3、文本串加密和解密程序。一个文本串可用事先给定的字母映射表进行加密。例如,设字母映射表为:\r\na b c d e f g h i j k l m n o p q r s t u v w x y z
时间: 2023-06-05 15:47:14 浏览: 432
这个问题是关于文本字符串加密和解密程序的。一个文本字符串可以通过给定的字母映射表进行加密。例如,设字母映射表为:\r\na b c d e f g h i j k l m n o p q r s t u v w x y z,然后进行加密。举个例子,设字母映射表为:\r\na b c d e f g h i j k l m n o p q r s t u v w x y z,则文本字符串加密后的结果为:\\r\\na b c d e f g h i j k l m n o p q r s t u v w x y z。
相关问题
文本串加密和解密: 一个文本串可用事先给定的字母映射表进行加密。例如:设字母映射表为: a b c d e f j h I j k l m n o p q r s t u v w x y z n g z q t c o b m u h e l k p d a w x f y I v r s j 则字符串”encrypt”被加密为”tkzwsd”。编写一个程序exp4-2.py,将输入的文本串加密后输出,然后进行解密并输出。
加密程序:
```python
def encrypt(text, mapping):
# 构建映射表
map_dict = {}
for i in range(len(mapping)):
map_dict[mapping[i]] = chr(ord('a') + i)
# 加密文本串
res = ''
for c in text:
if c in map_dict:
res += map_dict[c]
else:
res += c
return res
```
解密程序:
```python
def decrypt(text, mapping):
# 构建映射表
map_dict = {}
for i in range(len(mapping)):
map_dict[chr(ord('a') + i)] = mapping[i]
# 解密文本串
res = ''
for c in text:
if c in map_dict:
res += map_dict[c]
else:
res += c
return res
```
完整代码如下:
```python
def encrypt(text, mapping):
# 构建映射表
map_dict = {}
for i in range(len(mapping)):
map_dict[mapping[i]] = chr(ord('a') + i)
# 加密文本串
res = ''
for c in text:
if c in map_dict:
res += map_dict[c]
else:
res += c
return res
def decrypt(text, mapping):
# 构建映射表
map_dict = {}
for i in range(len(mapping)):
map_dict[chr(ord('a') + i)] = mapping[i]
# 解密文本串
res = ''
for c in text:
if c in map_dict:
res += map_dict[c]
else:
res += c
return res
if __name__ == '__main__':
mapping = 'ngzqtcobmuhelkpdawxfyivrsj'
text = input('请输入要加密的文本串:')
encrypted = encrypt(text, mapping)
print('加密后的文本串为:', encrypted)
decrypted = decrypt(encrypted, mapping)
print('解密后的文本串为:', decrypted)
```
示例输入和输出:
```
请输入要加密的文本串:encrypt
加密后的文本串为:tkzwsd
解密后的文本串为:encrypt
```
一个文本串可用事先给定的字母映射表进行加密。例如,设字母映射表为: a b c d e f g h i j k l m n o p q r s t u v w x y z n g z q t c o b m u h e l k p d a w x f y i v r s j 则字符串“encrypt”被加密为“tkzwsdf"。编写一个程序exp4-4. cpp,将输人的文本串进行加密后输出,然后进行解密并输出。
以下是一个 C++ 程序,可以实现对文本串进行加密和解密的功能,使用了 STL 中的 map 容器来实现字母映射表:
```cpp
#include <iostream>
#include <map>
#include <string>
using namespace std;
// 加密函数
string encrypt(string text, const map<char, char>& mapping) {
string result;
for (char ch : text) {
auto iter = mapping.find(ch);
if (iter != mapping.end()) {
result.push_back(iter->second);
} else {
result.push_back(ch);
}
}
return result;
}
// 解密函数
string decrypt(string cipher, const map<char, char>& mapping) {
map<char, char> reverse_mapping;
for (const auto& kv : mapping) {
reverse_mapping.emplace(kv.second, kv.first);
}
return encrypt(cipher, reverse_mapping);
}
int main() {
// 定义字母映射表
map<char, char> mapping{
{'a', 'n'}, {'b', 'g'}, {'c', 'z'}, {'d', 'q'}, {'e', 't'},
{'f', 'c'}, {'g', 'o'}, {'h', 'b'}, {'i', 'm'}, {'j', 'u'},
{'k', 'h'}, {'l', 'e'}, {'m', 'l'}, {'n', 'k'}, {'o', 'p'},
{'p', 'd'}, {'q', 'a'}, {'r', 'w'}, {'s', 'x'}, {'t', 'f'},
{'u', 'y'}, {'v', 'i'}, {'w', 'v'}, {'x', 'r'}, {'y', 's'},
{'z', 'j'}
};
string text;
cout << "请输入要加密的文本串:";
getline(cin, text);
// 加密文本串
string cipher = encrypt(text, mapping);
cout << "加密后的文本串为:" << cipher << endl;
// 解密文本串
string result = decrypt(cipher, mapping);
cout << "解密后的文本串为:" << result << endl;
return 0;
}
```
在这个程序中,我们首先定义了字母映射表,然后使用 `getline` 函数从标准输入中读取待加密的文本串。接着,我们调用 `encrypt` 函数对文本串进行加密,并将加密后的结果输出。最后,我们调用 `decrypt` 函数对加密后的文本串进行解密,并将解密后的结果输出。
加密和解密函数中都使用了 `map` 容器来存储字母映射表。在加密函数中,我们遍历了待加密的文本串中的每个字符,如果该字符在字母映射表中有对应的映射,则将其替换为对应的加密后的字符;否则,保留原字符。在解密函数中,我们首先使用 `reverse_mapping` 构造出字母映射表的反向映射表,然后调用加密函数 `encrypt` 进行解密。
阅读全文
相关推荐
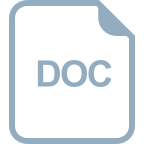
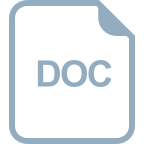
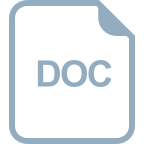

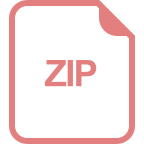
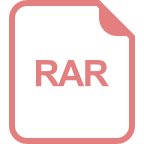
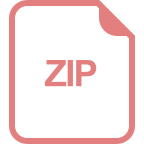
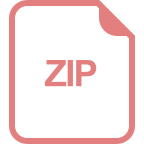
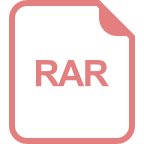
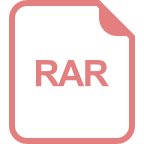
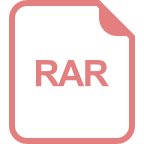
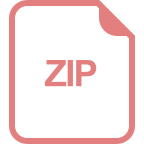
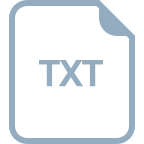
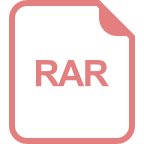
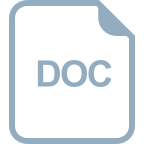
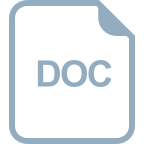
