【JSON数据处理最佳实践】:Python处理复杂JSON结构的专家指南
发布时间: 2024-09-12 05:45:09 阅读量: 75 订阅数: 23 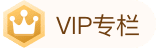
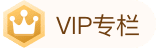
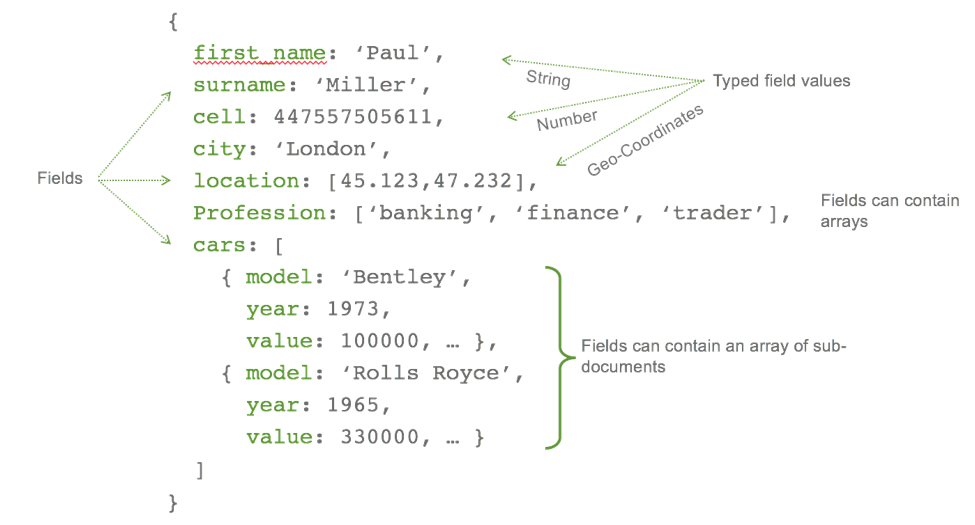
# 1. JSON数据处理基础知识
在现代信息技术领域,JSON(JavaScript Object Notation)已成为轻量级的数据交换格式,广泛应用于网络通信、数据存储和配置文件等场景。对于IT行业从业者来说,理解和掌握JSON数据处理的基本概念和方法是必须的。
JSON数据处理不仅包括对数据格式的熟悉,还包括能够使用各种编程语言实现数据的序列化和反序列化。序列化是指将数据结构或对象状态转换为可存储或传输的格式(例如字符串),而反序列化则是将这些格式重新转换回原始的数据结构。
JSON数据处理流程通常涉及以下几个基本步骤:
- **创建JSON数据**:确定需要表达的数据内容,并按照JSON格式规范组织数据。
- **序列化与反序列化**:将数据结构转换为JSON字符串,以及将JSON字符串解析回数据结构。
- **数据传输与存储**:将JSON数据进行网络传输或存储到文件系统中。
- **数据验证**:确保JSON数据格式正确,且符合预期的数据结构。
在本文中,我们将深入探讨JSON数据处理的每一个环节,同时提供具体的代码示例和实际应用场景分析,帮助读者建立一个系统化的JSON处理框架。接下来的章节将着重介绍JSON数据的结构、Python中处理JSON的方法、复杂JSON结构的处理技巧、性能优化、数据安全以及综合案例分析。
# 2. Python中的JSON库详解
## 2.1 JSON数据格式解析
### 2.1.1 JSON数据结构简介
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人阅读和编写,同时也易于机器解析和生成。它基于JavaScript的一个子集,但JSON是独立于语言的,很多编程语言都能很好地支持JSON格式数据的处理。JSON数据结构主要包括以下几种:
- **对象**:由键值对组成,使用大括号 `{}` 包围。
- **数组**:元素序列,使用方括号 `[]` 包围。
- **值**:可以是字符串(用双引号表示)、数值、布尔值、null、对象或数组。
- **键**:对象中的名称,也使用双引号表示。
例如,一个典型的JSON结构可能如下所示:
```json
{
"name": "John Doe",
"age": 30,
"isEmployed": true,
"skills": ["Python", "JavaScript"],
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}
```
### 2.1.2 JSON与Python数据类型对应关系
JSON数据结构与Python中相应的数据类型有直接的对应关系。当使用Python处理JSON数据时,以下类型将被映射:
- JSON对象对应Python的**字典(dict)**。
- JSON数组对应Python的**列表(list)**。
- JSON字符串对应Python的**字符串(str)**。
- JSON数值对应Python的**数字(int或float)**。
- JSON布尔值对应Python的**布尔(bool)**。
- JSON的null值对应Python的**None**。
这种对应关系让在Python中处理JSON数据变得十分直接,利用Python内置的`json`模块,可以轻松地进行序列化和反序列化操作。
## 2.2 Python的json模块使用
### 2.2.1 json模块的序列化与反序列化
Python的`json`模块提供了两个主要的功能:将Python对象编码成JSON字符串(序列化),以及将JSON字符串解码成Python对象(反序列化)。以下是具体的代码示例及其逻辑分析:
```python
import json
# 将Python字典序列化为JSON字符串
data = {
'name': 'John Doe',
'age': 30,
'isEmployed': True,
'skills': ['Python', 'JavaScript'],
