json_file = open(json_path, "r") class_indict = json.load(json_file)
时间: 2024-04-01 07:31:03 浏览: 21
这段代码首先使用Python内置的`open`函数打开了一个文件对象`json_file`,该文件对象表示了`class_indices.json`文件。打开文件时,使用了`"r"`参数,表示以只读模式打开文件。
然后,代码使用`json.load`函数将`json_file`中的内容加载为一个Python字典对象`class_indict`。该字典表示了类别名称到类别索引的映射关系,即字典的键为类别名称,值为类别索引。这个字典通常用于将模型的输出转换为类别名称,或者将类别名称转换为类别索引。
需要注意的是,`class_indices.json`文件的格式必须符合JSON规范,否则`json.load`函数会抛出异常。如果无法确定文件是否符合JSON规范,可以使用`json.loads`函数将文件内容加载为Python对象,如果加载成功,则说明文件符合JSON规范。
相关问题
为以下每句代码做注释:device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") data_transform = transforms.Compose( [transforms.Resize(256), transforms.CenterCrop(224), transforms.ToTensor(), transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]) img = Image.open("./huanglongbing.JPG") plt.imshow(img) img = data_transform(img) img = torch.unsqueeze(img, dim=0) try: json_file = open('./class_indices.json', 'r') class_indict = json.load(json_file) except Exception as e: print(e) exit(-1) model = resnet152(num_classes=38) model_weight_path = "./resNet152.pth" model.load_state_dict(torch.load(model_weight_path, map_location=device)) model.eval() with torch.no_grad(): output = torch.squeeze(model(img)) predict = torch.softmax(output, dim=0) predict_cla = torch.argmax(predict).numpy() print(class_indict[str(predict_cla)], predict[predict_cla].numpy()) plt.show()
# 设备选择:如果有可用的cuda设备,则使用cuda:0,否则使用cpu
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
# 数据变换操作,包括图像大小调整、中心裁剪、转换为张量、归一化等
data_transform = transforms.Compose(
[transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]
)
# 打开图像文件,显示图像
img = Image.open("./huanglongbing.JPG")
plt.imshow(img)
# 对图像进行数据变换
img = data_transform(img)
img = torch.unsqueeze(img, dim=0)
# 读取类别标签与索引的对应关系
try:
json_file = open('./class_indices.json', 'r')
class_indict = json.load(json_file)
except Exception as e:
print(e)
exit(-1)
# 加载预训练的resnet152模型,并载入预训练权重
model = resnet152(num_classes=38)
model_weight_path = "./resNet152.pth"
model.load_state_dict(torch.load(model_weight_path, map_location=device))
model.eval()
# 使用载入的模型进行推理,并输出预测结果
with torch.no_grad():
output = torch.squeeze(model(img))
predict = torch.softmax(output, dim=0)
predict_cla = torch.argmax(predict).numpy()
print(class_indict[str(predict_cla)], predict[predict_cla].numpy())
plt.show()
为以下每句代码做注释:import torch from model import resnet152 from PIL import Image from torchvision import transforms import matplotlib.pyplot as plt import json device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") data_transform = transforms.Compose( [transforms.Resize(256), transforms.CenterCrop(224), transforms.ToTensor(), transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]) img = Image.open("./huanglongbing.JPG") plt.imshow(img) img = data_transform(img) img = torch.unsqueeze(img, dim=0) try: json_file = open('./class_indices.json', 'r') class_indict = json.load(json_file) except Exception as e: print(e) exit(-1) model = resnet152(num_classes=38) model_weight_path = "./resNet152.pth" model.load_state_dict(torch.load(model_weight_path, map_location=device)) model.eval() with torch.no_grad(): output = torch.squeeze(model(img)) predict = torch.softmax(output, dim=0) predict_cla = torch.argmax(predict).numpy() print(class_indict[str(predict_cla)], predict[predict_cla].numpy()) plt.show()
# 导入所需的库
import torch
from model import resnet152
from PIL import Image
from torchvision import transforms
import matplotlib.pyplot as plt
import json
# 判断是否有GPU可用,若有则使用GPU,否则使用CPU
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
# 定义数据预处理的步骤,包括图片的resize、中心裁剪、转换为张量、以及标准化
data_transform = transforms.Compose(
[transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]
)
# 打开图片并显示
img = Image.open("./huanglongbing.JPG")
plt.imshow(img)
# 对图片进行预处理,并增加一维作为batch_size
img = data_transform(img)
img = torch.unsqueeze(img, dim=0)
# 读取class_indices.json文件,获取类别标签
try:
json_file = open('./class_indices.json', 'r')
class_indict = json.load(json_file)
except Exception as e:
print(e)
exit(-1)
# 加载预训练好的模型,以及其对应的权重文件
model = resnet152(num_classes=38)
model_weight_path = "./resNet152.pth"
model.load_state_dict(torch.load(model_weight_path, map_location=device))
model.eval()
# 在不进行梯度计算的情况下,使用模型进行预测
with torch.no_grad():
output = torch.squeeze(model(img))
predict = torch.softmax(output, dim=0) # 对输出进行softmax处理
predict_cla = torch.argmax(predict).numpy() # 获取预测的类别
# 输出预测结果以及对应的概率,并显示图片
print(class_indict[str(predict_cla)], predict[predict_cla].numpy())
plt.show()
相关推荐
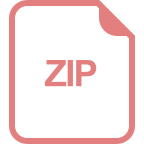
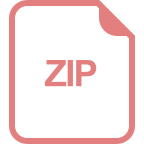










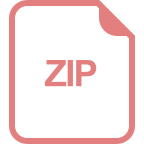
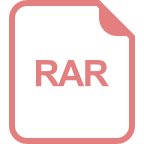