c语言小游戏源代码贪吃蛇
时间: 2024-12-28 21:26:34 浏览: 14
### C语言实现的贪吃蛇游戏完整源码示例
以下是基于提供的参考资料整理的一个完整的C语言版本的贪吃蛇游戏源代码:
```c
#include <stdio.h>
#include <conio.h> // For _kbhit() and getch()
#include <windows.h> // For Sleep()
#define WIDTH 20
#define HEIGHT 20
#define SNAKE_SIZE 10
#define SPEED 100 // Speed of the game, lower is faster
int gameOver;
char board[HEIGHT][WIDTH];
int snakeX[SNAKE_SIZE], snakeY[SNAKE_SIZE]; // Snake coordinates array
int fruitX, fruitY;
int nTail;
enum eDirection { STOP = 0, LEFT, RIGHT, UP, DOWN };
enum eDirection dir;
void Setup() {
int i;
gameOver = 0;
dir = STOP;
nTail = 3; // Initial length of snake
// Initialize snake position at center
for (i = 0; i < nTail; ++i) {
snakeX[i] = WIDTH / 2 - i;
snakeY[i] = HEIGHT / 2;
}
// Place first piece of food randomly on grid
srand(time(0));
fruitX = rand() % WIDTH;
fruitY = rand() % HEIGHT;
}
void Draw() {
system("cls"); // Clear screen before drawing new frame
for (int row = 0; row < HEIGHT; ++row) {
for (int col = 0; col < WIDTH; ++col) {
if (row == 0 || row == HEIGHT - 1 || col == 0 || col == WIDTH - 1)
printf("#");
else if (row == snakeY[0] && col == snakeX[0])
printf("O"); // Head of snake
else {
int printed = 0;
for (int z = 1; z < nTail; z++) {
if (snakeX[z] == col && snakeY[z] == row) {
printf("o"); // Body part of snake
printed = 1;
}
}
if (!printed) {
if (fruitY == row && fruitX == col)
printf("*"); // Food item
else
printf(" ");
}
}
}
printf("\n");
}
for (int i = 0; i < WIDTH + 2; ++i)
printf("#");
printf("\nScore: %d\n", nTail);
}
void Input() {
if (_kbhit()) {
switch(getch())
{
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x': // Exit key
gameOver = 1;
break;
}
}
}
void Logic() {
int prevX = snakeX[0];
int prevY = snakeY[0];
int prev2X, prev2Y;
snakeX[0] = snakeX[0] + ((dir == RIGHT ? 1 : 0) - (dir == LEFT ? 1 : 0));
snakeY[0] = snakeY[0] + ((dir == DOWN ? 1 : 0) - (dir == UP ? 1 : 0));
// Check collision with walls or self
if(snakeX[0] >= WIDTH || snakeX[0] < 0 ||
snakeY[0] >= HEIGHT || snakeY[0] < 0 ||
IsCollisionWithSelf(prevX, prevY))
gameOver = 1;
// Move body parts one by one following head movement
for(int i=1;i<nTail;i++){
prev2X = snakeX[i];
prev2Y = snakeY[i];
snakeX[i]=prevX;
snakeY[i]=prevY;
prevX = prev2X;
prevY = prev2Y;
}
// Grow when eating a fruit
if(snakeX[0]==fruitX&&snakeY[0]==fruitY){
nTail++;
fruitX=rand()%WIDTH;
fruitY=rand()%HEIGHT;
}
}
// Helper function to check collisions between snake's segments
int IsCollisionWithSelf(int x, int y) {
for(int i=1;i<nTail;i++)
if(x==snakeX[i]&&y==snakeY[i]) return 1;
return 0;
}
int main(){
Setup();
while(!gameOver){
Draw();
Input();
Logic();
Sleep(SPEED); // Control speed using Sleep from windows.h library[^3]
}
}
```
这段代码实现了基本功能,包括初始化设置、绘制界面、处理输入以及逻辑更新。通过`Sleep()`函数可以调节游戏的速度。
阅读全文
相关推荐
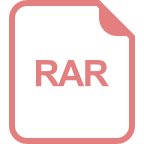
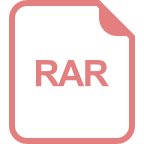
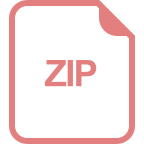
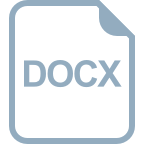
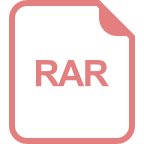
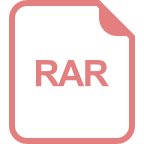
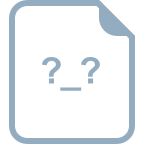
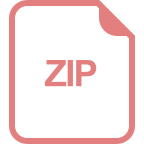
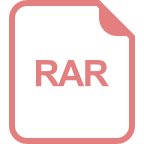
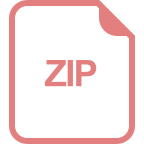
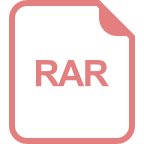
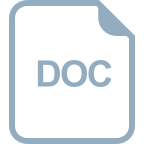
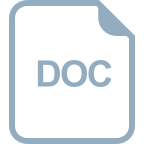
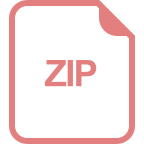

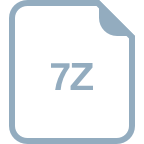