遗传算法路径规划python
时间: 2023-07-31 15:06:59 浏览: 104
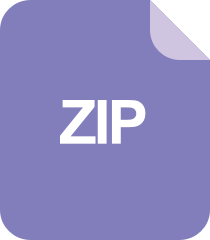
Python-遗传算法路径规划及仿真
遗传算法是一种优化算法,可用于解决路径规划问题。在Python中,您可以使用遗传算法库进行路径规划的实现。以下是一个简单的示例:
```python
import random
# 定义路径规划问题
# 假设我们有一个包含城市坐标的列表,需要找到最短路径经过所有城市并回到起点
city_list = [(2, 4), (5, 7), (8, 3), (1, 6), (9, 2)]
# 定义遗传算法参数
population_size = 100 # 种群大小
elite_size = 20 # 精英个体数量
mutation_rate = 0.01 # 变异率
generations = 100 # 迭代次数
# 创建一个路径类表示个体
class Path:
def __init__(self, path):
self.path = path
self.distance = 0
def calculate_distance(self):
total_distance = 0
for i in range(len(self.path) - 1):
city1 = self.path[i]
city2 = self.path[i + 1]
total_distance += ((city2[0] - city1[0]) ** 2 + (city2[1] - city1[1]) ** 2) ** 0.5
self.distance = total_distance
# 创建初始种群
def create_population():
population = []
for _ in range(population_size):
path = random.sample(city_list, len(city_list))
population.append(Path(path))
return population
# 计算每个个体的适应度(路径长度)
def calculate_fitness(population):
for path in population:
path.calculate_distance()
# 选择精英个体
def select_elite(population):
sorted_population = sorted(population, key=lambda x: x.distance)
return sorted_population[:elite_size]
# 交叉操作
def crossover(parent1, parent2):
child = []
gene_a = int(random.random() * len(parent1.path))
gene_b = int(random.random() * len(parent1.path))
start_gene = min(gene_a, gene_b)
end_gene = max(gene_a, gene_b)
for i in range(start_gene, end_gene):
child.append(parent1.path[i])
for city in parent2.path:
if city not in child:
child.append(city)
return Path(child)
# 变异操作
def mutate(path):
for _ in range(len(path.path)):
if random.random() < mutation_rate:
index1 = int(random.random() * len(path.path))
index2 = int(random.random() * len(path.path))
path.path[index1], path.path[index2] = path.path[index2], path.path[index1]
# 迭代进化
def evolve(population):
elite = select_elite(population)
new_population = elite
while len(new_population) < population_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = crossover(parent1, parent2)
mutate(child)
new_population.append(child)
return new_population
# 主函数
def main():
population = create_population()
for _ in range(generations):
calculate_fitness(population)
population = evolve(population)
best_path = min(population, key=lambda x: x.distance)
print("最短路径:", best_path.path)
print("最短路径长度:", best_path.distance)
if __name__ == '__main__':
main()
```
上述代码演示了如何使用遗传算法解决路径规划问题。您可以根据实际需求进行扩展和修改。希望对您有所帮助!
阅读全文
相关推荐
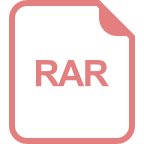
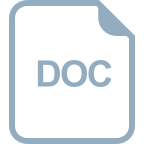









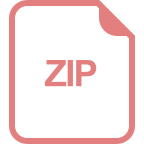
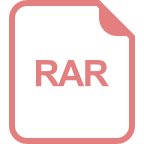
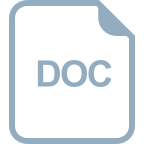



