在Linux上使用C语言编写两个程序,其 中一个程序(进程)创建一个共享内存 区域,并向该区域写入一些内容,另一 个程序(进程)从该共享区域读出内容 ,验证写入的内容与读出的内容是否一 致
时间: 2024-05-14 12:18:48 浏览: 150
下面是一个简单的示例程序,其中一个进程创建共享内存,另一个进程读取共享内存并验证写入的内容是否一致:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define SHM_SIZE 1024
int main()
{
int shmid;
char *shmaddr;
char *str = "Hello, World!";
// 创建共享内存
shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT | 0666);
if (shmid < 0) {
perror("shmget");
exit(1);
}
// 附加共享内存
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *)-1) {
perror("shmat");
exit(1);
}
// 写入数据到共享内存
strcpy(shmaddr, str);
// 创建子进程
pid_t pid = fork();
if (pid == -1) {
perror("fork");
exit(1);
} else if (pid == 0) {
// 子进程读取共享内存数据
char buf[SHM_SIZE];
memcpy(buf, shmaddr, SHM_SIZE);
printf("Read from shared memory: %s\n", buf);
exit(0);
} else {
// 等待子进程结束
wait(NULL);
}
// 解除共享内存附加
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(1);
}
// 删除共享内存
if (shmctl(shmid, IPC_RMID, 0) == -1) {
perror("shmctl");
exit(1);
}
return 0;
}
```
在这个示例程序中,我们使用`shmget`函数创建了一个大小为1024字节的共享内存段,并使用`shmat`函数将其附加到当前进程的地址空间中。然后,我们将字符串"Hello, World!"写入该共享内存段中。接着,我们创建一个子进程,并在子进程中使用`memcpy`函数将共享内存中的数据读取到缓冲区中,并打印出来。
最后,我们使用`shmdt`函数解除共享内存的附加,并使用`shmctl`函数删除该共享内存段。
阅读全文
相关推荐
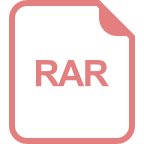
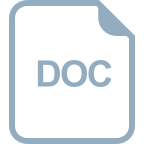
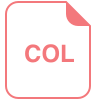
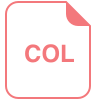
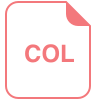
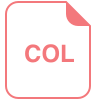
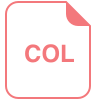
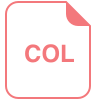
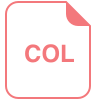
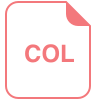
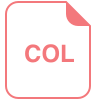
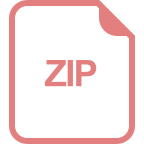