stm32f103zet6按键控制led通过四个按键来控制灯 S1:按下,LED增加一盏灯亮 S2:按下,LED减少一盏灯亮 S3:按下,LED全亮 S4:按下,LED全灭
时间: 2023-07-15 08:15:42 浏览: 120
这个问题可以通过使用GPIO来实现。首先,需要将四个按键和LED连接到相应的GPIO引脚上。
然后,在初始化时,需要将LED的GPIO引脚设置为输出,将四个按键的GPIO引脚设置为输入,并且启用GPIO时钟。
在主循环中,可以使用GPIO_ReadInputDataBit()函数读取四个按键的状态,根据按键的状态来控制LED的亮度。可以使用GPIO_SetBits()和GPIO_ResetBits()函数来设置LED的亮度。
下面是示例代码:
```c
#include "stm32f10x.h"
#define LED_GPIO_PIN GPIO_Pin_5
#define LED_GPIO_PORT GPIOB
#define S1_GPIO_PIN GPIO_Pin_0
#define S1_GPIO_PORT GPIOA
#define S2_GPIO_PIN GPIO_Pin_1
#define S2_GPIO_PORT GPIOA
#define S3_GPIO_PIN GPIO_Pin_2
#define S3_GPIO_PORT GPIOA
#define S4_GPIO_PIN GPIO_Pin_3
#define S4_GPIO_PORT GPIOA
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_GPIO_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = S1_GPIO_PIN | S2_GPIO_PIN | S3_GPIO_PIN | S4_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
int main(void)
{
GPIO_Configuration();
uint8_t led_count = 0;
while (1)
{
if (GPIO_ReadInputDataBit(S1_GPIO_PORT, S1_GPIO_PIN) == RESET)
{
if (led_count < 4) {
led_count++;
}
}
if (GPIO_ReadInputDataBit(S2_GPIO_PORT, S2_GPIO_PIN) == RESET)
{
if (led_count > 0) {
led_count--;
}
}
if (GPIO_ReadInputDataBit(S3_GPIO_PORT, S3_GPIO_PIN) == RESET)
{
led_count = 4;
}
if (GPIO_ReadInputDataBit(S4_GPIO_PORT, S4_GPIO_PIN) == RESET)
{
led_count = 0;
}
for (uint8_t i = 0; i < 4; i++) {
if (i < led_count) {
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN << i);
} else {
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN << i);
}
}
}
}
```
在这个例子中,LED的GPIO引脚为PB5,四个按键的GPIO引脚为PA0-PA3。在主循环中,根据四个按键的状态来控制LED的亮度,led_count变量表示当前亮着的LED数量。当按下S1时,led_count加1,当按下S2时,led_count减1,当按下S3时,led_count设置为4,当按下S4时,led_count设置为0。最后,通过循环来控制LED的亮度。
阅读全文
相关推荐
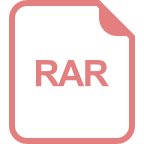
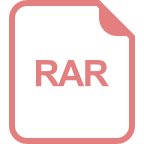
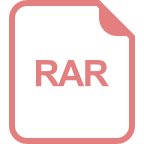
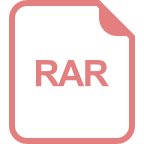
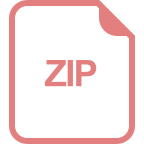
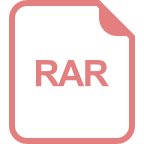
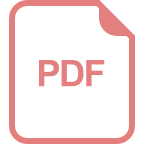
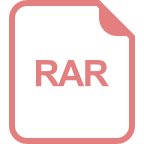
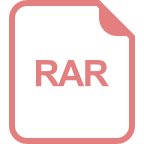
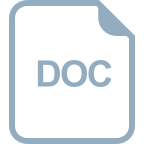
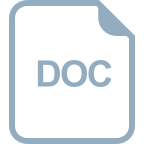
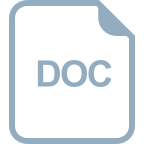
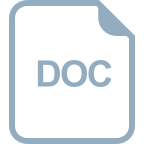
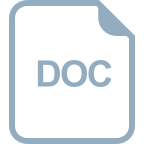
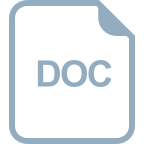
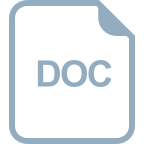