c语言调用shell命令
时间: 2023-06-05 21:47:56 浏览: 319
在C语言中,可以使用system()函数来调用shell命令。该函数的原型如下:
int system(const char *command);
其中,command参数是要执行的shell命令字符串。例如,要在C语言中执行ls命令,可以使用以下代码:
system("ls");
该代码会在终端中执行ls命令,并将结果输出到终端。注意,system()函数的返回值是执行命令的返回值,如果命令执行成功,返回值为,否则返回非零值。
相关问题
c语言调用shell
C语言可以通过system函数调用shell命令。system函数可以执行一个shell命令,并等待命令执行完毕后返回。例如,下面的代码可以在Linux系统中执行ls命令:
#include <stdlib.h>
int main()
{
system("ls");
return 0;
}
在Windows系统中,可以使用system函数调用cmd命令。例如,下面的代码可以在Windows系统中执行dir命令:
#include <stdlib.h>
int main()
{
system("cmd /c dir");
return 0;
}
需要注意的是,system函数调用的命令会在一个新的shell进程中执行,因此可能会对程序的性能产生影响。同时,由于system函数调用的命令可以是任意的shell命令,因此也存在一定的安全风险。因此,在使用system函数时需要谨慎处理。
c语言调用shell脚本
在C语言中,可以使用system()、popen()和exec系列函数来调用shell脚本。
1. 使用system()函数调用shell脚本:
```c
#include <stdlib.h>
int main() {
system("sh script.sh"); // 调用名为script.sh的shell脚本
return 0;
}
```
2. 使用popen()函数调用shell脚本并获取输出结果:
```c
#include <stdio.h>
int main() {
FILE *fp;
char buffer[1024];
fp = popen("sh script.sh", "r"); // 调用名为script.sh的shell脚本,并以只读方式打开管道
if (fp == NULL) {
printf("Failed to run command\n");
return 1;
}
while (fgets(buffer, sizeof(buffer), fp) != NULL) {
printf("%s", buffer); // 输出shell脚本的输出结果
}
pclose(fp); // 关闭管道
return 0;
}
```
3. 使用exec系列函数调用shell脚本:
```c
#include <unistd.h>
int main() {
execl("/bin/sh", "sh", "script.sh", (char *)0); // 调用名为script.sh的shell脚本
return 0;
}
```
相关推荐
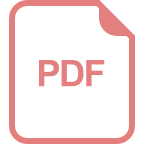
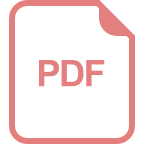
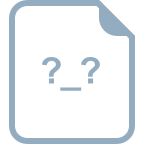
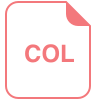
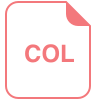










