def generate_samples(m, k): X_normal = 2 * (np.random.rand(m, 1) - 0.5) y_normal = X_normal + np.random.normal(0, 0.1, (m,1)) X_outlier = 2 * (np.random.rand(k, 1) - 0.5) y_outlier = X_outlier + np.random.normal(3, 0.1, (k,1)) X = np.concatenate((X_normal, X_outlier), axis=0) y = np.concatenate((y_normal, y_outlier), axis=0) return X, ynp.random.seed(0) X, y = generate_samples(100, 5)
时间: 2023-06-18 10:06:24 浏览: 48
这段代码定义了一个名为`generate_samples()`的函数,该函数有两个参数:m和k。
函数的主要功能是生成包含异常值的数据集。具体来说,它生成了两个大小分别为m和k的数组X_normal和X_outlier,这些数组包含了在[-1, 1]之间均匀分布的随机数,并将它们乘以2,使它们的范围变为[-2, 2]。然后,它为每个X值生成一个相应的y值,该y值等于X值加上一个从均值为0,标准差为0.1的正态分布中随机抽取的值。这些值被存储在数组y_normal中。
接下来,函数生成了另外的X_outlier和y_outlier数组,以模拟异常值。这些值也是在[-1, 1]之间均匀分布的随机数,但是在计算相应的y值时,它们被加上了从均值为3,标准差为0.1的正态分布中随机抽取的值。这些值被存储在y_outlier数组中。
最后,函数将X_normal和X_outlier数组以及y_normal和y_outlier数组连接起来,生成完整的X和y数组,并将它们作为函数的返回值。
在这里,函数被调用,使用100和5作为参数来生成数据集,并将结果存储在X和y变量中。
相关问题
优化这段import numpy as np import matplotlib.pyplot as plt %config InlineBackend.figure_format='retina' def generate_signal(t_vec, A, phi, noise, freq): Omega = 2*np.pi*freq return A * np.sin(Omega*t_vec + phi) + noise * (2*np.random.random def lock_in_measurement(signal, t_vec, ref_freq): Omega = 2*np.pi*ref_freq ref_0 = 2*np.sin(Omega*t_vec) ref_1 = 2*np.cos(Omega*t_vec) # signal_0 = signal * ref_0 signal_1 = signal * ref_1 # X = np.mean(signal_0) Y = np.mean(signal_1) # A = np.sqrt(X**2+Y**2) phi = np.arctan2(Y,X) print("A=", A, "phi=", phi) # t_vec = np.linspace(0, 0.2, 1001) A = 1 phi = np.pi noise = 0.2 ref_freq = 17.77777 # signal = generate_signal(t_vec, A, phi, noise, ref_freq) # lock_in_measurement(signal, t_vec, ref_freq)
import numpy as np
import matplotlib.pyplot as plt
%config InlineBackend.figure_format='retina'
def generate_signal(t_vec, A, phi, noise, freq):
Omega = 2*np.pi*freq
return A * np.sin(Omega*t_vec + phi) + noise * (2*np.random.random)
def lock_in_measurement(signal, t_vec, ref_freq):
Omega = 2*np.pi*ref_freq
ref_0 = 2*np.sin(Omega*t_vec)
ref_1 = 2*np.cos(Omega*t_vec)
signal_0 = signal * ref_0
signal_1 = signal * ref_1
X = np.mean(signal_0)
Y = np.mean(signal_1)
A = np.sqrt(X**2+Y**2)
phi = np.arctan2(Y,X)
print("A=", A, "phi=", phi)
t_vec = np.linspace(0, 0.2, 1001)
A = 1
phi = np.pi
noise = 0.2
ref_freq = 17.77777
signal = generate_signal(t_vec, A, phi, noise, ref_freq)
lock_in_measurement(signal, t_vec, ref_freq)
优化这段pythonimport numpy as np import matplotlib.pyplot as plt %config InlineBackend.figure_format='retina' #输入信号 def generate_signal(t_vec, A, phi, noise, freq): Omega = 2*np.pi*freq noise = 0.2 return A * np.sin(Omega*t_vec + phi) + noise * (2*np.random.random(t_vec.size)-1) #锁相测量 def lock_in_measurement(signal,t_vec,A,phi,noise,ref_freq): Omega = 2*np.pi*ref_freq ref_0 = 2*np.sin(Omega*t_vec) ref_1 = 2*np.cos(Omega*t_vec) #参量 t_vec = np.linspace(0, 0.2, 1001) A = 1 phi = np.pi ref_freq = 17.77777 #混频 signal_0 = signal * ref_0 signal_1 = signal * ref_1 #滤波 X = np.mean(signal_0) Y = np.mean(signal_1) #计算振幅和相位 A = np.sqrt(X**2+Y**2) phi = np.arctan2(Y,X) print("A=", A, "phi=", phi) # 进行锁相放大测量 lock_in_measurement(signal,t_vec,A,phi,noise,ref_freq)
这段代码中存在一些问题,需要进行优化。首先,函数 `generate_signal` 中的变量 `noise` 在函数内部被重新赋值,这可能导致一些问题。其次,在函数 `lock_in_measurement` 中,参量的赋值应该在函数外部进行,而不是在函数内部进行。最后,在函数 `lock_in_measurement` 中,存在递归调用的问题,需要进行修正。以下是优化后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
%config InlineBackend.figure_format='retina'
def generate_signal(t_vec, A, phi, noise, freq):
Omega = 2*np.pi*freq
return A * np.sin(Omega*t_vec + phi) + noise * (2*np.random.random(t_vec.size)-1)
def lock_in_measurement(signal, t_vec, ref_freq):
Omega = 2*np.pi*ref_freq
ref_0 = 2*np.sin(Omega*t_vec)
ref_1 = 2*np.cos(Omega*t_vec)
# 混频
signal_0 = signal * ref_0
signal_1 = signal * ref_1
# 滤波
X = np.mean(signal_0)
Y = np.mean(signal_1)
# 计算振幅和相位
A = np.sqrt(X**2+Y**2)
phi = np.arctan2(Y,X)
print("A=", A, "phi=", phi)
# 参量
t_vec = np.linspace(0, 0.2, 1001)
A = 1
phi = np.pi
noise = 0.2
ref_freq = 17.77777
# 生成信号
signal = generate_signal(t_vec, A, phi, noise, ref_freq)
# 进行锁相放大测量
lock_in_measurement(signal, t_vec, ref_freq)
```
优化后的代码中,变量 `noise` 的赋值已经移动到了函数外部,函数 `lock_in_measurement` 中的参量赋值也已经移动到了函数外部,递归调用的问题也已经被修正了。此外,函数 `generate_signal` 中的变量 `noise` 已经被正确使用。
相关推荐
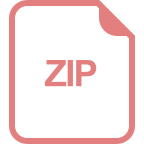













