typedef struct student 数据结构用尾插法建链输出姓名学号成绩
时间: 2024-10-18 10:22:06 浏览: 29
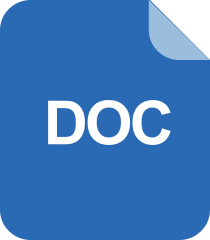
成绩统计系统数据结构课程设计

typedef struct student {
char name[20]; // 学生姓名
int id; // 学号
float score; // 成绩
struct student* next; // 指向下一个学生的指针(用于链表)
} Student;
如果你想用尾插法(也称为“单链表的尾部插入”)来构建这种数据结构并输出学生信息,你可以按照以下步骤操作:
1. 首先,创建一个Student类型的头节点,并设置其next指针为NULL。
```c
Student* head = (Student*)malloc(sizeof(Student));
head->name[0] = '\0'; // 初始化空字符串作为默认值
head->id = 0;
head->score = 0;
head->next = NULL;
```
2. 然后,每当有新学生的信息需要添加到链表中,就创建一个新的Student结构体,并将其插入到现有链表的末尾。
```c
void addStudent(Student** head, const char* name, int id, float score) {
Student* newStudent = (Student*)malloc(sizeof(Student));
strcpy(newStudent->name, name);
newStudent->id = id;
newStudent->score = score;
newStudent->next = NULL;
if (*head == NULL) { // 如果链表为空,直接将新学生设为头结点
*head = newStudent;
} else {
Student* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newStudent;
}
}
```
3. 最后,遍历链表并打印每个学生的信息:
```c
void printStudents(Student* head) {
if (head == NULL) {
printf("链表为空\n");
} else {
Student* current = head;
while (current != NULL) {
printf("姓名:%s, 学号:%d, 成绩:%f\n", current->name, current->id, current->score);
current = current->next;
}
}
}
```
阅读全文
相关推荐
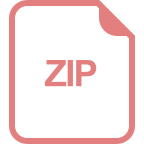
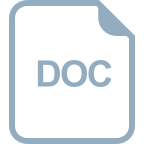
















