用js的方法marker是一个数组,data也是一个数组,用data里面lat的值替换marker里面latitude的值
时间: 2024-02-06 17:09:43 浏览: 183
假设marker数组中的每个元素都包含一个名为latitude的属性,表示标记的纬度,data数组中的每个元素也都包含一个名为lat的属性,表示该位置的纬度。可以通过循环遍历两个数组来实现这个替换的过程。具体的代码如下:
```javascript
for (var i = 0; i < marker.length; i++) {
marker[i].latitude = data[i].lat;
}
```
这段代码会遍历marker数组中的所有标记,并将每个标记的latitude属性替换为data数组中对应位置的lat属性。需要注意的是,这段代码假设marker数组和data数组的长度相同,如果两个数组的长度不同,则需要在循环中进行额外的判断和处理。
相关问题
@app.route('/get_trip_time', methods=['POST']) def get_trip_time(): data = request.get_json() method = data['method'] center_coor = data['center_coor'] t = data['t'] radius = get_radius(method, t) gtt = GetTripTime(method, center_coor, t, radius) gtt.main() return jsonify({'message': 'Trip time data collected successfully'}) @app.route('/visualize_trip_time', methods=['GET']) def visualize_trip_time(): data = pd.read_csv('time1.csv') lng = data['lng'] lat = data['lat'] time = data['time'] grid_lng, grid_lat = np.meshgrid(np.linspace(lng.min(), lng.max(), 100), np.linspace(lat.min(), lat.max(), 100)) grid_time = griddata((lng, lat), time, (grid_lng, grid_lat), method='linear') fig, ax = plt.subplots(figsize=(8, 8)) contour_plot = ax.contourf(grid_lng, grid_lat, grid_time, cmap='jet', levels=6) ax.contour(contour_plot, colors='k', linewidths=0.5) plt.colorbar(contour_plot) last_lng = lng.iloc[-1] last_lat = lat.iloc[-1] ax.scatter(last_lng, last_lat, color='green', marker='o', s=50, label='Start Point') ax.legend() plt.title('Isochrone') ax.set_xlabel('Longitude') ax.set_ylabel('Latitude') ax.xaxis.set_major_formatter(mticker.FormatStrFormatter('%.2f')) plt.show() return jsonify({'message': 'Trip time visualization generated successfully'}) @app.route('/get_isochrone_coords', methods=['GET']) def get_isochrone_coords(): with open('contour_coords.json', 'r') as f: contour_coords = json.load(f) return jsonify(contour_coords)用rest client调用GET http://localhost:5000/visualize_trip_time时报错ValueError: signal only works in main thread of the main interpreter
这个错误是由于在非主线程中调用了Matplotlib的绘图函数导致的。解决这个问题的方法是使用Matplotlib的线程安全设置。
您可以尝试在应用程序的入口处添加以下代码,以确保在非主线程中使用Matplotlib时不会出现此错误:
```python
import matplotlib
matplotlib.use('Agg')
```
这将将Matplotlib的后端设置为“Agg”,它不依赖于任何GUI库,并且线程安全。
如果上述解决方案无效,您还可以尝试将绘图的相关代码放在一个函数中,并使用`matplotlib.pyplot.switch_backend('Agg')`在函数内部设置后端,例如:
```python
import matplotlib.pyplot as plt
def visualize_trip_time():
# 设置Matplotlib后端为Agg
plt.switch_backend('Agg')