'address': {
'street': '123 Main St',
'city': 'Anytown'
}
}
json_str = json.dumps(data)
print(json_str)
```
在上面的代码段中,`json.dumps()`函数将Python字典转换成了JSON格式的字符串。输出的`json_str`将是一个字符串,包含了以JSON格式编码的数据。
要将JSON字符串转换回Python对象,可以使用`json.loads()`函数:
```python
# 将JSON字符串反序列化为Python字典
data_back = json.loads(json_str)
print(data_back)
```
这段代码展示了如何将JSON字符串解码为Python字典。`json.loads()`函数读取JSON格式的字符串,并返回对应的Python字典。
### 2.2.2 处理JSON编码与解码错误
在处理JSON数据时,可能会遇到编码错误(比如Python中的非UTF-8字符串)或解码错误(比如不规则的JSON格式)。Python的`json`模块允许捕获和处理这些错误:
```python
import json
# 假设有一个包含非ASCII字符的字符串
data = {"greeting": "你好"}
# 尝试将包含非ASCII字符的字典序列化为JSON字符串
try:
json_str = json.dumps(data)
print(json_str)
except UnicodeEncodeError as e:
print(f"编码错误: {e}")
# 尝试将格式不正确的JSON字符串反序列化为Python字典
broken_json = "{\"name\":\"John Doe\" \"age\":30}"
try:
data_back = json.loads(broken_json)
print(data_back)
except json.JSONDecodeError as e:
print(f"解码错误: {e}")
```
以上示例代码展示了如何处理可能发生的编码和解码错误。错误被正确捕获,并打印了异常信息,而不是让程序崩溃。
### 2.2.3 自定义JSON解码器和编码器
当标准的JSON序列化和反序列化不足以满足需求时,`json`模块允许用户定义自定义的解码器和编码器。这在处理特殊的数据类型或进行数据转换时尤其有用。
```python
import json
import decimal
# 自定义编码器,用于处理Decimal类型
class DecimalEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, decimal.Decimal):
return str(obj)
return json.JSONEncoder.default(self, obj)
# 使用自定义的编码器
data = {'price': decimal.Decimal('10.99')}
json_str = json.dumps(data, cls=DecimalEncoder)
print(json_str)
# 自定义解码器
def custom_decoder(dct):
for key, value in dct.items():
if isinstance(value, str):
try:
dct[key] = decimal.Decimal(value)
except decimal.InvalidOperation:
pass
return dct
# 使用自定义的解码器
data_back = json.loads(json_str, object_hook=custom_decoder)
print(data_back)
```
在上面的代码中,定义了一个自定义的`DecimalEncoder`类,它覆盖了`JSONEncoder`类的`default`方法,以确保`decimal.Decimal`类型的实例能够被正确地转换为字符串。同时,定义了一个`custom_decoder`函数作为解码钩子,以将JSON字符串正确地转换回`Decimal`类型。
## 2.3 高级JSON处理技术
### 2.3.1 处理大型JSON文件
处理大型JSON文件时,一次性加载整个文件到内存可能导致内存耗尽。Python的`json`模块支持流式处理,通过`json.JSONDecoder`类可以实现逐步解析,从而节省内存。
