根据 excel 其中一列邮箱地址.将对应内容邮件给对应人的 python 代码
时间: 2023-08-31 22:29:36 浏览: 98
### 回答1:
以下是一个示例代码,可以根据Excel中的一列邮箱地址发送邮件:
```python
import pandas as pd
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
# 登录SMTP服务器
smtp_server = 'smtp.example.com' # 请替换为您的SMTP服务器地址
smtp_port = 587 # 请替换为您的SMTP服务器端口号
smtp_username = 'your_username' # 请替换为您的SMTP服务器用户名
smtp_password = 'your_password' # 请替换为您的SMTP服务器密码
smtp_sender = 'your_sender_email@example.com' # 请替换为您的发件人邮箱地址
smtp_conn = smtplib.SMTP(smtp_server, smtp_port)
smtp_conn.starttls()
smtp_conn.login(smtp_username, smtp_password)
# 读取Excel文件
excel_file_path = 'path/to/your/excel/file.xlsx' # 请替换为您的Excel文件路径
df = pd.read_excel(excel_file_path)
# 发送邮件
for index, row in df.iterrows():
recipient_email = row['Email'] # 请替换为您的Excel文件中的邮箱地址列名称
subject = '邮件主题' # 请替换为您的邮件主题
body = '邮件内容' # 请替换为您的邮件内容
msg = MIMEMultipart()
msg['Subject'] = subject
msg['From'] = smtp_sender
msg['To'] = recipient_email
text = MIMEText(body)
msg.attach(text)
# 请替换为您需要发送的附件
with open('path/to/your/attachment.pdf', 'rb') as f:
attachment = MIMEApplication(f.read(), _subtype='pdf')
attachment.add_header('Content-Disposition', 'attachment', filename='attachment.pdf')
msg.attach(attachment)
smtp_conn.sendmail(smtp_sender, recipient_email, msg.as_string())
smtp_conn.quit()
print('邮件发送完成')
```
注意:在使用此代码之前,请确保您已经安装了 pandas 和 openpyxl 库。此外,还需要提供SMTP服务器的详细信息,以及Excel文件的路径和电子邮件内容。
### 回答2:
可以使用Python的smtplib和email库来发送电子邮件。以下是一个示例代码:
```python
import smtplib
from email.mime.text import MIMEText
from email.header import Header
import pandas as pd
# 读取Excel文件
df = pd.read_excel('data.xlsx')
# 邮件服务器设置
smtp_server = '邮件服务器地址'
smtp_port = 25
# 发件人信息
sender = '发件人邮箱'
password = '发件人邮箱密码'
# 循环发送邮件
for index, row in df.iterrows():
receiver = row['邮箱地址'] # 表格中的邮箱地址列名称
content = row['邮件内容'] # 表格中的邮件内容列名称
# 创建邮件内容
message = MIMEText(content, 'plain', 'utf-8')
message['From'] = Header(sender, 'utf-8')
message['To'] = Header(receiver, 'utf-8')
message['Subject'] = Header('邮件主题', 'utf-8')
try:
# 连接邮件服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.login(sender, password)
# 发送邮件
server.sendmail(sender, receiver, message.as_string())
except Exception as e:
print(f"邮件发送失败:{e}")
finally:
# 关闭连接
server.quit()
```
这段代码首先使用pandas库来读取Excel文件中的邮箱地址和邮件内容列。然后,根据设置好的邮件服务器信息,通过循环遍历每一行数据,创建邮件内容并使用smtplib库连接邮件服务器,登录发件人邮箱,并发送邮件给对应的收件人。最后,关闭连接。
### 回答3:
以下是一个使用Python发送邮件的代码示例:
```python
import smtplib
from email.mime.text import MIMEText
from email.header import Header
# 邮件服务器配置
mail_host = "smtp.example.com" # 邮件服务器地址
mail_port = 25 # 邮件服务器端口
mail_user = "your_username" # 发件人邮箱用户名
mail_password = "your_password" # 发件人邮箱密码
# 导入邮箱地址数据
emails = [
"recipient1@example.com",
"recipient2@example.com",
"recipient3@example.com",
# ... 其他邮箱地址
]
# 发送邮件
for email in emails:
# 构造邮件内容
message = MIMEText("这是邮件内容", "plain", "utf-8")
message["From"] = Header(mail_user, "utf-8") # 发件人
message["To"] = Header(email, "utf-8") # 收件人
message["Subject"] = Header("这是邮件主题", "utf-8") # 邮件主题
try:
# 链接邮件服务器
smtpObj = smtplib.SMTP(mail_host, mail_port)
smtpObj.login(mail_user, mail_password)
# 发送邮件
smtpObj.sendmail(mail_user, email, message.as_string())
print("邮件发送成功")
except smtplib.SMTPException as e:
print("邮件发送失败:", str(e))
finally:
# 关闭邮件服务器链接
smtpObj.quit()
```
请根据实际情况修改邮件服务器配置(`mail_host`、`mail_port`、`mail_user`、`mail_password`)和邮件内容(`MIMEText`)。
注意:在使用此代码之前,请确保已经安装了所需的库:`smtplib`、`email`。
阅读全文
相关推荐
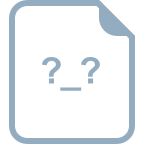




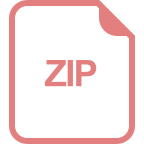
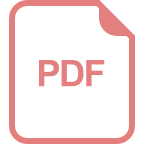
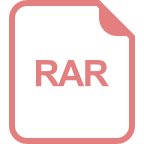
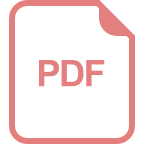
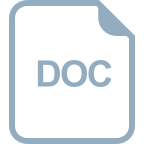
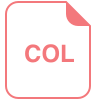
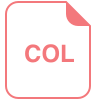
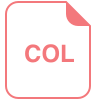
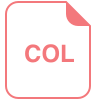
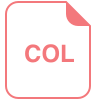
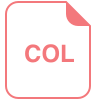
