用python编写五子棋游戏代码
时间: 2023-08-31 18:35:24 浏览: 150
### 回答1:
好的,我可以帮助你写五子棋游戏代码。首先,你需要准备一个二维列表来存储棋盘信息,并为每个玩家准备一个变量来存储他们的棋子颜色。然后,你可以使用一个循环来让玩家交替落子。在每次落子之后,你需要检查该玩家是否获胜,方法是检查他的棋子是否有五颗连在一起。如果有,则该玩家获胜。否则,游戏继续直到棋盘已满或有一方获胜。
下面是一个简单的示例代码,你可以根据自己的需要进行修改:
```
# 定义棋盘,用0表示没有棋子
board = [[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]]
# 定义玩家的棋子颜色
black = 1
white = 2
# 定义当前落子的玩家
current_player = black
# 定义游戏是否结束的标志
game_over = False
# 开始游戏循
### 回答2:
五子棋是一种非常经典的棋类游戏,玩家通过在棋盘上放置黑白两种颜色的棋子,以先在横、竖、斜线上连成五个相同颜色的棋子为目标。下面是使用Python编写的一个简单版本的五子棋游戏代码:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋盘
board = np.zeros((BOARD_SIZE, BOARD_SIZE))
# 定义当前落子的颜色,初始为黑子
current_color = 1
# 定义游戏主循环
while True:
# 打印当前棋盘
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i, j] == 1:
print("●", end=" ")
elif board[i, j] == -1:
print("○", end=" ")
else:
print("┼", end=" ")
print()
# 获取下棋位置
x = input("请输入横坐标:")
y = input("请输入纵坐标:")
# 将输入的位置转换为整数
x = int(x)
y = int(y)
# 判断输入是否合法
if x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE or board[x, y] != 0:
print("输入不合法,请重新输入!")
continue
# 在棋盘上落子
board[x, y] = current_color
# 判断游戏是否结束
# 判断横向是否有五子连线
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE):
if board[i, j] == current_color and board[i+1, j] == current_color \
and board[i+2, j] == current_color and board[i+3, j] == current_color \
and board[i+4, j] == current_color:
print("游戏结束,{}方获胜!".format("黑" if current_color == 1 else "白"))
exit(0)
# 判断纵向是否有五子连线
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if board[i, j] == current_color and board[i, j+1] == current_color \
and board[i, j+2] == current_color and board[i, j+3] == current_color \
and board[i, j+4] == current_color:
print("游戏结束,{}方获胜!".format("黑" if current_color == 1 else "白"))
exit(0)
# 判断主对角线是否有五子连线
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE - 4):
if board[i, j] == current_color and board[i+1, j+1] == current_color \
and board[i+2, j+2] == current_color and board[i+3, j+3] == current_color \
and board[i+4, j+4] == current_color:
print("游戏结束,{}方获胜!".format("黑" if current_color == 1 else "白"))
exit(0)
# 判断副对角线是否有五子连线
for i in range(BOARD_SIZE - 4):
for j in range(4, BOARD_SIZE):
if board[i, j] == current_color and board[i+1, j-1] == current_color \
and board[i+2, j-2] == current_color and board[i+3, j-3] == current_color \
and board[i+4, j-4] == current_color:
print("游戏结束,{}方获胜!".format("黑" if current_color == 1 else "白"))
exit(0)
# 切换落子颜色
current_color = -current_color
```
以上是使用Python编写的一个简单版本的五子棋游戏代码。通过键盘输入横、纵坐标进行落子,并判断游戏是否结束。希望以上内容能满足您的需求。
### 回答3:
五子棋是一种古老而受欢迎的棋类游戏,可以用Python编写一个基本的五子棋游戏代码。下面是一个简单的示例:
```python
# 导入必要的库
import numpy as np
# 初始化棋盘
def create_board():
board = np.zeros((15, 15))
return board
# 检查是否下棋到有效位置
def is_valid_move(board, row, col):
return board[row][col] == 0
# 下棋
def make_move(board, row, col, player):
board[row][col] = player
# 检查是否有玩家获胜
def is_winner(board, player):
# 检查行和列
for i in range(15):
if (board[i] == [player]*5).all() or (board[:,i] == [player]*5).all():
return True
# 检查对角线
for i in range(11):
for j in range(11):
if (board[i:i+5, j:j+5].diagonal() == [player]*5).all() or (np.fliplr(board[i:i+5, j:j+5]).diagonal() == [player]*5).all():
return True
return False
# 主程序
def play_game():
board = create_board()
player = 1
while True:
print(board)
row = int(input("请输入行数:"))
col = int(input("请输入列数:"))
if is_valid_move(board, row, col): # 检查是否有效位置
make_move(board, row, col, player) # 下棋
if is_winner(board, player): # 检查是否获胜
print(f"玩家 {player} 获胜!")
break
if player == 1:
player = 2
else:
player = 1
else:
print("无效的位置,请重新输入!")
# 运行游戏
play_game()
```
此代码使用numpy库来创建15x15的棋盘,玩家1使用数字1表示,玩家2使用数字2表示。游戏开始后,逐轮交替进行棋子落子,玩家根据提示输入行号和列号来落子,并检查落子的有效性。每次落子后,检查是否有玩家获胜。如果有玩家获胜,则游戏结束。
阅读全文
相关推荐












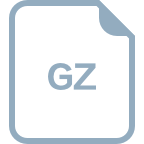