Python代码 编写程序实现对明文cipher的k=7,b=6的仿射加密和解密。
时间: 2024-10-21 16:11:15 浏览: 40
在Python中,我们可以使用简单的算术运算来实现仿射加密(Affine Cipher)。这是一个线性替换密码,它通过将字母表中的每个字符替换为其偏移量的某种函数得到。对于给定的k值(加密因子)和b值(偏移量),加密和解密的过程如下:
```python
def encrypt_char(char, k, b):
# 将字母映射到整数,然后进行加密处理
ascii_value = ord(char)
encrypted_ascii = (ascii_value + k - b) % 26 # 加上k再减去b
return chr(encrypted_ascii + b) # 添加偏移量b并转换回字母
def decrypt_char(char, k, b):
# 解密过程类似,但是需要先减去偏移量b
decrypted_ascii = (ord(char) - b - k) % 26
return chr(decrypted_ascii)
# 定义加密和解密函数
def affine_cipher(text, key=k, shift=b):
encrypted_text = ""
for char in text:
if char.isalpha(): # 只处理字母字符,忽略其他字符
encrypted_text += encrypt_char(char.lower(), key, shift).upper()
else:
encrypted_text += char # 非字母字符保持不变
return encrypted_text
def inverse_affine_cipher(text, key=k, shift=b):
return ''.join(decrypt_char(char, key, shift) for char in text)
# 示例明文和密钥
plaintext = "Hello, World!"
key = 7
shift = 6
# 加密和解密操作
ciphertext = affine_cipher(plaintext, key, shift)
decrypted_text = inverse_affine_cipher(ciphertext, key, shift)
print(f"原文: {plaintext}")
print(f"加密后: {ciphertext}")
print(f"解密后: {decrypted_text}\n")
阅读全文
相关推荐
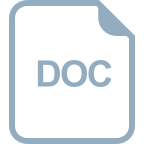
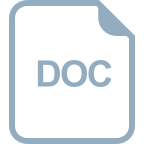
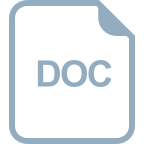



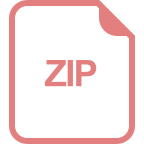
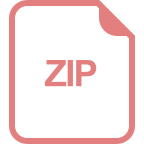
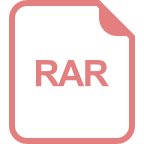
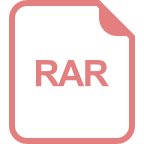
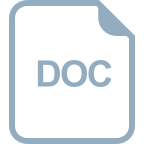




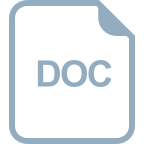
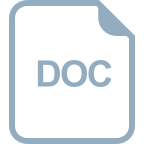