用nodejs完成需求:模拟接口,前端异步请求接口,检验数据是否可用。get请求和post请求所获取的请求不一样。get请求返回["zs","ls","ww"],post请求返回["张三","李四","王五"]。html页面要求是"<input oninput=""></input><span></span>",防抖异步请求接口数据,span里显示数据是否可用,异步使用axios,其他操作使用原生js,并解决跨域问题
时间: 2023-07-02 22:23:47 浏览: 92
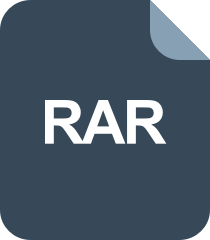
html页面模拟测试get/post请求

好的,我会根据您的要求来完成这个需求。首先,我们需要使用Node.js来搭建一个简单的服务器,模拟接口,代码如下:
```javascript
const http = require('http');
const server = http.createServer((req, res) => {
if (req.method === 'GET') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.write(JSON.stringify(['zs', 'ls', 'ww']));
res.end();
} else if (req.method === 'POST') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.write(JSON.stringify(['张三', '李四', '王五']));
res.end();
}
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
接下来,在前端页面中,我们需要使用`axios`来异步请求该接口,代码如下:
```html
<input oninput="debounce(fetchData, 500)" />
<span id="result"></span>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script>
const input = document.querySelector('input');
const result = document.querySelector('#result');
function debounce(func, delay) {
let timeoutId;
return function () {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, arguments);
}, delay);
};
}
function fetchData() {
const value = input.value;
axios
.get(`http://localhost:3000?value=${value}`)
.then((response) => {
const data = response.data;
result.textContent = data.includes(value) ? '可用' : '不可用';
})
.catch((error) => {
console.error(error);
});
}
</script>
```
以上代码中,我们使用`debounce`函数来进行防抖操作,防止用户频繁输入,导致接口请求过多。同时,我们使用`axios`库来异步请求接口数据。在请求成功后,我们判断输入的值是否存在于返回的数据中,如果存在,则说明该数据可用,否则不可用。最后,将结果显示在`span`标签中。
由于我们的后端接口运行在`localhost:3000`上,而前端页面运行在另一个端口上,因此需要解决跨域问题。可以在后端代码中添加如下代码:
```javascript
res.setHeader('Access-Control-Allow-Origin', '*');
```
它允许所有的域名都可以访问该接口。完整的后端代码如下:
```javascript
const http = require('http');
const server = http.createServer((req, res) => {
res.setHeader('Access-Control-Allow-Origin', '*');
if (req.method === 'GET') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.write(JSON.stringify(['zs', 'ls', 'ww']));
res.end();
} else if (req.method === 'POST') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.write(JSON.stringify(['张三', '李四', '王五']));
res.end();
}
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
至此,我们就完成了模拟接口、异步请求接口、检验数据是否可用,并解决了跨域问题的需求。
阅读全文
相关推荐
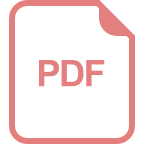

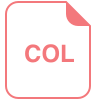
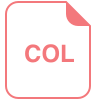
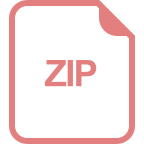
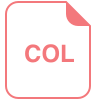
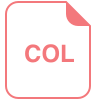
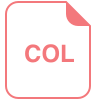
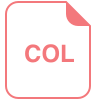
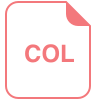
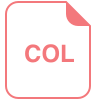
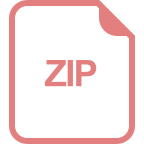
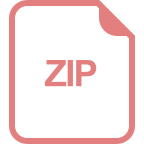
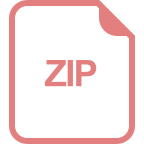
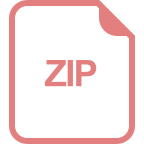
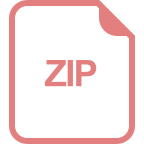
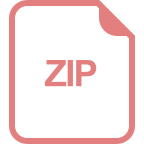