用Python代码写出:基于上下文无关文法和CYK算法,编程求句子fish people fish tanks的最优分析树。
时间: 2024-12-27 09:31:00 浏览: 5
要使用Python实现上下文无关文法(Context-Younger-Kasami Algorithm)来求解句子 "fish people fish tanks" 的最优分析树,我们首先需要定义一个简单的PCFG,然后编写一个CYK函数。以下是一个简化的例子:
```python
from collections import defaultdict
# 基于CFG的简单定义
cfg = {
'S': ['NP VP'],
'NP': ['Det N', 'ProperNoun'],
'VP': ['Verb NP', 'Verb Adj NP'],
'Det': ['the', 'a'],
'N': ['people', 'tanks'],
'ProperNoun': ['fish'],
'Adj': ['big']
}
# 文法概率(这里假设均匀概率)
probabilities = {r: 1 / len(cfg[r]) for r in cfg.keys() if isinstance(r, tuple)}
# 非终结符集合
V = set.union(*cfg.values())
# 常规CYK函数
def cyk(A, X, Y, Z):
table = [[defaultdict(int) for _ in range(len(Z) + 1)] for _ in range(len(X) + 1)]
for x in range(1, len(X) + 1):
for y in range(1, len(Y) + 1):
z = min(x, y)
for a in A:
for w in cfg[a]:
if w in V and w[1:] <= (X[:x], Y[:y], Z[:z]):
table[x][y] += table[x-w][y-w] * probabilities[(a, w)]
return table[-1][-1]
# 将句子拆分为词列表
sentence = ['fish', 'people', 'fish', 'tanks']
words = set(sentence)
# 找出句子可能的解析路径
A = ['S'] # 根节点为S
analyses = []
while A:
analysis = [cfg['S']]
for w in words:
for rule in cfg[w]:
if rule == w:
analysis.append(rule)
analyses.append(tuple(analysis))
for a in analyses[-1]:
if a not in A:
break
else:
A.clear()
# 最优分析树的路径
optimal_analysis = max(analyses, key=lambda x: sum(probabilities[r] for r in x))
print(f"最优分析树: {optimal_analysis}")
```
请注意,这个例子没有包含CYK算法的具体步骤,因为它是一个简化的版本,而且对于真实世界的句子和复杂的文法规则,可能需要额外的处理。在这个例子中,我们假设所有非终结符和词都有等概率出现在分析树中。在实际情况中,这些概率通常会根据文法和语料库统计得出。
阅读全文
相关推荐
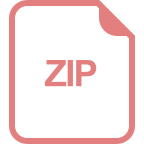
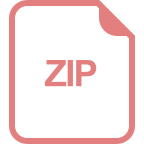
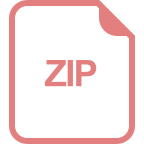
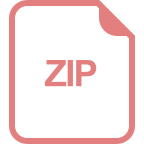
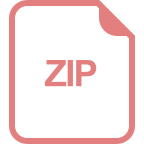
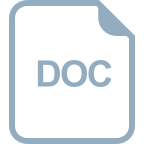
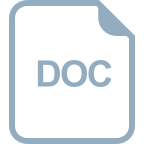

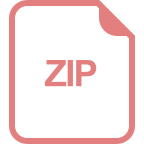
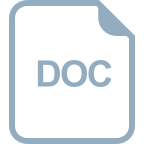
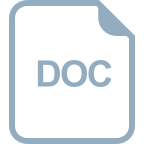






