用python程序使用 key 作为参数,对给定的文本文件执行加密运算,加密后的文件输出到另一 文本文件中,加密算法是对于文件中的每个字母,用字母表中其后第 n 个字母来替代,其中 n 为密 钥。加密后的文件可以用密钥-n 来解密
时间: 2024-03-14 18:45:31 浏览: 64
好的,这是一个关于加密和解密的问题。首先,我们需要编写一个加密函数,它将接收文本文件路径和密钥作为参数,并对文件中的每个字母进行加密。然后,我们需要将加密后的结果写入到另一个文件中。
```python
def encrypt_file(file_path, key):
with open(file_path, 'r') as file:
text = file.read()
encrypted_text = ""
for char in text:
if char.isalpha():
shifted_char = chr((ord(char.lower()) - 97 + key) % 26 + 97)
encrypted_text += shifted_char.upper() if char.isupper() else shifted_char
else:
encrypted_text += char
with open(f'encrypted_{file_path}', 'w') as encrypted_file:
encrypted_file.write(encrypted_text)
```
上述代码中,我们打开了一个文件并读取了其中的文本。然后,我们对文本文件中的每个字母进行加密,将加密后的结果存储到一个新的字符串变量中。最后,我们将加密后的结果写入到一个名为 encrypted_file 的新文件中。
接下来,我们需要编写一个解密函数,它将接收加密后的文件路径和密钥作为参数,并对文件中的每个字母进行解密。然后,我们需要将解密后的结果写入到另一个文件中。
```python
def decrypt_file(file_path, key):
with open(file_path, 'r') as file:
text = file.read()
decrypted_text = ""
for char in text:
if char.isalpha():
shifted_char = chr((ord(char.lower()) - 97 - key) % 26 + 97)
decrypted_text += shifted_char.upper() if char.isupper() else shifted_char
else:
decrypted_text += char
with open(f'decrypted_{file_path}', 'w') as decrypted_file:
decrypted_file.write(decrypted_text)
```
上述代码中,我们打开了一个加密后的文件并读取了其中的文本。然后,我们对文件中的每个字母进行解密,将解密后的结果存储到一个新的字符串变量中。最后,我们将解密后的结果写入到一个名为 decrypted_file 的新文件中。
现在,我们可以使用这些函数来加密和解密文件了。假设我们有一个名为 input.txt 的文件,我们可以使用以下代码来对它进行加密和解密:
```python
key = 3 # 密钥
file_path = 'input.txt'
# 加密文件
encrypt_file(file_path, key)
# 解密文件
decrypt_file(f'encrypted_{file_path}', key)
```
这样,我们就成功地对文件进行了加密和解密操作。
阅读全文
相关推荐
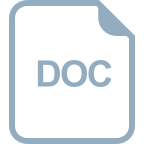
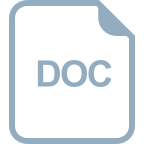
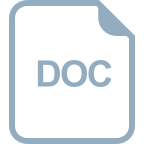

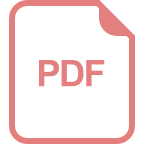
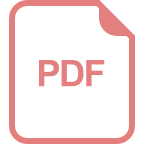
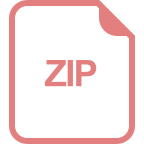
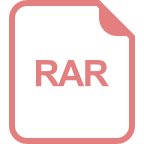
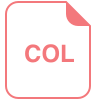
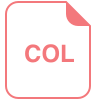
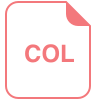
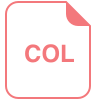
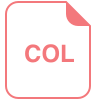
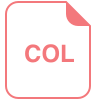
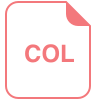



