c++中怎样传入回调函数作为参数,写出例子
时间: 2024-09-10 10:22:40 浏览: 39
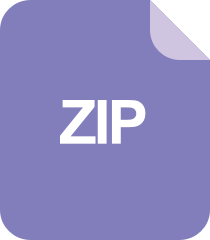
帮助理解回调函数的简洁实例
在C++中,可以使用函数指针、lambda表达式、std::function或boost库中的function对象来传递回调函数作为参数。这里给出几个例子:
1. 使用函数指针:
```cpp
#include <iostream>
void callback(int value) {
std::cout << "Callback received: " << value << std::endl;
}
void pass_callback(void (*cb)(int)) {
cb(42); // 调用传入的回调函数
}
int main() {
pass_callback(callback); // 将callback函数指针传递给pass_callback
return 0;
}
```
2. 使用lambda表达式:
```cpp
#include <iostream>
void call_back_lambda(int value) {
std::cout << "Lambda callback received: " << value << std::endl;
}
void pass_lambda(auto lambda) {
lambda(24); // 调用lambda函数
}
int main() {
auto my_lambda = [value]() mutable { call_back_lambda(value); };
pass_lambda(my_lambda);
return 0;
}
```
3. 使用std::function:
```cpp
#include <iostream>
#include <functional>
void std_callback(int value) {
std::cout << "std::function callback received: " << value << std::endl;
}
void pass_std_function(const std::function<void(int)> &func) {
func(64); // 调用std::function对象
}
int main() {
std::function<void(int)> my_func = std_callback;
pass_std_function(my_func);
return 0;
}
```
以上三种方法都可以将函数作为参数传递给其他函数,在适当的时候执行。
阅读全文
相关推荐
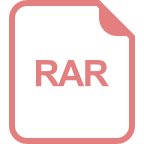
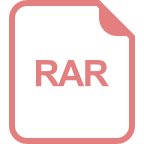

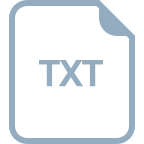
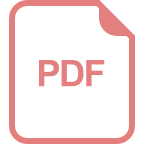
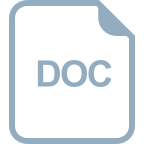
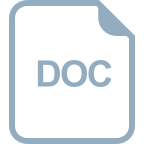
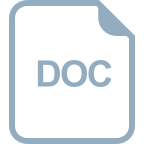
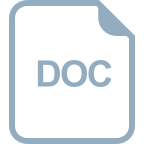
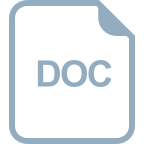
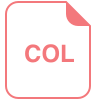
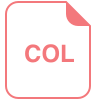
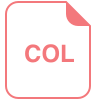
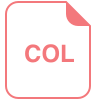
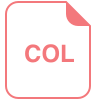
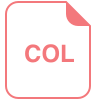

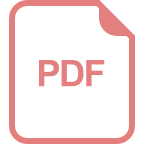