遗传算法python路径规划
时间: 2023-12-21 13:32:04 浏览: 119
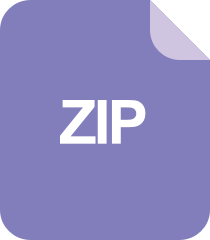
应用遗传算法求解机器人路径规划问题Python代码程序
遗传算法是一种优化算法,可以用于解决路径规划问题。在Python中,可以使用遗传算法来进行路径规划的仿真和优化。
以下是使用遗传算法进行路径规划的一般步骤:
1. 创建地图:首先,需要创建一个地图来表示机器人移动的环境。可以使用栅格图法或其他方法来建模地图。
2. 初始化种群:接下来,需要初始化一个种群,种群中的个体表示可能的路径解。每个个体都是一个路径序列,表示机器人从起点到终点的移动路径。
3. 计算适应度:对于每个个体,需要计算其适应度函数值,以评估其路径的质量。适应度函数可以根据具体问题进行定义,例如路径长度、避免障碍物等。
4. 选择:根据适应度函数值,选择一部分个体作为父代,用于产生下一代个体。常见的选择方法有轮盘赌选择、锦标赛选择等。
5. 交叉:通过交叉操作,将父代个体的基因信息进行组合,生成新的个体。交叉操作可以使用单点交叉、多点交叉等方法。
6. 变异:对于新生成的个体,可以进行变异操作,以增加种群的多样性。变异操作可以随机改变个体的某些基因信息。
7. 更新种群:根据选择、交叉和变异操作,生成新的种群,并替换原来的种群。
8. 输出结果:迭代上述步骤,直到达到停止条件。最终,可以选择适应度最高的个体作为最优路径解,并输出结果。
以下是一个使用遗传算法进行路径规划的Python代码示例:
```python
# 导入相关库
import random
# 创建地图
map = [[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 0, 0]]
# 初始化种群
population_size = 50
chromosome_length = 10
population = []
for _ in range(population_size):
chromosome = [random.randint(0, 4) for _ in range(chromosome_length)]
population.append(chromosome)
# 计算适应度
def fitness(chromosome):
x, y = 0, 0
path_length = 0
for gene in chromosome:
if gene == 0: # 向上移动
if y > 0 and map[y-1][x] == 0:
y -= 1
path_length += 1
elif gene == 1: # 向下移动
if y < len(map)-1 and map[y+1][x] == 0:
y += 1
path_length += 1
elif gene == 2: # 向左移动
if x > 0 and map[y][x-1] == 0:
x -= 1
path_length += 1
elif gene == 3: # 向右移动
if x < len(map[0])-1 and map[y][x+1] == 0:
x += 1
path_length += 1
return path_length
fitness_values = [fitness(chromosome) for chromosome in population]
# 选择
def selection(population, fitness_values):
total_fitness = sum(fitness_values)
probabilities = [fitness_value / total_fitness for fitness_value in fitness_values]
selected_population = random.choices(population, probabilities, k=population_size)
return selected_population
selected_population = selection(population, fitness_values)
# 交叉
def crossover(parent1, parent2):
crossover_point = random.randint(1, chromosome_length-1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异
def mutation(chromosome, mutation_rate):
for i in range(chromosome_length):
if random.random() < mutation_rate:
chromosome[i] = random.randint(0, 4)
return chromosome
# 更新种群
def update_population(population, fitness_values, mutation_rate):
selected_population = selection(population, fitness_values)
new_population = []
for i in range(population_size // 2):
parent1 = selected_population[i]
parent2 = selected_population[population_size-i-1]
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
new_population.append(child1)
new_population.append(child2)
return new_population
mutation_rate = 0.01
population = update_population(population, fitness_values, mutation_rate)
# 输出结果
best_chromosome = max(population, key=fitness)
best_path = [gene for gene in best_chromosome if gene != 4]
print("Best path:", best_path)
print("Path length:", fitness(best_chromosome))
```
阅读全文
相关推荐
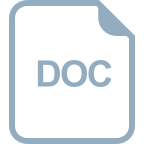
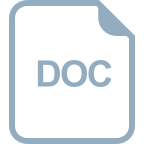










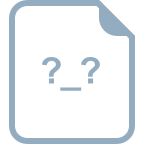
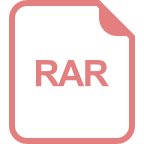
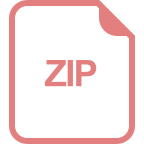


