php声明一个人类,成员属性有姓名、年龄、性别、身高等,成员方法有说话、走路等。声明第一个子类司机类继承人类,成员属性有驾龄,成员方法有开车;声明第二个子类医生类继承人类,成员属性有所在医院、专业,成员方法有治病。还可以自行设计其他子类。
时间: 2023-10-20 17:15:17 浏览: 60
好的,以下是 PHP 代码实现:
```php
class Person {
protected $name;
protected $age;
protected $gender;
protected $height;
public function __construct($name, $age, $gender, $height) {
$this->name = $name;
$this->age = $age;
$this->gender = $gender;
$this->height = $height;
}
public function say() {
echo "Hello, my name is " . $this->name . ".\n";
}
public function walk() {
echo $this->name . " is walking.\n";
}
}
class Driver extends Person {
protected $drivingYears;
public function __construct($name, $age, $gender, $height, $drivingYears) {
parent::__construct($name, $age, $gender, $height);
$this->drivingYears = $drivingYears;
}
public function drive() {
echo $this->name . " is driving for " . $this->drivingYears . " years.\n";
}
}
class Doctor extends Person {
protected $hospital;
protected $specialty;
public function __construct($name, $age, $gender, $height, $hospital, $specialty) {
parent::__construct($name, $age, $gender, $height);
$this->hospital = $hospital;
$this->specialty = $specialty;
}
public function cure() {
echo $this->name . " is curing patients in " . $this->hospital . ".\n";
}
}
// 测试代码
$person = new Person("Tom", 25, "male", 180);
$person->say();
$person->walk();
$driver = new Driver("Mike", 30, "male", 175, 10);
$driver->say();
$driver->walk();
$driver->drive();
$doctor = new Doctor("Lily", 35, "female", 165, "ABC Hospital", "Cardiology");
$doctor->say();
$doctor->walk();
$doctor->cure();
```
以上代码中,`Person` 类是一个基类,定义了人的基本属性和行为,`Driver` 类继承自 `Person` 类,新增了驾龄属性和开车行为,`Doctor` 类同样继承自 `Person` 类,新增了所在医院和专业属性,以及治病行为。通过多态的方式,我们可以根据需要实例化不同的对象,来调用不同的方法。
相关推荐
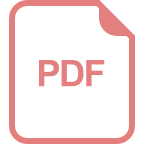