```python
import json
# 打开JSON文件
with open('large_json_file.json', 'r', encoding='utf-8') as f:
decoder = json.JSONDecoder()
chunk = f.read(1024) # 读取1KB的数据
while chunk:
# 部分解析JSON数据
obj, index = decoder.raw_decode(chunk)
# 进行处理
print(obj)
chunk = f.read(1024) # 继续读取下一部分数据
```
在上述代码中,通过逐块读取大型JSON文件,使用`JSONDecoder`的`raw_decode`方法,我们可以逐步解析JSON数据,而不是一次性加载整个文件到内存中。
### 2.3.2 流式解析JSON数据
流式解析JSON数据涉及到对JSON数据流的处理,这在处理网络请求或实时数据流时尤其有用。Python的`ijson`库是专门用于流式处理JSON数据的第三方库,它可以逐个元素地迭代大型JSON文件。
```python
import ijson
# 使用ijson逐个元素地处理大型JSON文件
with open('large_json_file.json', 'rb') as f:
parser = ijson.items(f, 'item')
for item in parser:
# 处理每个元素
print(item)
```
在这个示例中,`ijson.items()`函数用于迭代文件中的每个元素。它允许我们逐步处理大型JSON文件,而不需要一次性将整个文件加载到内存中。
### 表格:Python处理JSON的方法与场景对比
| 方法 | 优点 | 场景 |
| --- | --- | --- |
| `json.dumps()` | 简单易用,适用于数据量不大的情况 | 小型数据的序列化 |
| `json.loads()` | 简单易用,适用于数据量不大的情况 | 小型数据的反序列化 |
| `json.JSONDecoder` | 逐块解析,节省内存 | 大型JSON文件处理 |
| `ijson` | 逐个元素迭代,高效处理大型文件 | 实时数据流或大型文件的高效处理 |
在选择处理JSON数据的方法时,需要根据数据的大小和处理的效率来决定使用哪种方式。
通过本章节的介绍,我们深入探讨了Python中JSON库的基本使用方法,并通过具体的代码和逻辑分析演示了如何有效地处理JSON数据。下一章将深入探讨复杂JSON结构的处理策略和基于Python的高级JSON操作。
# 3. 复杂JSON结构处理实践
## 3.1 复杂JSON结构解析策略
### 3.1.1 处理嵌套JSON对象
嵌套的JSON对象是在实际应用中常见的结构,它们往往包含多层键值对,其中值可以是数据类型,也可以是其他JSON对象或数组。正确处理嵌套JSON对象,是确保数据准确性和提高数据处理效率的关键。下面给出一个处理嵌套JSON对象的Python示例。
```python
import json
# 示例JSON字符串,包含嵌套的JSON对象
json_str = '''
{
"company": "TechCorp",
"employees": [
{
"name": "John Doe",
"department": "Development",
"projects": ["Project Alpha", "Project Beta"]
},
{
"name": "Jane Smith",
"department": "Marketing",
"projects": ["Campaign Gamma"]
}
```
0
0
相关推荐
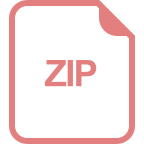
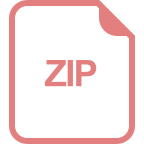
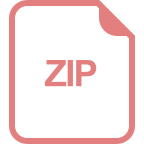
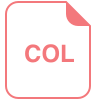
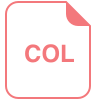
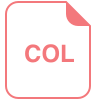
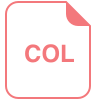

