 200)
# 创建按钮并连接到槽函数
self.capture_btn = QPushButton("Capture", self)
self.capture_btn.clicked.connect(self.start_capture)
# 创建布局,将按钮放入布局中,并设置布局为窗口的中心布局
layout = QVBoxLayout()
layout.addWidget(self.capture_btn)
central_widget = QWidget()
central_widget.setLayout(layout)
self.setCentralWidget(central_widget)
# 按钮点击事件的槽函数
def start_capture(self):
# 在这里调用 CaptureImages 函数进行图像捕获
CaptureImages("Capture Images", "student_id")
```
3. 创建一个线程类(例如 `CaptureThread`),用于在后台执行图像捕获操作:
```python
class CaptureThread(QThread):
def run(self):
CaptureImages("Capture Images", "student_id")
```
4. 在主程序中创建 Qt 应用程序并启动主窗口:
```python
if __name__ == "__main__":
app = QApplication([])
main_window = MainWindow()
main_window.show()
app.exec_()
```
这样,当你点击按钮时,它将调用 `start_capture` 槽函数,然后在后台启动一个线程来执行 `CaptureImages` 函数进行图像捕获操作。请注意,你需要将 `student_id` 更改为实际的学生ID。
希望这可以帮助到你!如果你有任何其他问题,请随时问我。
阅读全文
相关推荐
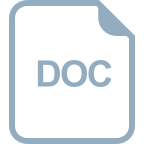
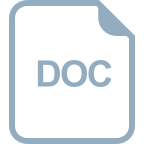
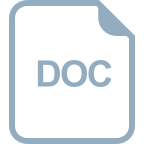














