用python输入年月日,输出第二天的年月日,考虑闰年闰月
时间: 2023-04-04 14:03:36 浏览: 109
您好,我可以回答这个问题。以下是代码示例:
```python
import datetime
# 输入年月日
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 转换为日期格式
date = datetime.date(year, month, day)
# 计算下一天的日期
next_day = date + datetime.timedelta(days=1)
# 输出结果
print("下一天的日期为:{}年{}月{}日".format(next_day.year, next_day.month, next_day.day))
```
这个代码可以考虑闰年和闰月的情况,因为它使用了 Python 内置的 `datetime` 模块来处理日期和时间。
相关问题
python输入年月日输出下一天
以下是Python程序,用于输入年月日并输出下一天的日期:
```python
# 获取用户输入的年、月、日
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 计算下一天的日期
if month in [1, 3, 5, 7, 8, 10, 12]:
if day == 31:
next_day = 1
next_month = month + 1
else:
next_day = day + 1
next_month = month
elif month in [4, 6, 9, 11]:
if day == 30:
next_day = 1
next_month = month + 1
else:
next_day = day + 1
next_month = month
else:
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
if day == 29:
next_day = 1
next_month = month + 1
else:
next_day = day + 1
next_month = month
else:
if day == 28:
next_day = 1
next_month = month + 1
else:
next_day = day + 1
next_month = month
if next_month == 13:
next_month = 1
next_year = year + 1
else:
next_year = year
# 输出下一天的日期
print("下一天的日期为:%d年%d月%d日" % (next_year, next_month, next_day))
```
这个程序首先获取用户输入的年、月、日,然后根据月份和日期计算下一天的日期。如果当前是月底,那么下一天就是下一个月的第一天;否则,下一天就是当月的下一天。对于闰年的情况,需要特别处理2月份的情况。最后,程序输出下一天的日期。
python输入年月日判断是这一年的第几天
### 回答1:
可以使用Python编写一个函数来实现输入年月日判断是这一年的第几天的功能。具体实现方法如下:
```python
def day_of_year(year, month, day):
"""
输入年月日判断是这一年的第几天
:param year: 年份
:param month: 月份
:param day: 日
:return: 这一年的第几天
"""
days_of_month = [, 31, 59, 90, 120, 151, 181, 212, 243, 273, 304, 334] # 每个月的天数
days = days_of_month[month - 1] + day # 当前月份之前的天数加上当前天数
if month > 2 and year % 4 == and (year % 100 != or year % 400 == ):
days += 1 # 如果是闰年且当前月份大于2月,则加上一天
return days
```
这个函数的实现思路是,先定义一个列表`days_of_month`,其中存储了每个月的天数。然后根据输入的年月日,计算出当前月份之前的天数加上当前天数,得到这一年的第几天。如果当前年份是闰年且当前月份大于2月,则需要再加上一天。
例如,输入年月日为2021年3月1日,则调用`day_of_year(2021, 3, 1)`函数,返回的结果为60,表示2021年的第60天。
### 回答2:
Python是一种面向对象、解释型高级编程语言,通常情况下Python语言的应用可以在数学、科学、Web应用程序、自然语言处理以及其他各种域中发挥作用。Python语言也支持日常生活中的各种常见操作。本文介绍一种通过Python输入年月日判断是这一年的第几天的方法。
要实现这个任务,我们可以使用Python中的datetime库中的datetime类。首先需要导入datetime类和date类:
```python
from datetime import datetime, date
```
然后我们可以将输入的年、月、日转换成datetime对象,如下所示:
```python
def day_of_year(year, month, day):
date1 = datetime(year=year, month=1, day=1)
date2 = datetime(year=year, month=month, day=day)
return (date2 - date1).days + 1
```
这里的datetime(year,month,day)函数可以将输入的年、月、日转换成一个datetime对象。然后我们将计算出这一天是这一年的第几天。这里用到了datetime库中的days()函数来计算这两个日期之间的天数。最后,从1加上计算出的天数就是这一年的第几天了。
最后,我们可以在Python中进行调用,如下所示:
```python
print(day_of_year(2021, 3, 13))
```
运行结果为:
```python
72
```
这说明了3月13日是2021年的第72天。
总之,Python作为一种高级编程语言,可以快速、高效、准确地实现各种功能。输入年月日判断是这一年的第几天这个问题可以通过datetime库中的datetime类轻松实现。
### 回答3:
Python是一种高级编程语言,具有强大的文本处理和数值计算工具,被广泛地应用于数据分析,数据可视化,科学计算等领域。在Python中,我们可以利用日历模块和datetime模块来计算输入日期是这一年的第几天。
首先,我们用input()函数让用户输入年份,月份和日期:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
```
接下来,我们可以利用datetime模块,将输入的年份、月份、日期转换成datetime对象:
```python
import datetime
date = datetime.datetime(year, month, day)
```
然后,我们可以使用timedelta()函数计算这一年的第一天与输入日期之间的时间差,再将时间差转换成天数,即可得到该日期是这一年的第几天:
```python
first_day = datetime.datetime(year, 1, 1)
delta = date - first_day
days = delta.days + 1
```
最后,我们输出计算结果:
```python
print("{0}年{1}月{2}日是这一年的第{3}天".format(year, month, day, days))
```
完整代码如下:
```python
import datetime
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
date = datetime.datetime(year, month, day)
first_day = datetime.datetime(year, 1, 1)
delta = date - first_day
days = delta.days + 1
print("{0}年{1}月{2}日是这一年的第{3}天".format(year, month, day, days))
```
Python运行代码后,会提示用户输入年份、月份、和日期,用户输入后,Python会自动计算出该日期是这一年的第几天,并输出计算结果。
相关推荐
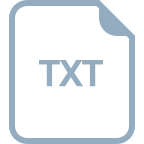












