根据 excel 其中一列邮箱地址.将对应内容以 excel 为附件邮件给对应人的 python 代码
时间: 2023-06-20 15:04:10 浏览: 213
以下是一个基于 Python 的示例代码,用于从 Excel 文件中读取电子邮件地址和内容,并将内容作为附件发送给相应的电子邮件地址:
```python
import pandas as pd
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
# 读取 Excel 文件中的电子邮件地址和内容
df = pd.read_excel("file.xlsx", sheet_name="Sheet1")
# SMTP 服务器设置
smtp_server = "smtp.example.com"
smtp_port = 587
smtp_username = "your_username"
smtp_password = "your_password"
# 登录 SMTP 服务器
smtp = smtplib.SMTP(smtp_server, smtp_port)
smtp.starttls()
smtp.login(smtp_username, smtp_password)
# 循环遍历每个电子邮件地址并发送邮件
for index, row in df.iterrows():
to_email = row["Email"]
subject = "Your subject here"
body = row["Content"]
# 构建邮件主体
message = MIMEMultipart()
text = MIMEText(body)
message.attach(text)
# 添加附件
with open("attachment_file", "rb") as f:
attach = MIMEApplication(f.read(), _subtype="pdf")
attach.add_header("Content-Disposition", "attachment", filename="attachment_filename")
message.attach(attach)
message["Subject"] = subject
message["From"] = smtp_username
message["To"] = to_email
# 发送邮件
smtp.sendmail(smtp_username, to_email, message.as_string())
# 关闭 SMTP 连接
smtp.quit()
```
请注意,您需要将代码中的以下值替换为适当的值:
- `file.xlsx`:包含电子邮件地址和内容的 Excel 文件名。
- `Sheet1`:包含电子邮件地址和内容的工作表名称。
- `smtp_server`:您的 SMTP 服务器的主机名或 IP 地址。
- `smtp_port`:您的 SMTP 服务器的端口号。
- `smtp_username`:您的 SMTP 服务器的用户名。
- `smtp_password`:您的 SMTP 服务器的密码。
- `attachment_file`:要附加到电子邮件的文件路径和名称。
- `attachment_filename`:要附加到电子邮件的文件名称。
阅读全文
相关推荐
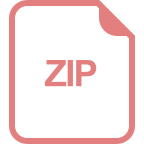
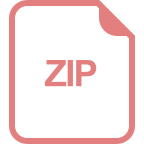




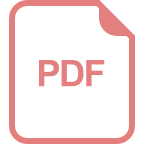
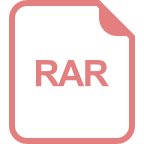
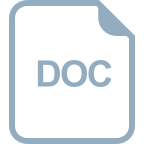
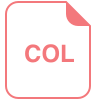
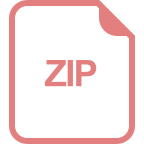