如何用java实现AES加解密
时间: 2024-01-23 16:03:17 浏览: 31
以下是使用Java实现AES加解密的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.Key;
public class AESUtil {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding";
public static byte[] encrypt(String key, String data) throws Exception {
Key secretKey = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data.getBytes(StandardCharsets.UTF_8));
}
public static String decrypt(String key, byte[] encryptedData) throws Exception {
Key secretKey = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedData = cipher.doFinal(encryptedData);
return new String(decryptedData, StandardCharsets.UTF_8);
}
}
```
在上面的代码中,我们使用了Java标准库中的`javax.crypto`包来实现AES加解密。`encrypt`方法接收一个密钥和需要加密的数据,返回加密后的字节数组。`decrypt`方法接收一个密钥和需要解密的字节数组,返回解密后的字符串。
请注意,上面的示例代码中使用了ECB模式和PKCS5Padding填充方式。这两种方式是常见的AES加密方式,但在某些情况下可能不够安全,请根据实际需求选择适合的加密方式。
相关推荐
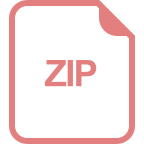














