用尾插法建链输出学生,姓名,学号,成绩
时间: 2024-10-18 21:22:09 浏览: 30
尾插法是指在链表的最后插入元素的方式。为了用尾插法创建一个存储学生信息(包括姓名、学号和成绩)的链表并输出,你需要首先定义一个`Student`结构体,然后编写函数来创建节点和遍历链表。以下是基本步骤:
1. 定义`Student`结构体:
```c
typedef struct {
char name[50]; // 姓名,假设最大长度为50字符
int id; // 学号
float score; // 成绩
struct Student* next; // 指向下一个学生的指针
} Student;
```
2. 创建一个空链表的头节点:
```c
Student* head = NULL;
```
3. 添加学生信息到链表(尾插法):
```c
void add_student(Student** head, char* name, int id, float score) {
Student* new_student = (Student*)malloc(sizeof(Student));
strcpy(new_student->name, name);
new_student->id = id;
new_student->score = score;
new_student->next = NULL;
if (*head == NULL) {
*head = new_student;
} else {
Student* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = new_student;
}
}
```
4. 输出链表中的所有学生信息:
```c
void print_students(Student* head) {
if (head == NULL) {
printf("链表为空\n");
} else {
Student* temp = head;
while (temp != NULL) {
printf("姓名:%s, 学号:%d, 成绩:%f\n", temp->name, temp->id, temp->score);
temp = temp->next;
}
}
}
```
你可以通过调用`add_student`函数添加学生,然后调用`print_students`函数来显示链表中的信息。记得处理好内存分配和释放,防止内存泄漏。
阅读全文
相关推荐
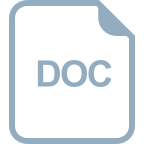
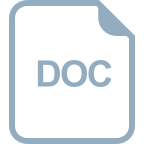
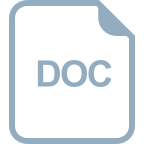




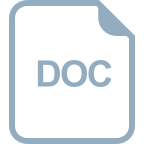
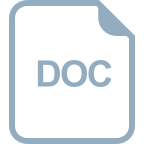
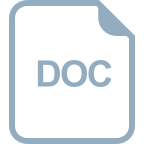
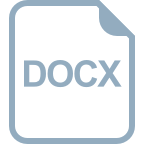
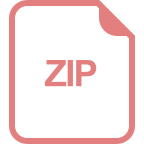
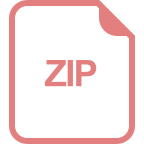
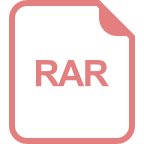
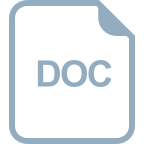
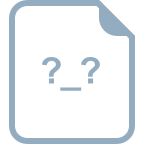
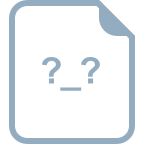
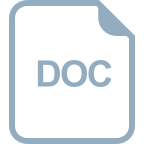
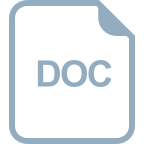