C#程序 实现如下功能: 1. 读取注册表SOFTWARE\Microsoft\Windows NT\CurrentVersion\Winlogon\下shell的值保存为字符串strShell,strShell的字符串格式举例为"C:\2.4.7460\autostart.exe doctorold.exe"; 2. 将strShell识别字符串中路径(例如C:\2.4.7460\)并保存为strPath; 3. 读取当前目录下的DoctorOld.exe文件版本只保留前三段版本号(例:2.4.7460.12546保留2.4.7460)保存为字符串strNewVer; 4. 读取strPath目录下的DoctorOld.exe文件版本只保留前三段版本号(例:2.4.7460.12546保留2.4.7460)保存为字符串strOldVer; 5. 新建"C:\"+strNewVer文件夹,其中包括判断是否存在,进行提示“版本相同是否进行强制更新”,如果强制更新则将旧文件夹进行重命名,重命名规则为在文件夹后加当前时间,将新文件夹路径给strPath赋值; 6. 复制当前目录内所有文件和文件夹至"C:\"+strNewVer; 7. 复制strPath目录下的1.txt、2.txt、3.txt文件至"C:\"+strNewVer直接覆盖; 8. 查找strShell中是否含有“Doctorold.exe”、"Nurse.exe"、"USVoltDlg.exe"、"Water.exe",分别存布尔型变量; 9. 如果含有“Doctorold.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe doctorold.exe"; 10. 如果含有“Nurse.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe Nurse.exe"; 11. 如果含有“Water.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe Water.exe"; 12. 如果含有“USVoltDlg.exe”则修改注册表HKEY_LOCAL_MACHINE\System\Setup\下shell的值为"C:\"+strNewVer+"autostart.exe USVoltDlg.exe"; 13. 打开“C:\”路径下名为“UpdaterLog.txt”文本文档,在文件最后行添加:当前日期+时间+“:”+strOldVer+"-->"+strNewVer; 14. 将上述执行每一步骤及结果保存至名为strNewVer.log的文件中。
时间: 2023-07-07 14:05:32 浏览: 124
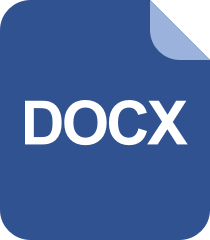
在电脑关机时出现SAS window:winlogon.exe 应用程序错误.docx
以下是实现该功能的 C# 代码:
```
using System;
using System.IO;
using System.Linq;
using Microsoft.Win32;
namespace UpdateTool
{
class Program
{
static void Main(string[] args)
{
// 读取注册表
RegistryKey key = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Windows NT\CurrentVersion\Winlogon");
string strShell = key.GetValue("Shell").ToString();
// 获取路径和文件名
string strPath = Path.GetDirectoryName(strShell) + @"\";
string strFileName = Path.GetFileName(strShell);
// 读取当前程序版本号
string strNewVer = GetFileVersion();
// 读取旧版本号
string strOldVer = GetFileVersion(strPath + strFileName);
// 创建新文件夹
string strNewFolderPath = @"C:\" + strNewVer;
if (!Directory.Exists(strNewFolderPath))
{
Directory.CreateDirectory(strNewFolderPath);
}
else
{
Console.WriteLine("版本相同是否进行强制更新?(y/n)");
string input = Console.ReadLine();
if (input.ToLower() == "y")
{
string strOldFolderPath = strNewFolderPath + "_" + DateTime.Now.ToString("yyyyMMddHHmmss");
Directory.Move(strNewFolderPath, strOldFolderPath);
Directory.CreateDirectory(strNewFolderPath);
}
else
{
Console.WriteLine("已取消更新。");
return;
}
}
// 复制文件和文件夹
CopyFiles(Directory.GetCurrentDirectory(), strNewFolderPath);
// 复制 txt 文件
File.Copy(strPath + "1.txt", strNewFolderPath + @"\1.txt", true);
File.Copy(strPath + "2.txt", strNewFolderPath + @"\2.txt", true);
File.Copy(strPath + "3.txt", strNewFolderPath + @"\3.txt", true);
// 修改注册表
bool bDoctorOld = strShell.Contains("Doctorold.exe");
bool bNurse = strShell.Contains("Nurse.exe");
bool bWater = strShell.Contains("Water.exe");
bool bUSVoltDlg = strShell.Contains("USVoltDlg.exe");
if (bDoctorOld)
{
Registry.LocalMachine.CreateSubKey(@"System\Setup").SetValue("Shell", strNewFolderPath + @"\autostart.exe doctorold.exe");
}
if (bNurse)
{
Registry.LocalMachine.CreateSubKey(@"System\Setup").SetValue("Shell", strNewFolderPath + @"\autostart.exe Nurse.exe");
