编写可实现人机对战和双人对战的五子棋的完整python代码
时间: 2023-10-11 14:05:54 浏览: 52
以下是一个简单的五子棋游戏的Python代码,支持人机对战和双人对战:
```python
import random
# 棋盘大小
BOARD_SIZE = 15
# 空白位置标记
EMPTY = 0
# 玩家和电脑标记
PLAYER = 1
COMPUTER = 2
# 初始化棋盘
def init_board():
board = []
for i in range(BOARD_SIZE):
row = [EMPTY] * BOARD_SIZE
board.append(row)
return board
# 打印棋盘
def print_board(board):
print(" ", end="")
for x in range(BOARD_SIZE):
print("{0:2d}".format(x+1), end="")
print()
for i in range(BOARD_SIZE):
print("{0:2d}".format(i+1), end="")
for j in range(BOARD_SIZE):
if board[i][j] == EMPTY:
print(" .", end="")
elif board[i][j] == PLAYER:
print(" O", end="")
elif board[i][j] == COMPUTER:
print(" X", end="")
print()
# 判断胜负
def check_win(board, player):
# 判断横向是否有五子连珠
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if board[i][j] == player and board[i][j+1] == player and board[i][j+2] == player and board[i][j+3] == player and board[i][j+4] == player:
return True
# 判断纵向是否有五子连珠
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE):
if board[i][j] == player and board[i+1][j] == player and board[i+2][j] == player and board[i+3][j] == player and board[i+4][j] == player:
return True
# 判断斜向是否有五子连珠
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE - 4):
if board[i][j] == player and board[i+1][j+1] == player and board[i+2][j+2] == player and board[i+3][j+3] == player and board[i+4][j+4] == player:
return True
# 判断反斜向是否有五子连珠
for i in range(4, BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if board[i][j] == player and board[i-1][j+1] == player and board[i-2][j+2] == player and board[i-3][j+3] == player and board[i-4][j+4] == player:
return True
return False
# 人类玩家下棋
def player_move(board):
while True:
row = int(input("请输入行号(1~15):"))
col = int(input("请输入列号(1~15):"))
if row < 1 or row > BOARD_SIZE or col < 1 or col > BOARD_SIZE:
print("输入错误,请重新输入!")
elif board[row-1][col-1] != EMPTY:
print("该位置已经有棋子,请重新输入!")
else:
board[row-1][col-1] = PLAYER
break
# 计算每个位置的分数
def calculate_score(board, player):
# 分数表
score_table = [
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 20, 30, 35, 40, 40, 35, 30, 20, 0, 0, 0, 0, 0, 0],
[0, 30, 40, 45, 50, 50, 45, 40, 30, 0, 0, 0, 0, 0, 0],
[0, 35, 45, 50, 55, 55, 50, 45, 35, 0, 0, 0, 0, 0, 0],
[0, 40, 50, 55, 60, 60, 55, 50, 40, 0, 0, 0, 0, 0, 0],
[0, 40, 50, 55, 60, 60, 55, 50, 40, 0, 0, 0, 0, 0, 0],
[0, 35, 45, 50, 55, 55, 50, 45, 35, 0, 0, 0, 0, 0, 0],
[0, 30, 40, 45, 50, 50, 45, 40, 30, 0, 0, 0, 0, 0, 0],
[0, 20, 30, 35, 40, 40, 35, 30, 20, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
]
# 计算每个位置的得分
scores = []
for i in range(BOARD_SIZE):
row = []
for j in range(BOARD_SIZE):
if board[i][j] == EMPTY:
score = score_table[i][j]
# 横向
if j > 0 and j < BOARD_SIZE-4:
if board[i][j-1] == player and board[i][j+1] == player:
score += 30
elif board[i][j-1] == player or board[i][j+1] == player:
score += 15
# 纵向
if i > 0 and i < BOARD_SIZE-4:
if board[i-1][j] == player and board[i+1][j] == player:
score += 30
elif board[i-1][j] == player or board[i+1][j] == player:
score += 15
# 斜向
if i > 0 and i < BOARD_SIZE-4 and j > 0 and j < BOARD_SIZE-4:
if board[i-1][j-1] == player and board[i+1][j+1] == player:
score += 30
elif board[i-1][j-1] == player or board[i+1][j+1] == player:
score += 15
# 反斜向
if i > 0 and i < BOARD_SIZE-4 and j > 3 and j < BOARD_SIZE:
if board[i-1][j+1] == player and board[i+1][j-1] == player:
score += 30
elif board[i-1][j+1] == player or board[i+1][j-1] == player:
score += 15
row.append(score)
else:
row.append(0)
scores.append(row)
return scores
# 电脑玩家下棋
def computer_move(board):
# 计算每个位置的得分
player_scores = calculate_score(board, PLAYER)
computer_scores = calculate_score(board, COMPUTER)
# 找到最高分数的位置
max_score = 0
candidates = []
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == EMPTY:
score = player_scores[i][j] + computer_scores[i][j]
if score > max_score:
max_score = score
candidates = [(i, j)]
elif score == max_score:
candidates.append((i, j))
# 在候选位置中随机选择一个
row, col = random.choice(candidates)
board[row][col] = COMPUTER
# 游戏主循环
def main():
board = init_board()
print_board(board)
while True:
# 人类玩家下棋
player_move(board)
print_board(board)
if check_win(board, PLAYER):
print("恭喜你获胜!")
break
# 电脑玩家下棋
computer_move(board)
print_board(board)
if check_win(board, COMPUTER):
print("很遗憾,你输了!")
break
if __name__ == '__main__':
main()
```
运行程序后,按照提示输入行号和列号即可下棋。如果选择人机对战,电脑会自动计算每个位置的得分,并选择得分最高的位置下棋。如果选择双人对战,两个玩家轮流下棋,直到有一方获胜。
相关推荐
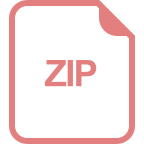












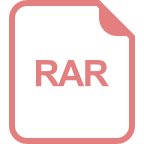