编写五子棋的完整python代码_python制作简单五子棋游戏
时间: 2023-08-25 21:36:45 浏览: 112
好的,以下是一个简单的五子棋游戏的Python代码:
```python
import numpy as np
# 初始化棋盘
def create_board():
board = np.zeros((15,15))
return board
# 判断落子是否合法
def is_valid_move(board, row, col):
if board[row][col] == 0:
return True
else:
return False
# 落子
def place_piece(board, row, col, piece):
board[row][col] = piece
# 判断是否有五子连珠
def check_win(board, piece):
# 横向
for r in range(15):
for c in range(11):
if board[r][c] == piece and board[r][c+1] == piece and board[r][c+2] == piece and board[r][c+3] == piece and board[r][c+4] == piece:
return True
# 纵向
for r in range(11):
for c in range(15):
if board[r][c] == piece and board[r+1][c] == piece and board[r+2][c] == piece and board[r+3][c] == piece and board[r+4][c] == piece:
return True
# 斜向
for r in range(11):
for c in range(11):
if board[r][c] == piece and board[r+1][c+1] == piece and board[r+2][c+2] == piece and board[r+3][c+3] == piece and board[r+4][c+4] == piece:
return True
for r in range(11):
for c in range(4, 15):
if board[r][c] == piece and board[r+1][c-1] == piece and board[r+2][c-2] == piece and board[r+3][c-3] == piece and board[r+4][c-4] == piece:
return True
return False
# 打印棋盘
def print_board(board):
print(np.flip(board, 0))
# 主程序
def play_game():
board = create_board()
game_over = False
turn = 1
while not game_over:
if turn == 1:
row = int(input("Player 1, please enter row (0-14): "))
col = int(input("Player 1, please enter column (0-14): "))
if is_valid_move(board, row, col):
place_piece(board, row, col, 1)
if check_win(board, 1):
print("Player 1 wins!")
game_over = True
turn = 2
else:
print("Invalid move! Please try again.")
else:
row = int(input("Player 2, please enter row (0-14): "))
col = int(input("Player 2, please enter column (0-14): "))
if is_valid_move(board, row, col):
place_piece(board, row, col, 2)
if check_win(board, 2):
print("Player 2 wins!")
game_over = True
turn = 1
else:
print("Invalid move! Please try again.")
print_board(board)
play_game()
```
这个程序使用了numpy库来创建和操作二维数组,实现了五子棋的基本功能。你可以在控制台中输入行和列的坐标来落子,程序会自动判断是否合法并检查是否有五子连珠。如果有玩家获胜,程序会输出相应的提示信息并结束游戏。
阅读全文
相关推荐
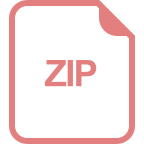
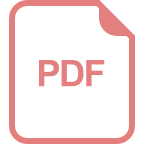
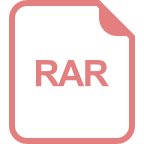

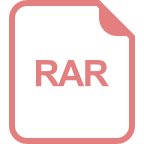
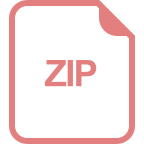
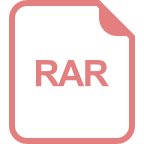
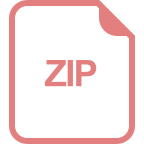
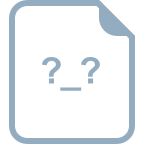
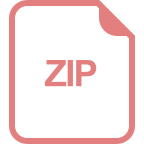