c++输入年月日,输出该年有多少天
时间: 2023-09-07 08:04:19 浏览: 150
### 回答1:
您好,根据输入的年月日,可以计算出该年有多少天。具体的计算方法是,先判断该年是否为闰年,如果是闰年,则该年有366天,否则为365天。然后根据输入的月份,计算出该月有多少天,最后将该月的天数加上前面所有月份的天数,即可得到该年的总天数。
### 回答2:
输入一个日期,可以通过判断是闰年还是平年来确定该年有多少天。
闰年的判断规则是:年份能被4整除但不能被100整除,或者能被400整除的年份都是闰年。
首先,我们将输入的年份、月份、日期存储到变量中,然后进行判断。
判断该年份是否为闰年,如果是闰年,将该年份的天数设置为366天;如果不是闰年,则天数为365天。
然后,我们根据输入的月份判断每个月份的天数。一般来说,1月、3月、5月、7月、8月、10月、12月都是31天;4月、6月、9月、11月都是30天;2月则需要判断是否是闰年。
如果是2月且是闰年,将天数设置为29天;如果是2月且不是闰年,将天数设置为28天。
最后输出计算得出的天数即可。
代码示例:
```
def calculate_days(year, month, day):
days = 0
# 判断年份是否为闰年
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
days = 366
else:
days = 365
# 判断月份的天数
if month in [1, 3, 5, 7, 8, 10, 12]:
days += 31
elif month in [4, 6, 9, 11]:
days += 30
elif month == 2:
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
days += 29
else:
days += 28
return days
# 输入年月日
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 计算天数
total_days = calculate_days(year, month, day)
# 输出结果
print("该年有", total_days, "天。")
```
运行示例:
请输入年份:2022
请输入月份:3
请输入日期:15
该年有 365 天。
### 回答3:
要输出某年有多少天,需要考虑以下几个方面:
1. 首先判断该年是否为闰年。闰年有366天,非闰年为365天。通过以下规则来判断是否为闰年:
- 若年份能被4整除但不能被100整除,则为闰年。
- 若年份能被400整除,则也是闰年。
2. 对于非闰年,每个月的天数如下:
- 1月:31天
- 2月:28天
- 3月:31天
- 4月:30天
- 5月:31天
- 6月:30天
- 7月:31天
- 8月:31天
- 9月:30天
- 10月:31天
- 11月:30天
- 12月:31天
3. 对于闰年,2月的天数为29天,其他月份与非闰年相同。
因此,可以根据输入的年份和月份来判断该年有多少天。
以下是一个用Python语言实现的例子:
```python
def get_days_in_year(year, month, day):
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0: # 判断闰年
days_in_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
else:
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
return sum(days_in_month[:month - 1]) + day # 累加前几个月的天数,再加上当前月的天数
# 输入年月日
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
days = get_days_in_year(year, month, day) # 调用函数计算天数
print(f"{year}年有{days}天。")
```
注意:由于输入的年份、月份和日期的合法性没有进行特别的判断,在实际应用中可能需要添加一些错误处理的逻辑。
阅读全文
相关推荐
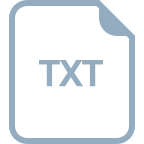
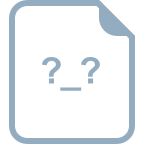
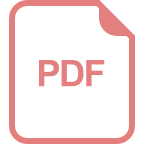
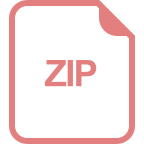
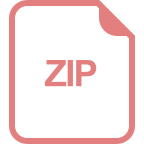
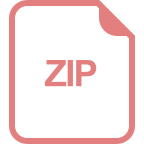
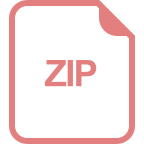
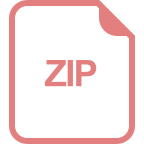