# 绘图代码...
# ...
# ...
plt.savefig('trip_time.png')
```
这将确保在非主线程中调用绘图函数时不会出现错误,并将图像保存到文件中。
请注意,在使用`matplotlib.use('Agg')`或`matplotlib.pyplot.switch_backend('Agg')`之后,您将无法在应用程序中直接显示图像。如果需要显示图像,您可以保存图像到文件,并通过其他方式访问该文件。
希望这些解决方案能帮助您解决问题。如果问题仍然存在,请提供更多代码和错误信息,以便我们能够更好地帮助您。
<map longitude="{{longitude}}" latitude="{{latitude}}" markers="{{markers}}"satellite="true" scale="15"> <!--在地图上添加一个搜索框并实现定位功能--> <form class="search-form"> <input type="text" placeholder="请输入位置名称以搜索" bindchange="onSearchInputChange" /> </form> <!-- 添加圆形定位按钮 --> <view class="map-button"> <image class="map-button-image" src="/images/map-button.png" /> </view> </map>Page({ /** * 页面的初始数据 */ data: { //经纬度 latitude: 45.745842, longitude: 127.212255, markers: [{ latitude: 45.745842, longitude: 127.212255, width: 35, height: 50 }] }, onLoad: function() { var that = this; wx.getLocation({ type: 'gcj02', success: function(res) { that.setData({ latitude: res.latitude, longitude: res.longitude, }); } }); }, onSearchInputChange: function(event) { var that = this; var searchValue = event.detail.value; if (searchValue) { wx.getLocation({ type: 'gcj02', success: function(res) { var latitude = res.latitude; var longitude = res.longitude; var marker = { id: 0, latitude: latitude, longitude: longitude, width: 50, height: 50, }; this.setData({ longitude: longitude, latitude: latitude, markers: [marker] }); } }); } } })实现代码中的搜索和定位功能
可以使用腾讯地图的 JavaScript API 实现搜索和定位功能。具体实现步骤如下:
1. 在页面中引入腾讯地图 JavaScript API:
```
<script src="https://map.qq.com/api/js?v=2.exp&key=YOUR_KEY"></script>
```
其中,YOUR_KEY 是申请腾讯地图 API 时获取到的密钥。
2. 在页面中添加地图组件,以及搜索框和定位按钮:
```
<map id="map" longitude="{{longitude}}" latitude="{{latitude}}" markers="{{markers}}" scale="{{scale}}" show-location>
<form class="search-form">
<input type="text" placeholder="请输入位置名称以搜索" bindconfirm="onSearchConfirm" />
</form>
<view class="map-button" bindtap="onLocationTap">
<image class="map-button-image" src="/images/map-button.png" />
</view>
</map>
```
其中,id 属性为地图组件的标识符,longitude 和 latitude 属性为地图的经纬度,markers 属性为地图上的标记点,scale 属性为地图的缩放级别,show-location 属性为是否显示当前位置。
3. 在页面的 onLoad 函数中获取当前位置,并更新地图的经纬度:
```
onLoad: function() {
var that = this;
wx.getLocation({
type: 'gcj02',
success: function(res) {
that.setData({
latitude: res.latitude,
longitude: res.longitude,
markers: [{
id: 0,
latitude: res.latitude,
longitude: res.longitude,
iconPath: '/images/location.png',
width: 20,
height: 20
}]
});
}
});
},
```
其中,markers 属性为一个数组,用于在地图上显示标记点。这里只显示一个当前位置的标记点,使用了一个 location.png 图标。
4. 在页面的 onSearchConfirm 函数中根据关键字搜索地点,并更新地图的经纬度和标记点:
```
onSearchConfirm: function(event) {
var that = this;
var keyword = event.detail.value;
var map = new qq.maps.Map(document.getElementById('map'));
var searchService = new qq.maps.SearchService({
complete: function(results) {
if (results.type === 'no_data') {
wx.showToast({
title: '未找到相关位置',
icon: 'none'
});
} else {
var location = results.detail.location;
that.setData({
latitude: location.lat,
longitude: location.lng,
markers: [{
id: 0,
latitude: location.lat,
longitude: location.lng,
iconPath: '/images/location.png',
width: 20,
height: 20
}]
});
}
},
error: function() {
wx.showToast({
title: '搜索失败,请重试',
icon: 'none'
});
}
});
searchService.search(keyword);
},
```
其中,qq.maps.Map 函数用于创建一个地图对象,qq.maps.SearchService 函数用于创建一个搜索服务对象。complete 回调函数在搜索成功时被调用,error 回调函数在搜索失败时被调用。如果搜索成功,从搜索结果中获取到地点的经纬度,并更新地图的经纬度和标记点。
5. 在页面的 onLocationTap 函数中获取当前位置,并更新地图的经纬度和标记点:
```
onLocationTap: function() {
var that = this;
wx.getLocation({
type: 'gcj02',
success: function(res) {
that.setData({
latitude: res.latitude,
longitude: res.longitude,
markers: [{
id: 0,
latitude: res.latitude,
longitude: res.longitude,
iconPath: '/images/location.png',
width: 20,
height: 20
}]
});
}
});
},
```
这里与 onLoad 函数中的代码类似,只是在点击按钮时触发获取当前位置的操作。
以上就是搜索和定位功能的实现代码。需要注意的是,这里使用的是腾讯地图 JavaScript API,而非微信小程序自带的地图组件。因此需要引入腾讯地图 JavaScript API,并在代码中使用相关的对象和方法。
阅读全文
相关推荐
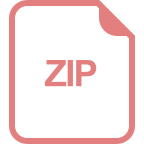
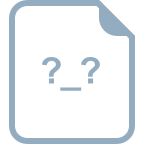
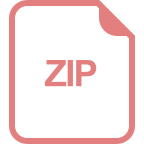


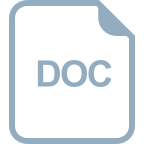
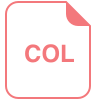
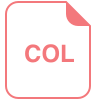
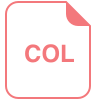
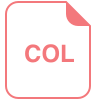






