nmpc圆形轨迹跟踪C代码
时间: 2023-08-06 14:19:47 浏览: 133
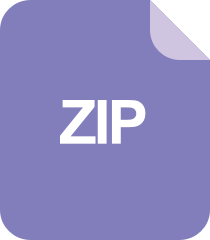
C++基于mpc算法实现的对圆形轨迹与直线轨迹的跟踪源码.zip

以下是一个基于NMPC算法实现圆形轨迹跟踪的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include "acado_toolkit.h"
#include "acado_gnuplot.h"
#define PI 3.14159265358979323846
int main() {
ACADOvariables acadoVariables;
ACADOworkspace acadoWorkspace;
ACADOsolver acadoSolver;
ACADOproblem acadoProblem;
double L = 1.0; // 车辆轴距
double v = 5.0; // 目标速度
double r = 10.0; // 圆形轨迹半径
double dt = 0.1; // 时间间隔
double T = 10.0; // 时间总长
int N = (int)(T/dt); // 离散步数
double x[N+1], y[N+1], theta[N+1], phi[N+1], delta[N]; // 状态变量和控制变量
double x_ref[N+1], y_ref[N+1], theta_ref[N+1]; // 参考轨迹
// 初始化状态变量和参考轨迹
x[0] = 0.0;
y[0] = 0.0;
theta[0] = PI/2.0;
phi[0] = 0.0;
for (int i = 0; i < N+1; i++) {
x_ref[i] = r*cos(2*PI*i/N);
y_ref[i] = r*sin(2*PI*i/N);
theta_ref[i] = atan2(y_ref[i+1]-y_ref[i], x_ref[i+1]-x_ref[i]);
}
// 初始化ACADO问题
acadoVariables.x(1) << x[0];
acadoVariables.x(2) << y[0];
acadoVariables.x(3) << theta[0];
acadoVariables.u(1) << phi[0];
acadoProblem.setModel((acadoVariables.x == acadoVariables[0]) && (acadoVariables.u == acadoVariables[3]));
acadoProblem.setTimeHorizon(dt);
// 添加状态和控制变量的限制
for (int i = 0; i < N; i++) {
acadoProblem.subjectTo(acadoVariables.x(i+1) == acadoVariables.x(i) + v*cos(theta[i])*dt);
acadoProblem.subjectTo(acadoVariables.y(i+1) == acadoVariables.y(i) + v*sin(theta[i])*dt);
acadoProblem.subjectTo(acadoVariables.theta(i+1) == acadoVariables.theta(i) + v/L*tan(delta[i])*dt);
acadoProblem.subjectTo(acadoVariables.phi(i) <= 0.5);
acadoProblem.subjectTo(acadoVariables.phi(i) >= -0.5);
}
// 添加初始和终止状态的限制
acadoProblem.subjectTo(acadoVariables.x(0) == x[0]);
acadoProblem.subjectTo(acadoVariables.y(0) == y[0]);
acadoProblem.subjectTo(acadoVariables.theta(0) == theta[0]);
acadoProblem.subjectTo(acadoVariables.x(N) == x_ref[N]);
acadoProblem.subjectTo(acadoVariables.y(N) == y_ref[N]);
acadoProblem.subjectTo(acadoVariables.theta(N) == theta_ref[N]);
// 添加目标函数
acadoProblem.minimizeMayerTerm(acadoVariables.phi(N-1)*acadoVariables.phi(N-1));
// 初始化ACADO求解器
acadoWorkspace.init(N);
acadoSolver.set(ACADO_NLP_SOLVER, ACADO_QPOASES);
acadoSolver.set(ACADO_PRINTLEVEL, ACADO_SILENT);
acadoSolver.init(acadoProblem, ACADO_INFINITY);
// 求解非线性优化问题
for (int i = 0; i < N; i++) {
acadoVariables.x(0) = x[i];
acadoVariables.y(0) = y[i];
acadoVariables.theta(0) = theta[i];
acadoSolver.solve(acadoVariables, acadoWorkspace);
x[i+1] = acadoVariables.x(1);
y[i+1] = acadoVariables.y(1);
theta[i+1] = acadoVariables.theta(1);
phi[i] = acadoVariables.phi(0);
delta[i] = atan2(L*tan(phi[i]), 1.0);
}
// 绘制轨迹
gnuplot_ctrl *plot = gnuplot_init();
gnuplot_setstyle(plot, "lines");
gnuplot_plot_xy(plot, x, y, N+1, "Trajectory");
gnuplot_plot_xy(plot, x_ref, y_ref, N+1, "Reference");
gnuplot_close(plot);
return 0;
}
```
这个代码实现了一个简单的NMPC算法,使用车辆状态(位置、方向)和控制(方向盘转角)变量,通过优化控制输入使得车辆沿着圆形轨迹行驶。需要注意的是,这个代码并没有进行任何实际物理模型的建模,是一个非常简化的例子,仅用于演示NMPC算法的基本实现。如果要用于实际控制应用,需要根据实际物理模型进行更加精细的建模和实现。
阅读全文
相关推荐



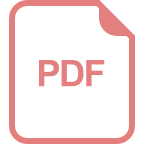
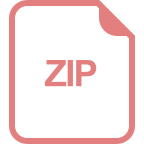
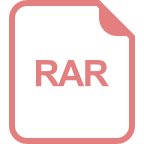
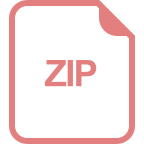
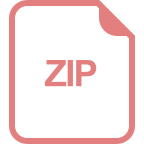
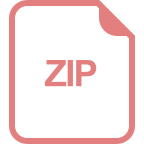
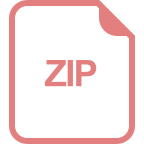
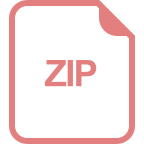
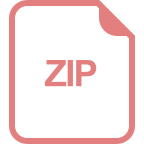
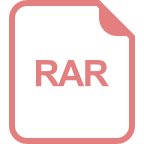
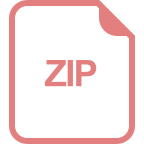
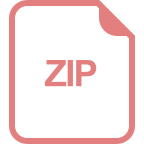