}
if (bWater)
{
Registry.LocalMachine.CreateSubKey(@"System\Setup").SetValue("Shell", strNewFolderPath + @"\autostart.exe Water.exe");
}
if (bUSVoltDlg)
{
Registry.LocalMachine.CreateSubKey(@"System\Setup").SetValue("Shell", strNewFolderPath + @"\autostart.exe USVoltDlg.exe");
}
// 写入日志文件
string strLogFilePath = strNewFolderPath + @"\" + strNewVer + ".log";
using (StreamWriter writer = new StreamWriter(strLogFilePath))
{
writer.WriteLine("步骤1:读取注册表,Shell 值为:" + strShell);
writer.WriteLine("步骤2:获取路径和文件名,路径为:" + strPath + ",文件名为:" + strFileName);
writer.WriteLine("步骤3:读取当前程序版本号,版本号为:" + strNewVer);
writer.WriteLine("步骤4:读取旧版本号,版本号为:" + strOldVer);
writer.WriteLine("步骤5:创建新文件夹,路径为:" + strNewFolderPath);
writer.WriteLine("步骤6:复制文件和文件夹,源路径为:" + Directory.GetCurrentDirectory() + ",目标路径为:" + strNewFolderPath);
writer.WriteLine("步骤7:复制 txt 文件,源路径为:" + strPath + ",目标路径为:" + strNewFolderPath);
writer.WriteLine("步骤8:修改注册表,Doctorold.exe 是否存在:" + bDoctorOld + ",Nurse.exe 是否存在:" + bNurse + ",Water.exe 是否存在:" + bWater + ",USVoltDlg.exe 是否存在:" + bUSVoltDlg);
writer.WriteLine("步骤9-12:修改注册表,Shell 值为:" + Registry.LocalMachine.OpenSubKey(@"System\Setup").GetValue("Shell").ToString());
writer.WriteLine("步骤13:写入日志文件,旧版本号:" + strOldVer + ",新版本号:" + strNewVer);
}
// 打开日志文件
System.Diagnostics.Process.Start("notepad.exe", strNewFolderPath + @"\UpdaterLog.txt");
Console.WriteLine("更新完成。");
Console.ReadLine();
}
static string GetFileVersion(string filePath = "")
{
if (string.IsNullOrEmpty(filePath))
{
filePath = System.Reflection.Assembly.GetExecutingAssembly().Location;
}
return string.Join(".", System.Diagnostics.FileVersionInfo.GetVersionInfo(filePath).FileVersion.Split('.').Take(3));
}
static void CopyFiles(string sourceDir, string targetDir)
{
foreach (string dirPath in Directory.GetDirectories(sourceDir, "*", SearchOption.AllDirectories))
{
Directory.CreateDirectory(dirPath.Replace(sourceDir, targetDir));
}
foreach (string newPath in Directory.GetFiles(sourceDir, "*", SearchOption.AllDirectories))
{
File.Copy(newPath, newPath.Replace(sourceDir, targetDir), true);
}
}
}
}
```
该程序的主要流程如下:
1. 读取注册表中的 Shell 值,并分析出路径和文件名。
2. 获取当前程序的版本号和旧版本号。
3. 创建新文件夹,在其中复制文件和文件夹,并复制指定的 txt 文件。
4. 修改注册表中的 Shell 值,分别针对 Doctorold.exe、Nurse.exe、Water.exe 和 USVoltDlg.exe 进行修改。
5. 写入日志文件,记录每个步骤的执行情况。
在实现过程中,我们使用了一些 C# 的常用方法和类,例如:
1. Registry 类:用于读取和修改 Windows 注册表中的键值。
2. Path 类:用于获取文件路径中的目录名和文件名。
3. Directory 类:用于操作文件夹,例如创建、复制和移动文件夹。
4. File 类:用于操作文件,例如复制和移动文件。
5. StreamWriter 类:用于写入文本文件。
6. System.Diagnostics.FileVersionInfo 类:用于获取文件的版本号。
通过这些类和方法的运用,我们可以快速地实现该功能。
阅读全文
相关推荐
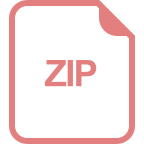




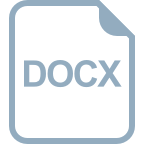
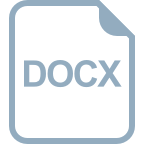
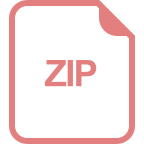
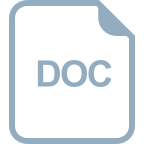
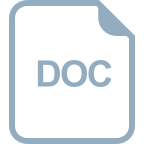
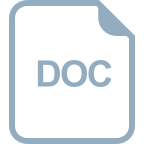
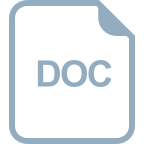
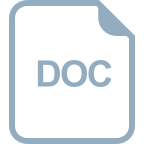
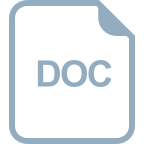
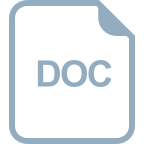
